Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial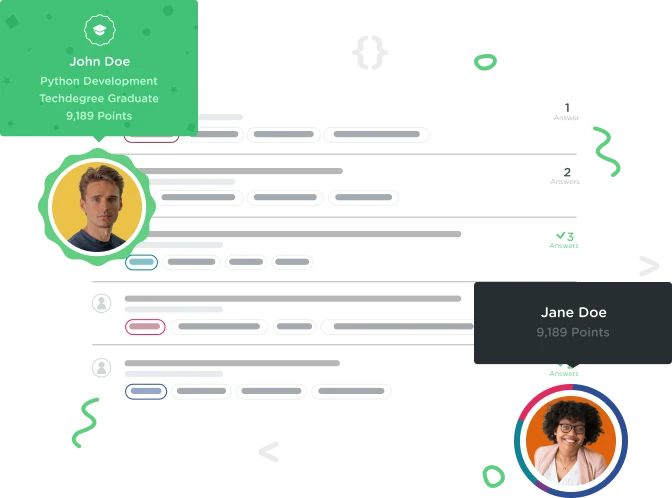
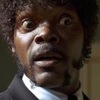
John Hammett
6,173 PointsCan someone please answer this??? It keeps saying I didn't add a new method to shopping cart!
Please Answer I need help!
public class Example {
public static void main(String[] args) {
ShoppingCart cart = new ShoppingCart();
Product pez = new Product("Cherry PEZ refill (12 pieces)");
cart.addItem(pez, 5);
/* Since a quantity of 1 is such a common argument when adding a product to the cart,
* your fellow developers have asked you to make the following code work, as well as keeping
* the ability to add a product and a quantity.
*/
Product dispenser = new Product("Yoda PEZ dispenser");
// Uncomment this line after using method signatures to solve their request in ShoppingCart.java
// cart.addItem(dispenser);
}
}
public class ShoppingCart {
public void addItem(Product item, int quantity) {
quantity = 1;
System.out.printf("Adding %d of %s to the cart.%n", quantity, item.getName());
/* Other code omitted for clarity */
}
}
public class Product {
/* Other code omitted for clarity, but you could imagine
it would store price, options like size and color
*/
private String mName;
public Product(String name) {
mName = name;
}
public String getName() {
return mName;
}
}
3 Answers
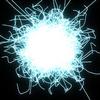
Rob Bridges
Full Stack JavaScript Techdegree Graduate 35,467 PointsHey John,
What this challenge is wanting you to do is add a new method addCart (We are keeping the old method the same) that takes only a Product as an argument, much like the original method did. Instead of writing out the same method that's already been defined, we can put in the body of the method the already previously defined addCart method that did take an int argument, it would look something like below.
We can do this by
public void addItem(Product item) {
addItem(item, 1);
}
This is called method overloading, basically what that means is that Java will see addItem(Product item, int Quanity) and addItem(Product item) as two unique methods, because they take different argument parameters, which is actually very useful.
Also be sure to uncomment the line cart.addItem(dispenser); in the Example.java
So here's what ShoppingCart.java should look like once we're done.
public class ShoppingCart {
public void addItem(Product item, int quantity) {
System.out.printf("Adding %d of %s to the cart.%n", quantity, item.getName());
/* Other code omitted for clarity */
}
public void addItem(Product item) {
addItem(item, 1);
}
}
So what did we accomplish with this? Well, say a user just wants to add a single item addItem(apple); It would add it to the cart with the assumed quantity of one. This also makes the program more user friendly instead of throwing an error message if the user forgets to add a quantity. On top of that it gives the user freedom, they can also type in addItem(apple, 10); if they want multiples of them. Both of these commands would pass since we have a method prepared for both situations.
Thanks, let me know if this doesn't help.

Josh Gold
12,207 PointsThanks for the info. I wasn't sure even where to start on this particular challenge, but now I have a better understanding of method overloading.
I found this challenge more difficult than the others so far in this course, as it was a more open-ended question.

Jess Sanders
12,086 PointsExample.java has a line of code that is commented out
cart.addItem(dispenser);
If all you do is uncomment this line and check your work, you get a compiler error. We need to write a second addItem method that only takes one argument, rather than two.
So, in ShoppingCart.java, we can copy and paste the existing addItem method, but remove the second argument. We also need to change what is printed, because we know that we are Adding 1 of the item to the cart.
John Hammett
6,173 PointsJohn Hammett
6,173 PointsI did what you said but it still wouldn't let me pass about 29 syntax errors popped up, I also uncommented the lines beneath the ShoppingCart line.
Rob Bridges
Full Stack JavaScript Techdegree Graduate 35,467 PointsRob Bridges
Full Stack JavaScript Techdegree Graduate 35,467 PointsHey John, I check code to make sure it runs before I post it, that's very weird. Were you sure to uncomment the line
// cart.addItem(dispenser);
so that it's
cart.addItem(dispenser);
If so can you please post your code again, or some of the errors that it is giving you?
John Hammett
6,173 PointsJohn Hammett
6,173 PointsYep I uncommented all the lines lol, my bad thanks you so much!
Rob Bridges
Full Stack JavaScript Techdegree Graduate 35,467 PointsRob Bridges
Full Stack JavaScript Techdegree Graduate 35,467 PointsNo problem! I'm glad I could help. Its amazing what one line can do to our code.