Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial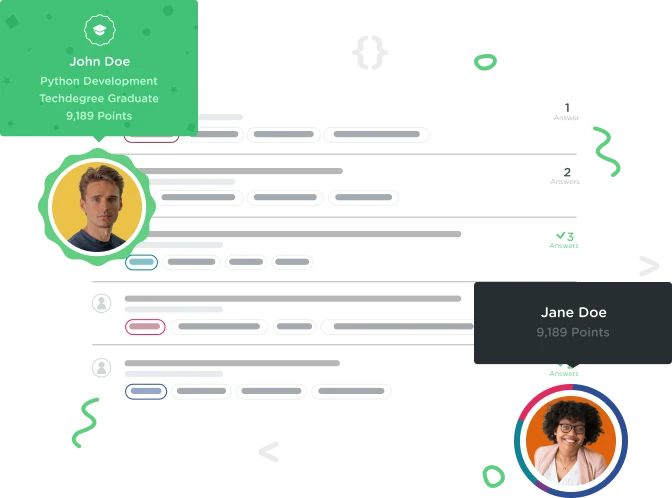

Jordan Man
2,736 PointsCan someone please help me solve this?
using a for each loop; create a getTileCount Method, run through the possible letters and any repeating letters increment on a counter.
public class ScrabblePlayer {
private String mHand;
public ScrabblePlayer() {
mHand = "";
}
public String getHand() {
return mHand;
}
public void addTile(char tile) {
// Adds the tile to the hand of the player
mHand += tile;
}
public boolean hasTile(char tile) {
return mHand.indexOf(tile) > -1;
}
public boolean getTileCount(char tile)
for (char tile : mHand.ToCharArray){
++frequency[mHand.indexOf(tile) >= 0];
} return frequency;
}
}
1 Answer
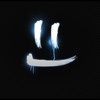
Grigorij Schleifer
10,365 PointsHi Jordan,
let us look at my for loop suggestion:
The for-loop creates a new variable (here char type variable called "character") and stores every character from the mHand inside of it using the method toCharArray() to split the String mHand into single characters.
So the loop goes over every sigle character inside mHand and you can compare your argument "tile" with every character inside mHand.
public int getTileCount (char tile) {
int count = 0;
// create an integer to count how many characters are equal to the argument "tile"
for (char character: mHand.toCharArray()) {
// create a for loop and split mHand in an array of chars
if (character == tile) {
// compare every char from mHand with the argument "tile"
count++;
// increase the conut if tile has been found in mHand
}
}
return count;
// return how many "tiles" are inside mHand
}
I commented your code so you can see the errors and compare them with my code suggestion:
public boolean getTileCount(char tile) { // curly bracket added
for (char tile : mHand.ToCharArray()){ // method parenthesis added
int frequency = 0; // counter added
if (frequency == tile) { // condition modified
frequency++; // increase the counter
} return frequency;
} // close the method
Does it make sense?
Please don´t hesitate to ask again. Here are so many cool members who will help you
Grigorij
Jordan Man
2,736 PointsJordan Man
2,736 PointsYay! this is brilliant. I understand it very well now. thank you so much for your help.
Jordan Man
2,736 PointsJordan Man
2,736 Pointscould you please clarify the meaning of "public int" at the top of the screen as opposed to a normal method. thanks
Grigorij Schleifer
10,365 PointsGrigorij Schleifer
10,365 PointsHey there,
I overlooked it, sorry
This is a so called method return type. When you call a method you want that it does something. Maybe counting how many peaple are in the bus/boat or something similar like how many peaple are online at treehouse (sorry for this silly examples).
The number of peaple will be stored inside an Integer right and this number stays in the method without beeing visible to other code. But if you plan to use the amount of peaple somewhere else maybe for a second method that proofs if the bus or boat are full and no more peaple can go on you will need to display this integer for other methods via returning it to the code outside using a return statement:
return _type that should be returned_;
Very important to mention is that the type you are returning must be the same as the return type of your method.
Lets go back to the challenge. You have declared a method that has a boolean (true or false) return type. But the challenge wants you to count the number of tiles inside mHand and return it. This number is an Integer so the return type must be integer.
Makes sense?
Grigorij