Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial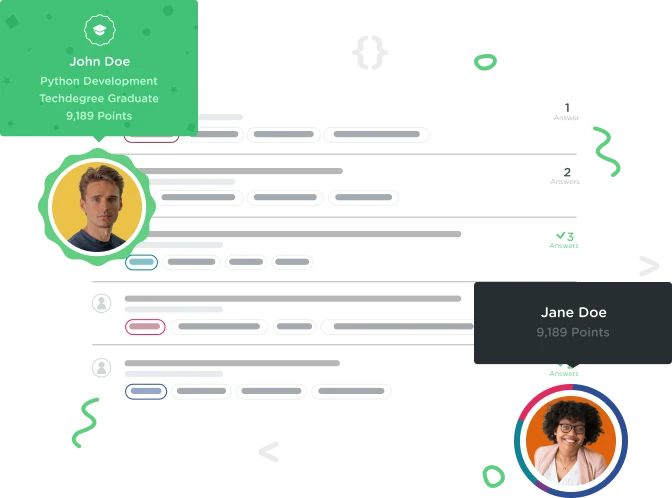
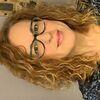
Rachael Zordan
1,379 PointsCan steps 2 and 3 be combined, or does it create messy code? (I've included my solution to the challenge as an example.)
// Collect input from a user
const userInput = prompt('Zoltan asks, "How many different countries have you visited?"');
// Convert the input to a number // Use Math.random() and the user's number to generate a random number
const numberOfCountries = Math.floor(Math.random()* parseFloat(userInput))+1;
// Create a message displaying the random number
console.log(Zoltan forsees that you will enjoy at least ${numberOfCountries} foreign love affairs in your lifetime.
);
EDITED TO ADD: The code above seemed to work until I continued the challenge by creating an conditional statement to avoid a result of NAN. By combining steps 2 and 3 of the code comments, the conditional statement did not run the ELSE clause, but seemed to treat the first clause as true. Can conditional statements not run parseFloat()?
1 Answer
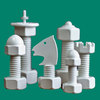
Steven Parker
231,140 PointsDid you perhaps put an assignment in the conditional instead of a comparison? Any non-empty string will be interpreted as "true".
If that's not the issue, show the final version that you are having the trouble with.
Rachael Zordan
1,379 PointsRachael Zordan
1,379 PointsI believe that I figured out my mistake. In the conditional statement I had the wrong variable, referencing the user's response instead of the converted variable. When I ran the program, it would always run the conditional as true. If the input was NaN, it would still run as true.
My if-statement should have referenced (parseFloat(userInput)) instead of just (userInput)
When I made that change, the code worked. Below is my working code. (Would it have been cleaner code for me convert the string to a number separately from the if statement as was demonstrated in the video? Or was it okay that I'd combine these two steps?)
const userInput = prompt('Zoltan asks, "How many different countries have you visited?"');
if (parseFloat(userInput)) { const numberOfCountries = Math.floor(Math.random()* parseFloat(userInput)) + 1;
console.log(
Zoltan foresees that you will enjoy at least ${numberOfCountries} foreign love affairs in your lifetime.
); } else { console.log("Zoltan requires you to provide a number. Please try again."); }Steven Parker
231,140 PointsSteven Parker
231,140 PointsConverting the string separately fulfills the "DRY" best-practice principle ("Don't Repeat Yourself") by requiring the conversion only once. But you can also do this by combining the conversion with the prompt,
Also, testing the value directly is not a good way to check for a valid number, since 0 will also fail. But the "isNaN" function is available to do that job:
Also, since folks are not likely to visit fractions of a country, you could use "parseInt" here instead of "parseFloat".
And for future questions, be sure to format any code using Markdown formatting. (as I did here), and provide a link to the course page you are working with.