Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial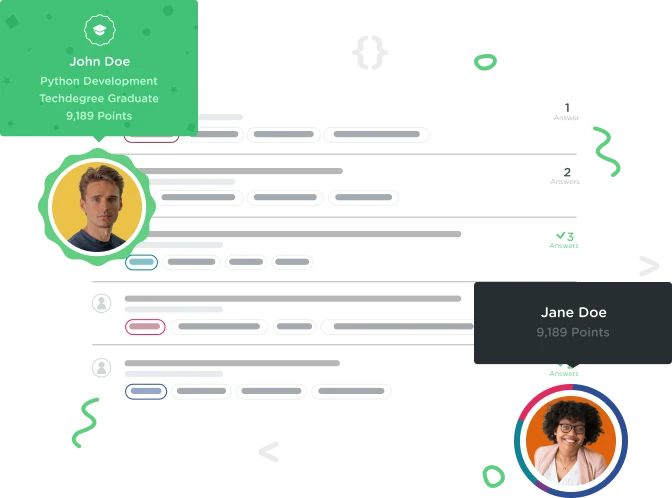

Udrea Mihai
3,465 Points...Cannot find symbol class FactBook
public class FunFactsActivity1 extends AppCompatActivity { 1 private FactBook mFactBook = new FactBook(); // Declare our view variables private TextView mFactTextView; private Button mShowFactButtom;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_fun_facts1);
// Assign the Views from the layout file to the corresponding variables
mFactTextView = (TextView) findViewById(R.id.factEditText);
mShowFactButtom = (Button) findViewById(R.id.factButton);
View.OnClickListener listener = new View.OnClickListener() {
@Override
public void onClick(View v) {
2 String fact = mFactBook.getFact();
// Update the screen with our dynamic fact
mFactTextView.setText(fact);
1 private FactBook mFactBook = new FactBook(); FactBook and FactBook() from this line are red and the suggestions is: "cannot resolve symbol FactBook"
2 String fact = mFactBook.getFact(); getFact from here is also red and suggestion is: "cannot resolve the method getFact()"

Udrea Mihai
3,465 PointsThe class FactBook.java was created and is in the same package with FunFactsActivity1, this is the code Louis Sankey. Thank you for help!
package com.example.mihai.funfacts1;
import java.util.Random;
public class FactBook { // Fileds (Member Variables) - Properties about the objects private String[] mFacts = { "Ants stretch when they wake up in the morning.", "Ostriches can run faster than horses.", "Olympic gold medals are actually made mostly of silver.", "You are born with 300 bones; by the time you are an adult you will have 206.", "It takes about 8 minutes for light from the Sun to reach Earth.", "Some bamboo plants can grow almost a meter in just one day.", "The state of Florida is bigger than England.", "Some penguins can leap 2-3 meters out of the water.", "On average, it takes 66 days to form a new habit.", "Mammoths still walked the earth when the Great Pyramid was being built." }; // Methods - Action the object can take public String getFact(){
String fact = "";
// Randomnly select a fact
Random randomGenerator = new Random();
int randonNumber = randomGenerator.nextInt(mFacts.length);
fact = mFacts[randonNumber];
return fact;
}
}
5 Answers

Aniketh Suresh
2,349 PointsHi Udrea Mihai , I had this exact problem and I realized my mistake which I think is the same as yours. I think you added your class to the folder with the Application Test instead of the folder with the Main Activity. On relocating your class you'll see that android Studio is able to locate the FactBook Class and you'll be able to run your program :)

Udrea Mihai
3,465 PointsI didn't check your answer but is most probably a good one if I solve the problem making again same class. Thanks!

Gavin Ralston
28,770 PointsIf the words FactBook are highlighted in the IDE, try placing your cursor there, hitting alt-Enter and seeing if it suggests automatically adding the import for you. If it does, go ahead and select that option and hit enter so it's added to your import statements and you can access it.
If not, or you get suggestions about building the class yourself, then post your code here so it can be reviewed.

Udrea Mihai
3,465 PointsWhen i press Alt-Enter it gives me the opportunity to create class FactBook even the class is allready created.
package com.example.mihai.funfacts1;
import android.support.v7.app.AppCompatActivity; import android.os.Bundle; import android.view.View; import android.widget.Button; import android.widget.TextView;
import java.util.Random;
public class FunFactsActivity1 extends AppCompatActivity { private FactBook mFactBook = new FactBook(); // Declare our view variables private TextView mFactTextView; private Button mShowFactButtom;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_fun_facts1);
// Assign the Views from the layout file to the corresponding variables
mFactTextView = (TextView) findViewById(R.id.factEditText);
mShowFactButtom = (Button) findViewById(R.id.factButton);
View.OnClickListener listener = new View.OnClickListener() {
@Override
public void onClick(View v) {
String fact = mFactBook.getFact();
// Update the screen with our dynamic fact
mFactTextView.setText(fact);
}
};
mShowFactButtom.setOnClickListener(listener);
}
}

Gavin Ralston
28,770 PointsDoes this behavior persist even after you clean the project and rebuild it, or even if you restart Android Studio?

Udrea Mihai
3,465 PointsI tryed to clean and close android studio but is not working.

Gavin Ralston
28,770 PointsOne option would be to get your project set up with version control and post it on github. It'd be a lot easier to troubleshoot something like that if we could check it out and throw it into our own development environment.
IntelliJ should definitely be able to locate a class in the same project (and namespace even) without trouble and set up the import for you.
When you add the import statement yourself, does it show up at least? I don't see it in the code you posted, all I see are the following:
import android.support.v7.app.AppCompatActivity;
import android.os.Bundle;
import android.view.View;
import android.widget.Button;
import android.widget.TextView;
import java.util.Random;
Try typing out the import statement in there, just for kicks.
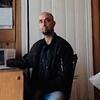
Louis Sankey
22,595 Pointshmm, I'm not sure what is causing the problem. It shouldn't be anything too complicated. I will try to come back to it later in the day if no one else has provided an answer by then.

Udrea Mihai
3,465 PointsI solved the problem by creating again the class FactBook but with another name and after I renamed it.

Christopher Kehl
18,180 Pointsmake sure your java class is FactBook and not factBook
Louis Sankey
22,595 PointsLouis Sankey
22,595 Pointshave you also created FactBook.java? Right now you are showing us code from one of the necessary files, which is FunFactsActivity.java. You also need to create FactBook.java. Once you have done that, please post your code and I will help you figure out what is going wrong.