Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial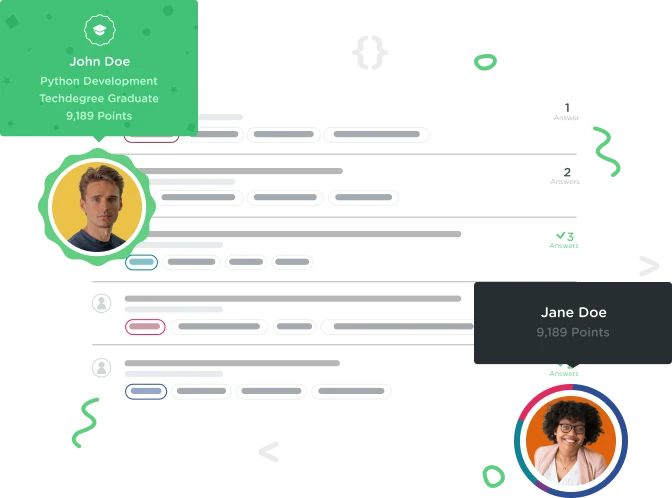

Alfredo Prince
6,175 PointsCan't figure out what's wrong with Example.java
on line 12 I added the author, title, and description but i still get the following error:
./Example.java:12: error: cannot find symbol
ForumPost post = new ForumPost(author,title ,description);
^
symbol: variable title
location: class Example
./Example.java:12: error: cannot find symbol
ForumPost post = new ForumPost(author,title ,description);
^
symbol: variable description
location: class Example
./Example.java:13: error: non-static method addPost(ForumPost) cannot be referenced from a static context
Forum.addPost(post);
^
3 errors
public class ForumPost {
private User mAuthor;
private String mTitle;
private String mDescription;
public User getAuthor() {
return mAuthor;
}
public String getTitle() {
return mTitle;
}
public String getDescription() {
return mDescription;
}
public ForumPost(User author, String title, String description) {
mAuthor = author;
mTitle = title;
mDescription = description;
}
// TODO: We need to expose the description
}
public class User {
private String mFirstName;
private String mLastName;
public String getFirstName (){
return mFirstName;
}
public String getLastName (){
return mLastName;
}
public User(String firstName, String lastName) {
// TODO: Set the private fields here
mFirstName = firstName;
mLastName = lastName;
}
}
public class Forum {
private String mTopic;
public String getTopic() {
return mTopic;
}
public Forum (String newTopic){
mTopic = newTopic;
}
public void addPost(ForumPost post) {
System.out.printf("New post from %s %s about %s.\n",
post.getAuthor().getFirstName(),
post.getAuthor().getLastName(),
post.getTitle());
}
}
public class Example {
public static void main(String[] args) {
System.out.println("Starting forum example...");
if (args.length < 2) {
System.out.println("first and last name are required. eg: java Example Craig Dennis");
}
Forum forum = new Forum("Java");
//Take the first two elements passed args
User author = new User(args[0],args[1]);
//Add the author, title and description
ForumPost post = new ForumPost(author,title ,description);
Forum.addPost(post);
}
}
2 Answers

Lukas Dahlberg
53,736 PointsYou need to pass in a string to Forum post for the title and description.
ForumPost post = new ForumPost(author,title ,description)
should be (you can use whatever strings you like0:
Forum post = new ForumPost(author, "This challenge", "Stumped me");
The static error at the end should go away after you do this.

Lukas Dahlberg
53,736 PointsIf you did the Strings in the Example.class, that should work. If you didn't, and put them in, say, ForumPost, instead, they won't port over to Example.class (unless you bring them in, which isn't a good idea).
As for changing addPost to static, that won't work the way you intend it to work. A static class is done on the class itself -- not on the individual instance of it. So, if you create a static forum post, it will never be associated to any particular object, just the class itself. We don't want that. (A good reference for static uses is here:http://stackoverflow.com/questions/2671496/java-when-to-use-static-methods ) This is definitely one of the harder aspects of Object Oriented Programming, but it's also very powerful.
Does that help?

Alfredo Prince
6,175 PointsI see what you mean and I understand ite but what worked is doing a combination of what I did with addPost
method and putting the strings in for title and description. Like this:
Forum post = new ForumPost(author, "Can't believe", "...this sh");
also doing the following also worked:
String title = " ";
String description = " ";
So I am still stumped hahaha
Alfredo Prince
6,175 PointsAlfredo Prince
6,175 PointsYour method seems more correct than mine. Can you help me understand why?
I did this right before the error line:
then I changed
public void addPost(ForumPost post) {
to
public static void addPost(ForumPost post) {