Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial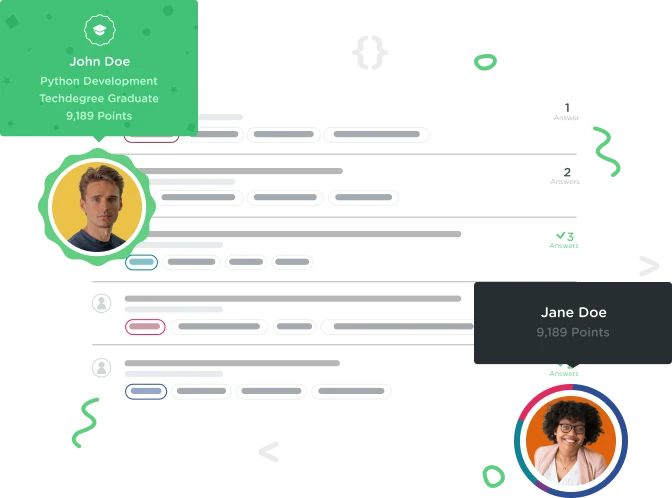

Oswaldo Zarate
35,612 PointsCan't send selected checkbox type values to my email.
I'm working on a website that has a form. In the form there's some options that can be selected. I used
<input type="checkbox" name="services[]" value="service name">
for each of the available options.
Now, when I try to submit the form, everything seems to work if I don't select any option, but once I click on a checkbox and try to submit the form there's an error. I checked my code and it displays the error message given by this method:
if(!isset($error_message)) {
foreach( $_POST as $value ){
if( stripos($value,'Content-Type:') !== FALSE ){
$error_message = "there was an error";
}
}
}
I think it takes the option as malicious code.
Any ideas how I can solve this? Thank you
3 Answers
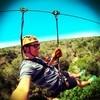
thomascawthorn
22,986 PointsIt's because you're sending through an array here:
<input type="checkbox" name="services[]" value="service name">
I believe if content type is left out of a value passed through in POST, it's a string. As soon as you start passing through arrays, it will say something like "Content-Type: array" (not necessarily this exactly!).
I imagine you picked up this validation from the shirts4mike tutorial?
With this line, you're trying to validate string as 'things without a content type'. However, in your app it's totally valid for the 'services' (p.s. your shouldn't this be 1 or 0?) to be an array of values.
You could say:
<?php
$validateAsString = ['first_name', 'last_name'];
foreach($validateAsString as $field) {
if( stripos($_POST[$field],'Content-Type:') !== FALSE ){
$error_message = "there was an error";
}
}

Oswaldo Zarate
35,612 PointsThanks, I think you showed me the right path. First I converted the POST value only for the checkboxes to a string like this:
<?php
$_POST["services"] = implode(", ", $_POST["services"]);
?>
and now the form submits. I just hope this is a good practice.
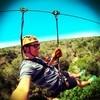
thomascawthorn
22,986 PointsAwesome!
Hmm, I wouldn't set anything to $_POST - I prefer only to get values from $_POST as opposed to set values.
You could however sotre $_POST in an a new variable:
<?php
$formData = $_POST;
$formData['services'] = implode(', ', $_POST['services']);

Oswaldo Zarate
35,612 PointsI tried:
<?php
foreach( $_POST[""] as $value ){
if( stripos($value,'Content-Type:') !== FALSE ){
$error_message = "there was an error";
}
}
Because otherwise that statement would check each $_POST regardless of POST SERVICES being in a variable. But I don't know if using Post[""] will still check for other input values like:
<input type="text" name="name">
because those are not arrays.

Oswaldo Zarate
35,612 PointsBy the way, I did took it from the shirts 4 mike exercise. And I would like to be able to check if each POST value has a content-type because I understand it makes the form more secure. Thank you for your help.
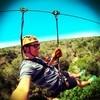
thomascawthorn
22,986 PointsThat's cool, but your current method won't work. It's a while ago since I ran through the course, but I had a go at doing it this way. It's not great.. but might point you in the right direction!
<?php
$validateAsString = ['first_name', 'last_name', 'username'];
$validateAsRequired = ['first_name', 'last_name', 'user_name', 'services'];
$formData = $_POST;
foreach($formData as $field => $input) {
if (in_array($field, $validateAsRequired)) {
// validate it's not empty etc...
}
if (in_array($field, $validateAsString) {
// validate as string / content type
}
}

Oswaldo Zarate
35,612 PointsI think I finally got it. I started by placing the POST values inside the formData variable and then I imploded the services array like this:
<?php
$formData = $_POST;
$formData["services"] = implode(", ", $formData["services"]);
And then i changed the foreach statement like this:
<?php
if(!isset($error_message)) {
foreach( $formData as $value ){
if( stripos($value,'Content-Type:') !== FALSE ){
$error_message = "there was an error";
}
}
}
Thank you.
shawn stokes
5,651 Pointsshawn stokes
5,651 Pointswhat was the error message? and the form code and form processing would help to track down this also.