Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial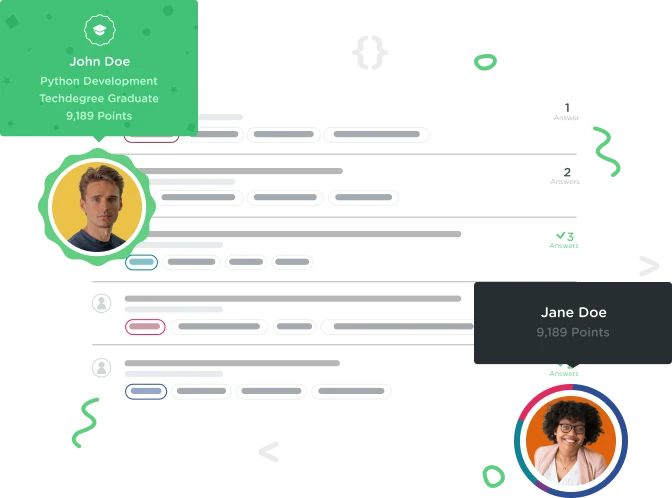

Haldon Francis
619 PointsChallenge Task 1 of 1
using System;
class Program
{
// YOUR CODE HERE: Define a method named Multiply. Remember
// to use the "static" and "void" keywords: "static void
// Multiply" (without quotes). Multiply should take two
// "double" values as parameters.
static void Multiply (double firstParameter, double secondParameter)
{
Console.WriteLine(firstParameter * secondParameter);
}
static void Main(string[] args)
{
// YOUR CODE HERE:
// Call Multiply with two arguments: 2.5 and 2.
// YOUR CODE HERE:
// Call Multiply with two arguments: 6 and 7.
Console.WriteLine(Multiply);
}
}
3 Answers
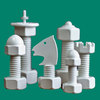
Steven Parker
241,970 PointsInstead of calling "WriteLine" and passing "Multiply" as the argument, you need to call "Multiply" and pass two number argments. You'll need to do this two times using different arguments.
The instructions say "make a call to Multiply with the arguments 2.5 and 2." and "Then call Multiply again with the arguments 6 and 7."
There are also reminder comments in the code itself.

Jordan young
Courses Plus Student 5,277 PointsThis code worked for me as well, but I couldn't determine why mine wouldn't work... The"bummer" here didn't help me .

Laine Dorchester
206 PointsHi all,
Why am I getting a bummer message on this one. When I press show preview it gives me 5 and 42 but it says bummer we ran the multiply function and got a different result.
class Program { static double Multiply(double first, double second) { return first * second; }
static void Main(string[] args)
{
Console.WriteLine(Multiply(2.5, 2));
Console.WriteLine(Multiply(6,7));
}
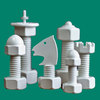
Steven Parker
241,970 PointsNext time start a new question instead of asking one in an answer. Otherwise, it's likely to only be seen by someone who asked or answered the old question.
But the instructions say that WriteLine should be called inside your Multiply method. Then you will only add calls to your method in Main (without nesting them in other calls).
The inline comments also say that the new method should be declared as static void Multiply
(so id does not return anything).
Hassainate Souhaib
675 PointsHassainate Souhaib
675 Pointsusing System;
class Program {
} it works on (treehouse Workspaces) but does not work on Code Challenge ! I don't know why!!!!
Steven Parker
241,970 PointsSteven Parker
241,970 PointsI pasted this code directly into the challenge and it passed.
Hassainate Souhaib
675 PointsHassainate Souhaib
675 PointsActually it doesn't , when i check it and still got {Bummer }
Steven Parker
241,970 PointsSteven Parker
241,970 PointsTry again after freshly restarting your browser.
Hassainate Souhaib
675 PointsHassainate Souhaib
675 Pointsit works , i don't know how (i kept the same solution as yesterday ), thank you