Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial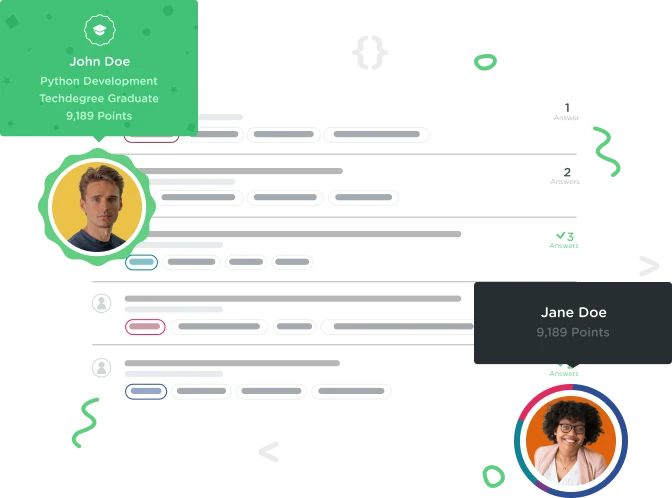
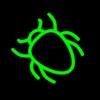
Matthew Stanciu
4,222 PointsChanges in workspace not reflecting to the program
Hey,
I noticed something really strange with my workspace. After watching and rewatching this video, I can't see anything wrong with my workspace. I added everything that this video talked about. However, when I run Hangman.java, it doesn't reflect the changes. When I guess the same letter, it still says there are 7 tries left, and when I guess a wrong letter more than once, it just takes away more than once. It seems like nothing has changed, but I still have the code that says that it should change.
Game.java:
public class Game {
public static final int MAX_MISSES = 7;
private String mAnswer;
private String mHits;
private String mMisses;
public Game(String answer) {
mAnswer = answer;
mHits = "";
mMisses = "";
}
private char validateGuess(char letter) {
letter = validateGuess(letter);
if (! Character.isLetter(letter)) {
throw new IllegalArgumentException("A letter is required!");
}
letter = Character.toLowerCase(letter);
if (mMisses.indexOf(letter) >= 0 || mHits.indexOf(letter) >= 0) {
throw new IllegalArgumentException(letter + " has already been guessed!");
}
return letter;
}
public boolean applyGuess(char letter) {
boolean isHit = mAnswer.indexOf(letter) >= 0;
if (isHit) {
mHits += letter;
} else {
mMisses += letter;
}
return isHit;
}
public String getCurrentProgress() {
String progress = "";
for (char letter: mAnswer.toCharArray()) {
char display = '-';
if (mHits.indexOf(letter) >= 0) {
display = letter;
}
progress += display;
}
return progress;
}
public int getRemainingTries() {
return MAX_MISSES - mMisses.length();
}
}
Prompter.java:
import java.io.Console;
public class Prompter {
private Game mGame;
public Prompter(Game game) {
mGame = game;
}
public void play() {
while (mGame.getRemainingTries() > 0) {
displayProgress();
promptForGuess();
}
}
public boolean promptForGuess() {
Console console = System.console();
boolean isHit = false;
boolean isValidGuess = false;
while (! isValidGuess) {
String guessAsString = console.readLine("Enter a letter: ");
char guess = guessAsString.charAt(0);
try {
isHit = mGame.applyGuess(guess);
isValidGuess = true;
} catch (IllegalArgumentException iae) {
console.printf("%s. Please try again.\n", iae.getMessage());
}
}
return isHit;
}
public void displayProgress() {
System.out.printf("You have %d tries left to solve: %s\n",
mGame.getRemainingTries(),
mGame.getCurrentProgress());
}
}
1 Answer

Craig Dennis
Treehouse TeacherLooks like you put the validateGuess
call in the wrong method. It should be called in applyGuess
...it looks to be never called ;)
Matthew Stanciu
4,222 PointsMatthew Stanciu
4,222 PointsThank you so much for your help! It finally works! I can't believe I didn't notice that the validateGuess call was in the the wrong method.
Craig Dennis
Treehouse TeacherCraig Dennis
Treehouse TeacherNo problem! Took me a while too ;)