Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial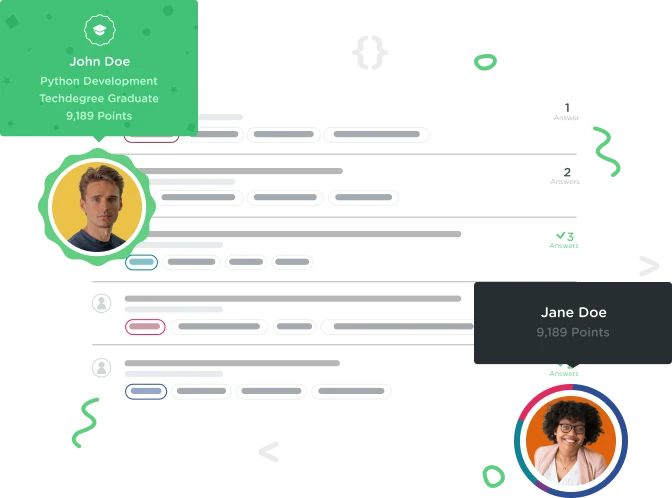
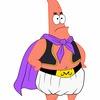
<noob />
17,063 PointsCheck if my solution is correct in a js question
the question: Write a program that generates 10 random numbers.
The numbers should be generated so each number is greater than the ones generated before.
To simplify, generate the first number n so it is between (0→1000), and each subsequent number will be in the range of ( n → n+1000)
my code:
count = 10;
let previous = null;
let randomNum;
while(count > 0) {
if(!randomNum) {
randomNum = Math.floor(Math.random() * Math.floor(1000));
}
if(!previous) {
previous = 0;
}
randomNum = Math.floor(Math.random() * Math.floor(1000));
if(randomNum < previous) {
randomNum += 1000;
}
console.log("the random num is ", randomNum);
console.log("previous num ", previous);
previous = randomNum;
count--;
}
1 Answer
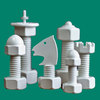
Steven Parker
241,809 PointsThis isn't meeting the stated objective. For example, I ran it and got this series of numbers: 36, 53, 709, 1489, 1019, 1476, 1413, 1127, 1007, 1076.
Adding a fixed value to a random number chosen with the same range isn't likely to produce a higher number more than just one time.
Perhaps a better solution would be to use the standard "high/low" random formula, but each time use the previously chosen number (plus one) as the "low" value, and that same value plus 1000 as the "high" value. The basic formula could then be simplified to this:
randomNum = Math.floor(Math.random() * 1001) + previous + 1;