Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial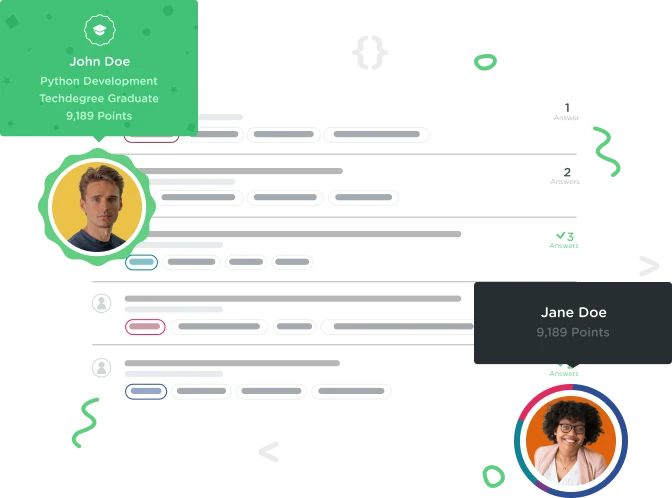

cam
7,468 PointsCode Challenge: How to deal with different number of arguments?
I'm just not getting it. I'm trying to have a method before the original method that scans the length of the second argument to determine if it is present, so that a default value can be put in. I'm wondering how to get around the fact that methods cannot have the same classes of arguments.How is it possible to give a Product and a int to the original method, if I can't use Product and int? Code below. Can someone please give me a hint?
public class ShoppingCart {
public Product addItem(Product test1, int test2) {
if(String.valueOf(test2).length()==0) {
return addItem(test1,1);
} else {
return addItem(test1,test2);
}
}
public void addItem(Product item, int quantity) {
System.out.printf("Adding %d of %s to the cart.%n", quantity, item.getName());
/* Other code omitted for clarity */
}
}
2 Answers
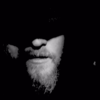
Micah Kline
17,831 PointsMethods can not support the same param values but can have different ones its the Object type that matters not the variable name. In the main Example.java it wants you to uncomment the line cart.addItem(dispenser);
So create a method that supports just that and assume that quatity is 1. Example Below..
public void addItem(Product item){
// call the other add Item with a defaul quatity of one like
addItem(item, 1); // this calls the method with the Products, int signature
}
public void addItem(Product item, int quantity){
// code omited
}

cam
7,468 PointsThanks for the response!

Jess Sanders
12,086 PointsHere's my simplest explanation of what's going on in the 'defaulting parameters' code challenge:
Example.java has a line of code that is commented out
cart.addItem(dispenser);
If all you do is uncomment this line and check your work, you get a compiler error. We need to write a second addItem method that only takes one argument, rather than two.
So, in ShoppingCart.java, we can copy and paste the existing addItem method, but remove the second argument. We also need to change what is printed, because we know that we are "Adding 1 of" of the item to the cart.
cam
7,468 Pointscam
7,468 Points