Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial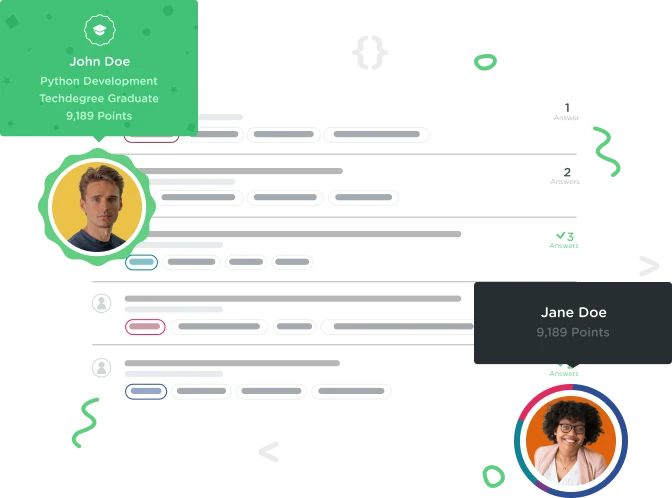
Miguel Nunez
3,266 PointsCode Challenge PHP
Use the $fullname variable to display the following string to the screen. Use an escape character to add a newline to the end of the string. 'Rasmus Lerdorf was the original creator of PHP'
$firstName = 'Rasmus'; $lastName = 'Lerdorf'; $fullname = $firstName .' '.$lastName;
<?php
//Place your code below this comment
?>
2 Answers
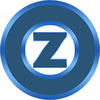
Daniel Gauthier
15,000 PointsHey Miguel,
This section of the challenge is trying to make sure you know how to use escape characters in PHP.
One thing to remember is that escape characters in PHP need to be placed into double quotes for them to work.
The working code is:
<?php
//Place your code below this comment
$firstName = 'Rasmus';
$lastName = 'Lerdorf';
$fullname = $firstName . ' ' . $lastName;
echo "$fullname was the original creator of PHP\n";
?>
Good luck with the course!
Miguel Nunez
3,266 PointsSo are you saying that they are used for different desired intended character structures ? Like grammar quotes etc...? and thanks and so the n one what they mean when they say its used for a new line are they referring spacing distance aka moving it down ?
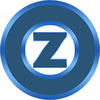
Daniel Gauthier
15,000 PointsHey again Miguel,
Yes, it effectively tells the code processor (the parser) to treat the character after the \ (escape character) differently than it normally would. It's like hitting the shift key on your keyboard :D
As for the \n new line escape sequence, you're simply saying "Don't print this n, create a new line below this one."
Daniel Gauthier
15,000 PointsDaniel Gauthier
15,000 PointsYou could also use a concatenation operator if you prefer, which would also work. Your final code would then look something like this:
Miguel Nunez
3,266 PointsMiguel Nunez
3,266 PointsThank you I appreciate your answer but I have another question what is the purpose of escape sequences in general I know there are different ones out there but its really hard to find sources on google that makes sense to newbies like me to understand because they use a lot of assume languages and big fancy words.
Daniel Gauthier
15,000 PointsDaniel Gauthier
15,000 PointsHey again Miguel,
An escape character basically tells the code processor to treat the character after the escape character (the backslash) differently than it normally would.
As a really simple example, let's assume that you need to write the line:
'Jen's awesome!'
but for whatever reason, you couldn't use double quotes to do it.
If you were in this position and tried to use the following line of code:
echo 'Jen's awesome!';
You'd trigger an error since the second ' is telling the code processor that the string is complete.
So, to get around this quickly and easily, we simply 'escape' the second ' with the code:
echo 'Jen\'s awesome!';
Which will tell the code processor to just print the apostrophe as an apostrophe and not to recognize it as the closing apostrophe of the string.
It really depends on what comes after the escape character though, since a new line can be easily triggered using the \n escape sequence.
In this case, it tells the code processor "Don't actually print out that n, just move to a new line."
If you're up to it, check out this page from the official PHP.net manual if you're interested in seeing which escape sequences are available. A few of them can come in really handy often enough to be aware of them :D
Escape Sequences
Edit: Added the actual link :D
Good luck with the course!