Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial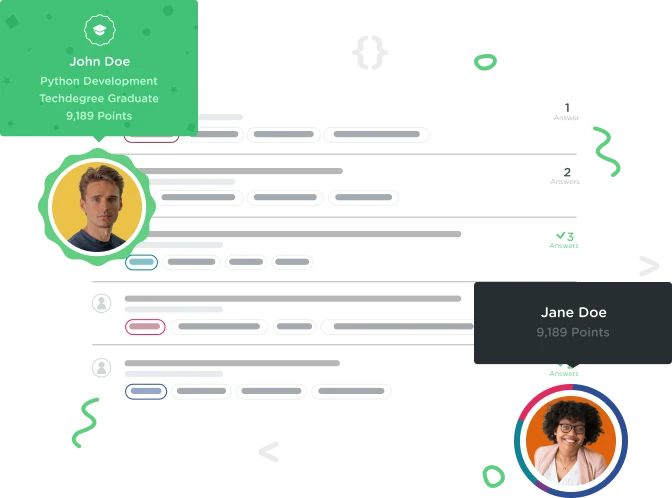
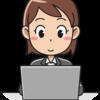
ilovecode
8,071 PointsComments won't save to mongoDB database (using nodejs, express, mongoose)
I am creating a simple blog app, and now I would like to add comments. I am using Expressjs, Mongoose, EJS, I am using a MVC framework, with models, views, controllers and routers folders.
I have tried playing around with the router the most, I feel it might be the problem.
I tried to put my project on a Treehouse workspace but it keeps crashing for some reason after I installed some node packages.
Currently I don't have a view, because I am hard coding the data I want to send to the database
My app.js
//create express function
const express = require('express');
const morgan = require('morgan');
const mongoose = require('mongoose');
const blogRoutes = require('./routes/blogRoutes');
const commentRoutes = require('./routes/commentRoutes');
//set up express app and invoke express and stor in app const
const app = express();
app.listen(3000)
// //connect to mongodb
const bdURI = 'mongodb+srv://something:somepass@nodetuts-6iczn.mongodb.net/node-tuts?retryWrites=true&w=majority';
// //deprecation warning so add 2nd parameter
mongoose.connect(bdURI, { useNewUrlParser: true, useUnifiedTopology: true })
.then(result => app.listen(8080))
.catch(err => console.log(err));
//set up view engine
app.set('view engine', 'ejs');
//middleware on static files
app.use(express.static('public'));
app.use(express.urlencoded({ extended: true }));
app.use(morgan('dev'));
//routes
app.get('/', (req, res) => {
res.redirect('/blogs');
});
app.get('/about', (req, res) => {
res.render('about', { title: 'about' });
});
//blog routes
//scope out the /blog and then remove /blog in all routes. It makes it easier if you want to change url later on
app.use('/blogs', blogRoutes);
app.use('/blogs', commentRoutes);
//404 must be last
app.use((req, res) => {
res.status(404).render('404' , { title: '404' });
});
Model:
const mongoose = require('mongoose');
const Schema = mongoose.Schema;
const commentSchema = new Schema({
blog_id: {
type: String
},
blog_name: {
type: String
},
comment: {
type: String
}
}, { timestamps: true });
const Comment = mongoose.model('Comment', commentSchema);
module.exports = Comment;
Controller
const Comment = require('../models/comment');
const comment_create_get = (req, res) => {
res.render('comments/comments', { title: 'create new blog' });
}
const post_comment = (req, res) => {
const comment = new Comment({
blog_id: 'df12345',
blog_name: 'This a a blog with comment',
comment: 'this is a comment 3'
});
comment.save()
.then(result => res.redirect('/blogs'))
.catch(err => console.log(err));
};
module.exports = {
post_comment,
comment_create_get
}
Routes
const express = require('express');
const commentController = require('../controllers/commentController');
const router = express.Router();
router.get('/', commentController.comment_create_get);
router.post('/', commentController.post_comment);
module.exports = router;
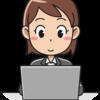
ilovecode
8,071 PointsHi Brandon White no I am not, I did the Nodejs course and Express. Can you please explain more about triggering the post route with Postman? I don't understand why I will need to do that if I can post new blogs successfully to the database and I am not using Postman, just Mongoose. It is just saving the comments that is such a problem.
EDIT: I checked throught Postman. I ran: http://localhost:8080/blogs and sent it via a Post request, and it just stays stuck on "Sending Request", as if in a loop.
Brandon White
Full Stack JavaScript Techdegree Graduate 34,662 PointsBrandon White
Full Stack JavaScript Techdegree Graduate 34,662 PointsHow are you triggering the post route? Are you using Postman?