Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial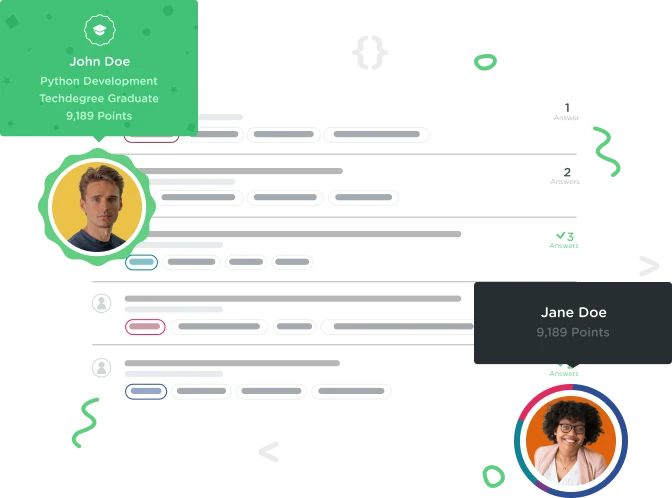

Paul Joiner
1,682 Pointscompiler error ("Preview won't load") What am I doing wrong? [Incrementing]
I'm trying to follow this using the same logic provided in the example video, but I can't get this code to compile. Even worse, the preview function won't load to tell me where my error are. Can anyone please help me?
public class GoKart {
public static final int MAX_ENERGY_BARS = 8;
private String mColor;
private int mBarsCount;
public GoKart(String color) {
mColor = color;
mBarsCount = 0;
}
public String getColor() {
return mColor;
}
public boolean charge() {
boolean isCharging true;
if (!isFullyCharged()) {
mBarsCount++;
isCharging = true;
}
return isCharging;
}
public boolean isBatteryEmpty() {
return mBarsCount == 0;
}
public boolean isFullyCharged() {
return mBarsCount == MAX_ENERGY_BARS;
}
}
4 Answers
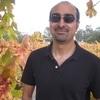
Kourosh Raeen
23,733 PointsThe charge method has a return type of void but you've changed it to boolean. Change it back to void and remove the return statement. Also, instead of the if statement you need to use a while loop. Loop until the batter is charged and inside the loop increment mBarsCount.
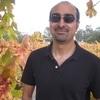
Kourosh Raeen
23,733 PointsYou have put the code in the wrong method. It should be in the charge() method, not in the isFullyCharged() method.

Paul Joiner
1,682 Pointspublic class GoKart {
public static final int MAX_ENERGY_BARS = 8;
private String mColor;
private int mBarsCount;
public GoKart(String color) {
mColor = color;
mBarsCount = 0;
}
public String getColor() {
return mColor;
}
public void charge() {
mBarsCount = MAX_ENERGY_BARS;
while(!isFullyCharged()) {
mBarsCount++;
}
public boolean isBatteryEmpty() {
return mBarsCount == 0;
}
public boolean isFullyCharged() {
return mBarsCount == MAX_ENERGY_BARS;
}
}
I've moved the while loop to the charge() method, but the code still won't compile. Is the mBarsCount=MAX_ENERGY_BARS line throwing me off? Or perhaps the fact that I'm using the isFullyCharged method? Thanks again for the help!
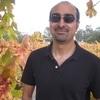
Kourosh Raeen
23,733 PointsRemove the line mBarsCount = MAX_ENERGY_BARS; Also, the while loop is missing a closing }

Paul Joiner
1,682 PointsIt worked. Thank you!
Paul Joiner
1,682 PointsPaul Joiner
1,682 Points