Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial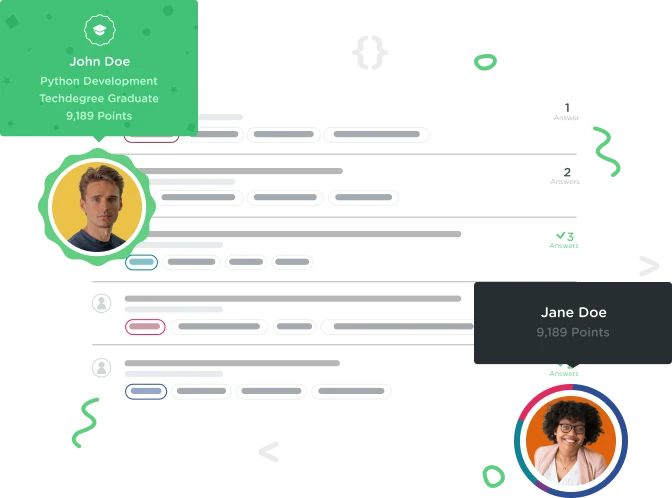

Kelly Ann Jones
1,075 PointsConditional Code
I can't figure out what is wrong with this. Can someone please tell me what I'm doing wrong?
<?php $flavor = "chocolate"; echo "<p>Your favorite flavor of ice cream is "; echo $flavor; echo ".</p>"; echo "<p><?php if ($flavor == "cookie dough") { echo "Randy's favorite flavor is cookie dough also!"; } ?></p>";
?>
Thank you, Kelly
11 Answers
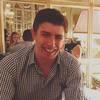
Matt Campbell
9,767 PointsYou've got too many PHP opening and closing tags. You don't want to be echoing out the if statement because you can't, instead you want to echo the result of the if statement which you already are.
I forgot that it's initially taught using echo statements with no concatenation. This is how the first, correct, part of that would look concatenated which is much nicer to read:
<?php
$flavor = "chocolate";
echo "Your favorite flavor of ice cream is: " . $flavor . ".";
?>
It's the bit after that that gets confused and messy. You've just got too many of some things in there.

Kelly Ann Jones
1,075 PointsThe only code in the entire statement i changed was to replace this sentence below which was enclosed with p tags: Randy's favorite flavor is cookie dough, also!
with this which I placed with in the original p tags:
<?php if ($flavor == "cookie dough") { echo "Randy's favorite flavor is cookie dough also!"; } ?>
I was not attempting to echo the information contained within the variable. The challenge was to make a conditional statement that would result in not showing, "Randy's favorite flavor is cookie dough, also!" sentence if my favorite flavor ice cream was not cookie dough.
So my question is not how to echo the variable, but what is wrong with my if statement? Obviously my $flavor is chocolate, so the statement should not show up. My output is creating a parse error. Any idea why?
Also, the code didn't look like that until I pasted it into the comment. It was very neat and easy to read...
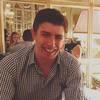
Matt Campbell
9,767 PointsTaking out the bit that's right and works, tell me what's wrong with the following:
<?php
echo "<?php if ($flavor == "cookie dough") { echo "Randy's favorite flavor is cookie dough also!"; } ?>";
?>

Kelly Ann Jones
1,075 PointsYou're asking me the same question I asked. If I knew what was wrong, I wouldn't have posted my question.
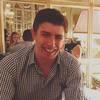
Matt Campbell
9,767 PointsI'm trying to get you to work out what's wrong with it rather than just give the answer. There's no point me just telling you because you won't have learnt anything.
Count how many <?php and ?> there are in that statement and read what I wrote about echoing out the if statement and echoing out the result.
You don't need to echo the if FUNCTION, just the result. echo and if are both parts of PHP and are already in a PHP block because you've got opening <?php before the variable declaration.
Making anymore sense?

Kelly Ann Jones
1,075 PointsI changed it to the following and it worked:
if ($flavor == "cookie dough") { echo "Randy's favorite flavor is cookie dough also!"; }
Of course the next challenge tells you to change the variable to cookie dough and see what happens. When I did, it says it looks like task three is no longer passing. Very frustrating...time to forget this one and move on. I can't see how changing the variable from chocolate to cookie dough would cause it not to "pass". It should just show the message.

Jason Anello
Courses Plus Student 94,610 PointsHi Kelly,
I take it that you've successfully passed task 3 and are now stuck on task 4. If you care to revisit this then post your code that you've used to successfully pass task 3 and then what you've put for task 4 that produces the problem. We can take a look and see where you might have gone wrong.
In general, I've found that when the error says a previous task is no longer passing that the problem is some kind of syntax error I've made in the current task.

Kelly Ann Jones
1,075 PointsI forgot to mention, I tried to work it out for about an hour before I finally asked. Not one to ever ask for help, I only asked after I was ready to give up. I didn't need to be made to feel like something of an idiot for doing so.
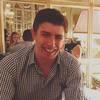
Matt Campbell
9,767 PointsYou shouldn't feel like you were made to feel like an idiot. In the forum, we prefer to put things in different perspectives and offer hints, tips and coax towards the right answer rather than just give it out. If the correct answer was just given out all the time, there's no point in having code challenges as a quick forum search would yield the answer. Nobody learns that way.
So, you've worked out what was wrong and adjusted it which is excellent and the whole point of this learning environment. :)
And you're correct, copying and pasting cookie dough from the if statement to be the value of $flavor should mean the statement resolves to true and thus show the result which is the echoed out string.

Kelly Ann Jones
1,075 PointsI am still working on this....Although I did the if statement correctly and all was fine for part 3 of 4, now the last part of the code challenge says to change the variable to match cookie dough, which I did. I reread the block of code and everything looks okay with the only difference being my $chocolate variable now reads $cookie dough. For some reason I keep getting a message that it is no longer parsing. I only changed $chocolate to $cookie dough and touched nothing else. Why I am getting an error?
Here is the code:
<?php
$flavor = "cookie dough";
echo "<p>Your favorite flavor of ice cream is ";
echo $flavor;
echo ".</p>";
if ($flavor == "cookie dough") {
echo "Randy's favorite flavor is cookie dough, also!";
}
?>

Jason Anello
Courses Plus Student 94,610 PointsHi Kelly,
I think that at some point you must have dropped the opening and closing <p>
tags around the string in the if
block. Add those back in and report back if there's still a problem.
I think it passed task 3 because the if condition was false and so the code checker probably wasn't even bothering with checking what the output of that echo statement was. In task 4, it does get echoed and the output isn't matching what's expected.

Kelly Ann Jones
1,075 PointsFinally! Thank you Jason!
Do I have to echo the p tags? I did, but I wasn't sure.
<?php
$flavor = "cookie dough";
echo "<p>Your favorite flavor of ice cream is ";
echo "$flavor";
echo ".</p>";
echo "<p>";
if ($flavor == "cookie dough") {
echo "Randy's favorite flavor is cookie dough, also!";
}
echo "</p>";
?>

Jason Anello
Courses Plus Student 94,610 PointsSorry, I wasn't very clear on where they should be placed.
If you refresh the code challenge and start over you'll see that the echo statement looks like this:
echo "<p>Randy's favorite flavor is cookie dough, also!</p>";
So the <p>
tags should be inside the string. You must have dropped them at some point because the starter code contains them and your code did not have them.
I suppose where you've echoed them would pass the code challenge but it's not a good way to do it. You're going to be echoing those p
tags to the page regardless of what happens with the if
block. Consider the case where the if
condition becomes false. You won't be echoing the Randy string but you'll still be echoing the p
tags because they're outside your if
block. You'll end up having empty p
tags on your page.
<p></p>
I hope that clears it up for you.
I think knowing what you know now, you can retake the challenge from the beginning and it will make more sense and work out better for you.

Kelly Ann Jones
1,075 PointsThank you Jason. I didn't think it looked right with the p tags echoed. It did pass the code challenge, but I will remember to do it differently in the future.
Thanks again for the response.
Kelly
Jason Anello
Courses Plus Student 94,610 PointsJason Anello
Courses Plus Student 94,610 PointsI think that because the html was stripped out of your posted code, it might have looked more problematic than it was. I think the main problem which you already figured out was that you tried to enclose your if block within php tags but you are already inside php tags so you don't have to do that.
I would suggest that you learn how to put your code in the code blocks since it will be a useful skill to have as you run into more problems. Here's a post with some tips on how to do that. https://teamtreehouse.com/forum/how-to-type-code-in-the-forum