Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial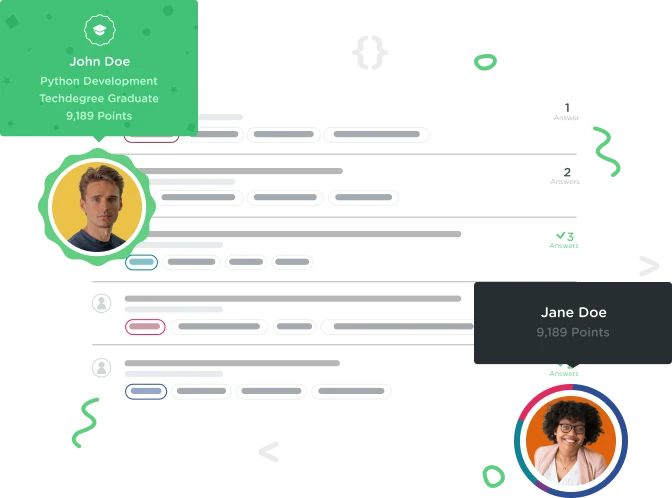
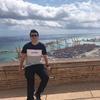
Tadjiev Codes
9,626 PointsConditional Statements IF in JavaScript
<!DOCTYPE html>
<html>
<head>
<title>Movie Fee</title>
<script>
//Declare variables to hold a user's input of 2 integers, using prompts /
var age = prompt("Enter your age", "29");
var snackOption = prompt("Enter A, B, C or D for your snack option:\nA - popcorn and pop\nB - chocolate bar and pop\nC - popcorn and candy and pop\nD - None", "B");
var coupon = prompt("Do you have a coupon?", "Yes");
var movieFee = 0.0;
var snack = 0.0;
var coupon = 0.0;
//Check the user's admission fee based on his age
if(age > 65)
{
fee = 6;
}
else if (age > 11)
{
fee = 8.5;
}
else
{
fee = 5.5;
}
//Check the user's choice of snack choice
if (snackOption ==="A")
{
snack = 7.9;
}
else if (snackOption === "B")
{
snack = 6.3;
}
else if (snackOption === "C")
{
snack = 8.9;
}
//Check if user has a coupon
if(coupon === "Yes")
{
coupon = 2;
}
//Calculate the movie fee
fee = fee + snack - coupon;
//Output the total cost of going to the movie
document.write("Total cost of going to the movie = $" + fee.toFixed(2) + "<br>");
</script>
</head>
<body>
</body>
</html>
Hi fellas, Why we don't need to declare the fee variable? That's whatI don't understand here
1 Answer
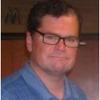
Mark Wilkowske
Courses Plus Student 18,131 PointsHi Mukhammadkhon - Variable scope. Inside the if statement the JavaScript interpreter treats: var fee = 6; as fee = 6. This is because the scope of fee is inside the if block. That is called block scope. Maybe your question comes from watching an older JavaScript course but today with ES6 we do want to use let/const in declaring block scope variables. This article covers those changes.
https://www.codepunker.com/blog/the-basics-of-variable-scope-in-javascript
Tadjiev Codes
9,626 PointsTadjiev Codes
9,626 PointsAlright, Thanks for the response) Really good to know the difference. So as per the new ES6, we better declare the variables with the keywords by the rules.