Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial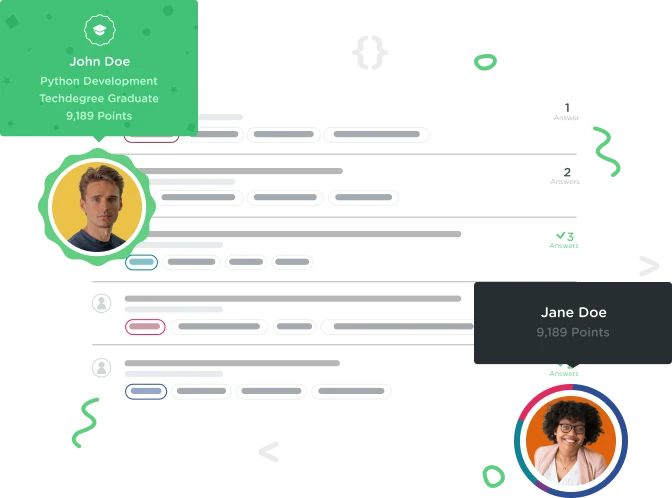

ammarkhan
Front End Web Development Techdegree Student 21,661 Pointsconsole runs my code fine
While i ran the code in console, it works fine. But not here, why?
function max(value1, value2) {
if (value1 > value2) {
return value1;
} else {
return value2;
}
}
max(15,25);
alert(Math.random));
2 Answers
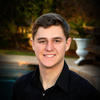
Colton Ehrman
Courses Plus Student 5,859 PointsYou need to alert your function if you want to see it.
alert(max(15,25));
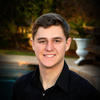
Colton Ehrman
Courses Plus Student 5,859 PointsOr you could save your result and alert it
var maxResult = max(15,25);
alert(maxResult);
ammarkhan
Front End Web Development Techdegree Student 21,661 Pointsammarkhan
Front End Web Development Techdegree Student 21,661 PointsWhat's the difference between the code you wrote and i wrote?
Colton Ehrman
Courses Plus Student 5,859 PointsColton Ehrman
Courses Plus Student 5,859 PointsThe difference is you just called the function max, which will return the value, but then get rid of it cause your not doing anything with it, you're just calling it. You need to either save the result of calling your function so you can do something with it later, or just use the function call inside alert which will display the result immedietly then get rid of it.
So really, your code is running, it just doesn't seem like it is because you aren't displaying anything.
Colton Ehrman
Courses Plus Student 5,859 PointsColton Ehrman
Courses Plus Student 5,859 PointsDoes that make sense?
ammarkhan
Front End Web Development Techdegree Student 21,661 Pointsammarkhan
Front End Web Development Techdegree Student 21,661 PointsBut where does Math.Random goes?
Colton Ehrman
Courses Plus Student 5,859 PointsColton Ehrman
Courses Plus Student 5,859 PointsWell in your code you alert Math.random, which probably won't do what you would expect because Math.random is a function and if you wanted to see the result you would have to call it using parenthesis like so Math.random() otherwise, without parenthesis, you are just passing the function to alert, not the returned value of the function
Tommy Gebru
30,164 PointsTommy Gebru
30,164 PointsHey Ammar, your original code has an extra parentheses or ")" and it called the Math.random function with no argument. Therefore it should not display anything. It has no value in this Code Challenge. However if you place the function max in alert, then max would be displayed because it passes the argument** 15 and 25 instead of placeholders **value1 and value2.
Hope this helps ;)