Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial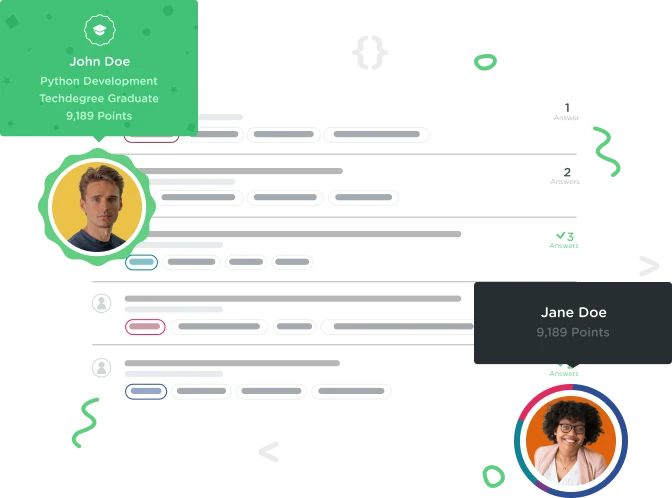

David Lindblad
941 Pointscorrect += does not seem to be working... I know I'm missing something obvious
/*
- Store correct answers
- When quiz begins, no answers are correct */ let correct = 0;
// 2. Store the rank of a player
let rank;
// 3. Select the <main> HTML element
/*
- Ask at least 5 questions
- Store each answer in a variable
- Keep track of the number of correct answers */ const q1 = prompt ('Number of fingers?'); if (q1 === 10){ correct += 1; } const q2 = prompt ('Number of toes?'); if (q2 === 10) { correct += 1; } const q3 = prompt ('Number of ears?'); if (q3 === 2) { correct += 1; }
const q4 = prompt ('Number of eyes?'); if (q4 === 2) { correct +=1; }
const q5 = prompt ('Number of mouths'); if (q5 === 1) { correct +=1; }
/*
- Rank player based on number of correct answers
- 5 correct = Gold
- 3-4 correct = Silver
- 1-2 correct = Bronze
- 0 correct = No crown */
if (correct >= 5){ rank = 'Gold'; } else if (correct >=3) { rank = 'Silver'; } else if (correct >=2) { rank = 'Bronze'; } else { rank = '"Giant Loser"'; }
// 6. Output results to the <main> element
alert (Thanks for playing, your ranking is ${rank}, you got ${correct} out of a possible 5
);

David Lindblad
941 PointsThat works thanks, what I don't understand is why that's necessary for numbers but not strings?
5 Answers

David Lindblad
941 PointsGood to know, thanks. With the strict comparison operator === in my code it was still not adding 1 to 'correct' even when I was using/inputting the expected number, that's what is confusing me... unless I'm still missing something? Sorry, I really am very new to all this.
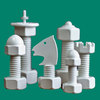
Steven Parker
231,153 PointsThat's right, with the strict comparison the answer never matched.
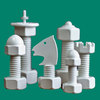
Steven Parker
231,153 PointsThe strict (type-sensitive) comparison operator "===" won't consider a string to match a number no matter what the displayed value is. It will compare numbers to numbers, or strings to strings, but not mixed.
There are three ways to handle it:
- convert the input into a number, as Raksmey suggested
- compare with a string instead of a number
if (q1 === "10") { correct += 1; }
- use the standard comparison operator
if (q1 == 10) { correct += 1; }

David Lindblad
941 PointsI was only wanting it to work if the number input was '10', guess I'm not yet far enough along in the course to understand why the + is required before the 'q1', 'q2' etc for a number but not a string. Thanks
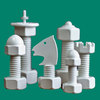
Steven Parker
231,153 PointsThe "+" in front of a string converts it to a number. And quotes around "10" makes it a string.
The standard "==" operator will do conversion for you.

David Lindblad
941 PointsNot sure what you mean by the answer never matched, if Iām expecting ... === 10 and entering 10 how does that not match?
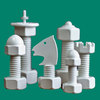
Steven Parker
231,153 PointsThe "prompt" function gives back a string, so "q1" had a string in it. When you compare it using "===" to the number 10, it doesn't match because one is a string and the other is a number.
But if you use "==" the system converts for you and they will match.
Glad I could help. You can mark a question solved by choosing a "best answer".
And happy coding!

David Lindblad
941 PointsAh, didn't realize the prompt only returned strings. Thanks Steven.
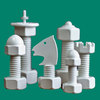
Steven Parker
231,153 PointsDavid Lindblad ā Glad to help. But normally, one would choose a "best answer" on the answer the contained the information that helped most to solve the issue!
(You can always change that choice )
Raksmey soriya
3,101 PointsRaksmey soriya
3,101 PointsYou forgot to convert a correct answer from the prompt. we can covert it by adding + before it or using parseInt();
And here is the answer:)
Store correct answers When quiz begins, no answers are correct */ let correct = 0; // 2. Store the rank of a player
let rank;
// 3. Select the <main> HTML element
/*
Ask at least 5 questions Store each answer in a variable Keep track of the number of correct answers */ const q1 = prompt ('Number of fingers?');if (+q1 === 10){ correct += 1;} const q2 = prompt ('Number of toes?'); if (+q2 === 10) { correct += 1; } const q3 = prompt ('Number of ears?'); if (+q3 === 2) { correct += 1; } const q4 = prompt ('Number of eyes?'); if (+q4 === 2) { correct +=1; } const q5 = prompt ('Number of mouths'); if (+q5 === 1) { correct +=1; }
/*
Rank player based on number of correct answers 5 correct = Gold 3-4 correct = Silver 1-2 correct = Bronze 0 correct = No crown */ if (correct >= 5){ rank = 'Gold'; } else if (correct >=3) { rank = 'Silver'; } else if (correct >=2) { rank = 'Bronze'; } else { rank = '"Giant Loser"'; }
// 6. Output results to the <main> element
alert (
Thanks for playing, your ranking is ${rank}, you got ${correct} out of a possible 5
);