Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial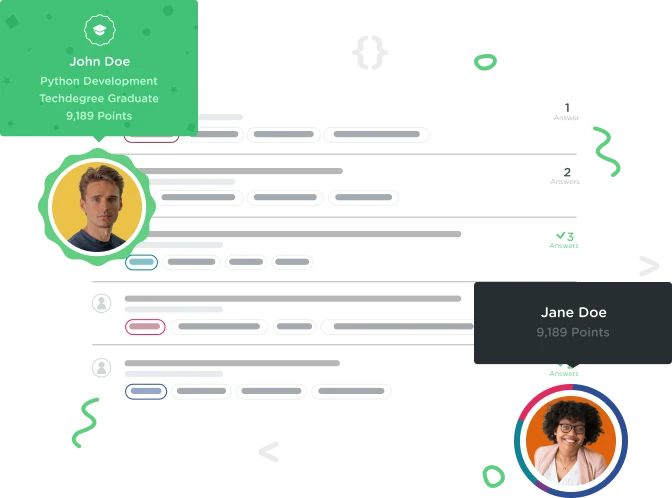
Kirby Abogaa
3,058 PointsCould I have done better?
I actually spent an awful lot of time figuring out this challenge. And I was wondering if there was anything I could have done better within the scope of this course.
Here is my code:
<script>
var questions = [
["An animal that says 'mooo'?", "cow"],
["What is the planet after earth?", "mars"],
["What is the planet at the end of our solar system?", "pluto"]
];
var ask;
var correctAns = 0;
var wrongAns = 0;
var correct;
var correctHtml = "<h2>You got these question(s) correct:</h2>";
var wrongHtml = "<h2>You got these question(s) wrong:</h2>";
function print(message) {
document.write(message);
}
function quizBee( list ) {
/*do not concatenate any <ol> or </ol> inside the loop because it will behave differently*/
correctHtml += '<ol>';
wrongHtml += '<ol>';
for ( var i=0; i<list.length; i++ ) {
ask = prompt(list[i][0]);
ask = ask.toLowerCase();
if(ask === list[i][1]) {
correctAns++;
correctHtml += "<li>" + list[i][0] + "</li>";
}else{
wrongAns++;
wrongHtml += "<li>" + list[i][0] + "</li>";
}
}//end for loop
correctHtml += '</ol>';
wrongHtml += '</ol>';
correct = "<p>You got " + correctAns + " correct answer(s).</p>"
print(correct);
if (correctAns > 0 && wrongAns > 0) {
print(correctHtml);
print(wrongHtml);
}else{
if(correctAns == 0) {
print(wrongHtml);
}else{
print(correctHtml);
}//end nested if
}//end if
}//end function
quizBee(questions);
</script>
3 Answers
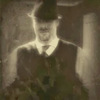
Joel Smith
14,779 PointsI went over your code and found no errors that I could see, aside from one simple mistake:
Pluto is no longer considered a planet in our solar system.
Great Job!! ;)
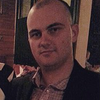
Dejan Buric
12,560 PointsExcellent code Kirby. I watched your code to finish my Build a quiz challenge 1. I cant build it alone, its really hard for me. I understand all lines of code, but i cant code it.
Good job mate!

Iain Simmons
Treehouse Moderator 32,305 PointsGreat job! I also used a function in this challenge, but my implementation was a little bit different:
var correct = 0;
var minResponseLength = 5;
var questions = [
["What is the largest living land animal?", "african elephant"],
["What is the largest living reptile?", "saltwater crocodile"],
["What is the largest living fish?", "whale shark"],
["What is the largest living bird?", "common ostrich"],
["What is the tallest living animal?", "giraffe"]
];
function print(message) {
document.write(message);
}
// a function to ask the question and return true if answered correctly
function quizQuestion(questions, questionNumber) {
// get the user's response to the question being asked, convert to lowercase
var response = prompt(questions[questionNumber][0]).toLowerCase();
/* return true if the user's response exists within
or is equal to the actual answer
AND the response is greater than or equal to the min characters
(so they can't cheat with a random letter or two)
- this allows for responses that aren't the full string in the answer */
return (
questions[questionNumber][1].indexOf(response) >= 0
&&
response.length >= minResponseLength
)
}
for (var i=0; i<questions.length; i++) {
if (quizQuestion(questions, i)) {
correct++;
}
}
print('<p>Out of ' + questions.length + ' questions, you got ' + correct + ' correct and ' + (questions.length - correct) + ' incorrect.</p>');