Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial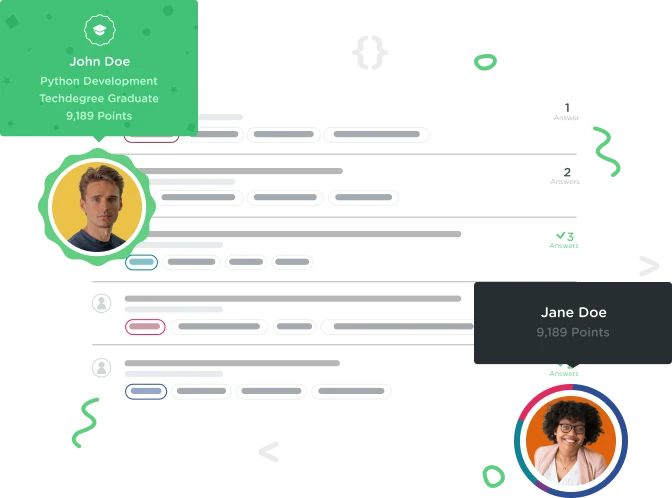

Dermot Condron
4,010 PointsCount function with array
In the quiz the answer to what does the following code displays is 6. Can someone explain how and why 6 is the value of $sum?
Thanks in advance.
<?php
$numbers = array(1,2,3,4);
$total = count($numbers);
$sum = 0;
$output = "";
$i = 0;
foreach($numbers as $number) {
$i = $i + 1;
if ($i < $total) {
$sum = $sum + $number;
}
}
echo $sum;
7 Answers

Robert Walker
17,146 PointsThe best way to explain this is to understand each part of the code.
So from top:
$numbers = array(1, 2, 3, 4);
$total = count($numbers);
What is happening here is we are counting how many values are in the array $numbers, so 4 numbers, each value separated with a , = 4 values so our $total variable would be equal to 4.
$numbers = array(1, 2, 3, 4);
$total = count($numbers); // $total = 4;
Ignore $output as its not needed in the explaining.
$sum = 0;
$i = 0;
Here we are simply giving sum and i the value of 0 but they become really important as we move tot he foreach loop.
foreach($numbers as $number) {
$i = $i + 1;
if ($i < $total) {
$sum = $sum + $number;
}
So lets break this down a little.
foreach($numbers as $number) {
$i = $i + 1;
}
So what this does is every time the loop runs we are adding 1 to the value of $i.
foreach($numbers as $number) {
$i = $i + 1;
if ($i < $total) {
}
Now we are saying, if $i is less than $total (Which remember is 4) then run the code that follows.
foreach($numbers as $number) {
$i = $i + 1;
if ($i < $total) {
$sum = $sum + $number;
}
Now if $i is less than 4 add $sum + number together and store it in the variable $sum.
So for the first loop:
$i becomes 1
$sum becomes 1 because $number on the first loop = 1 for the first value in our array.
So after this first loop:
$i = 1;
$sum = 1;
Time for the second loop:
$i = $i(1) + 1;
$sum = $sum(1) + $number so because this is the second loop $number is the second value in our array which is the number 2:
$sum(1) + $number(2) 1 + 2 = 3
So after the second loop:
$i = 2;
$sum = 3;
Time for the third loop:
$i = $i(2) + 1;
$sum = $sum(3) + $number so because this is the third loop $number is the third value in our array which is the number 3:
$sum(3) + $number(3)
3 + 3 = 6
So after the third loop:
$i = 3; $sum = 6;
Time for the fourth loop:
foreach($numbers as $number) {
$i = $i + 1;
if ($i < $total) {
$sum = $sum + $number;
}
Now because on the forth loop $i is = to 3 and at the start of our foreach loop we are adding 1 to $i when we get to our if statement $i is now equal to 4 meaning our if $i is less than $total(4) do this code but because $i is no longer less than 4 we stop the foreach loop and do NOT carry on with the code.
Leaving our $i = 4; and our $sum = 6;
In short our foreach loop is adding the values of our array together one at a time:
1 + 2 + 3 = 6.
Sorry for being so long winded but really wanted to try my best to explain this as once you crack this, foreach loops become really easy to understand no matter how many things are going on in them.
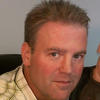
Dan Martin
33,150 Pointsinstead of $i < $total use $i <= $total.
Just less than only totals the numbers 1, 2, 3, on the fourth iteration $i is equal to the count.
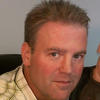
Dan Martin
33,150 PointsIn all honesty though, you don't even need to count. foreach handles that:
$numbers = array(1,2,3,4);
$sum = 0;
foreach($numbers as $number) {
$sum = $sum + $number;
}
echo $sum;
It is literally, for Each $numbers array, assign $number variable for each value.

Dermot Condron
4,010 PointsThanks for your answer Dan. I follow the logic but I am confused by the use of the 2 variables $sum and $i. Should it not be written $sum = $i + $number; rather than $sum = $sum + $number;
Also in the statement $sum = $sum + $number what are the values of the 2 variables $sum and $number to get 6 Is it $sum = 3 + 3 or is it $sum = 0 + 6?
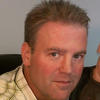
Dan Martin
33,150 Points$i is just the count... Oddly in this case it would almost match the array. The value of $i in the loop first time would be 0 then 1 then 2 then 3. $sum set at zero to start, then adds the $number in the $numbers array. Then adds the second value of the array "2", to the previous sum giving a total and updating the variable $sum to "3", repeating until all values of the array have been added together giving you "10"

Dermot Condron
4,010 PointsThanks Robert for your comprehensive answer. I now realise that my basic understanding of this was flawed. Your explanation has cleared that up! Thanks also Dan for your help!

Robert Walker
17,146 PointsNo problem glad it helped, was a little worried as im crap at explaining these types of things.