Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial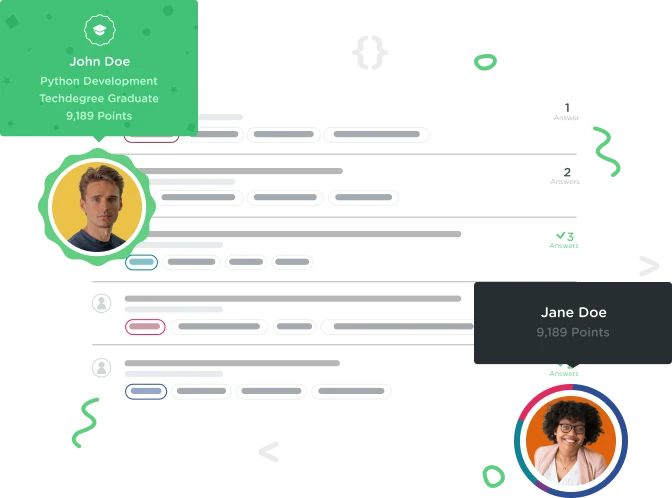

lakshmi Nandon Boruah
1,514 PointsCreate a new function named max() which accepts two numbers as arguments. The function should return the larger of the t
Create a new function named max() which accepts two numbers as arguments. The function should return the larger of the two numbers. You'll need to use a conditional statement to test the 2 numbers to see which is the larger of the two.
function max(20,10){
if(20>10){
return 20;}
}
13 Answers

Sean Henderson
15,413 PointsThe purpose of a function is that it can accept any value (like a number or a string) as an argument (the values inside parenthesis after the name) and perform operations on it.
On the first line, you are declaring a function called max
.
function max(20,10){
// ...
Inside of the parenthesis, there are 2 arguments. The problem is, these must be valid javascript identifiers (variables). They cannot start with a number (see http://www.w3schools.com/js/js_variables.asp).
The first thing to do is change the arguments you define into valid js variables, like this:
function max(a,b){
// ...
(It doesn't matter much what you name them, since they are only used inside of this function and not in the global scope. In more complex programs it is beneficial to give more descriptive names.)
Now that the function is ready to take in arguments (we'll do that last), we can create the logic inside of the function block using those arguments just like variables:
function max(a, b) {
if (a > b) {
return a;
}
}
Notice that we are using the same argument names that we defined in the first line, so the javascript interpreter knows we are referring to whatever was passed in to the function as an argument (not the literal letter "a").
Now this almost works, but what happens if b
is greater than a
? Nothing, so here we need to use more logic to cover all possible scenarios.
function max(a, b) {
if (a > b) {
return a;
} else {
return b;
}
}
Now, the function checks first if the first argument is greater, then returns (exits) if it is. If the first argument is not greater, then for the purposes of this exercise we can assume that the second argument is greater, so we return b
.
When you input this code into Team Treehouse's quiz box, they will test your code by calling the max()
function with different arguments and checking the answers, like this:
max(20,10) // => 20, CORRECT
max(10,20) // => 20, CORRECT
max(0,100) // => 100, CORRECT
max(45,-45) // => 45, CORRECT

James ...
5,131 Pointsvar a = 15; var b = 25;
function max(a, b) { if (a > b) { return a; } else { return b; } }
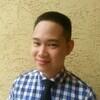
Tom Nguyen
33,502 Pointssolution I used:
function max(a, b){
if (a>b){
return a;
}else if (a==b){
return a;
}else{
return b;
};
};
console.log(max(2,3));

jboyegagzs
5,622 Pointsnoice!

Colton Schmucker
1,335 PointsGreat explanation, thank you!

Gertrude Dawson
5,371 PointsI haven't got the answer. I am confused by the bracketing and when to enter my variables. I keep checking other websites for solutions and they give me various other examples but they don't help me solve this problem. It would be great if I could have my previous Workspaces open while I was solving this problem or if I could cut in paste on my previous solved functions to a file that I could use while solving these problems.

Starky Paulino
Front End Web Development Techdegree Student 6,398 Pointsvar a = 15; var b = 25;
function max(a, b) { if (a > b) { return a; } else { return b; } }
it's the answer.
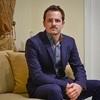
Nikos Papapetrou
6,305 PointsCould be this an another way to solve and to find the max number?
function myFunction( num1, num2 ) {
return Math.max( num1, num2 );
}
document.write( myFunction( 18, 9 ) );

CSL Team
Courses Plus Student 9,943 Pointsyes Nikos but the function needs to be called max.
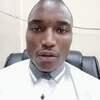
Blessed Marufu
3,927 Pointsthanks very helpful with clear steps

Samrawit Tsegay
4,367 Pointsconst par1 = 3; const par2 = 5; function max(par1,par2){ if(par1<par2){ return par2; } }

Elfar Oliver
3,924 Pointsfunction max(blue, red) { if (blue < red) { return red; } else { return blue; } }
let blue = 20; let red = 30;
Needing a conditional statement kinda made it easier imo
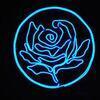
Ashton Little
7,352 Pointsfunction max(a, b){
if (a > b){
return a;
} else {
return b;
}
}

Tashae Love
325 PointsFigured it out!
function max (a, b) { if (a > b) { return a; } else { return b; } }
Learning coding
Front End Web Development Techdegree Student 9,937 PointsLearning coding
Front End Web Development Techdegree Student 9,937 PointsThanks, this answer helped me. It's well broken down ito pieces. Great to read and understand all of it!
Max Botez
5,146 PointsMax Botez
5,146 Pointsit's just perfect explanation!
B G
1,866 PointsB G
1,866 PointsGreat explanation. Thank you.
Michael Rooze
6,636 PointsMichael Rooze
6,636 Pointswhy do you say in the first line, it can accept any value including a number and then a few lines later say that it cant be a number?
David Jordan
2,365 PointsDavid Jordan
2,365 PointsAy, Ik it's been years since you made this comment on here, but this explanation may have just single handedly helped me to understand functions on a fundamental level and I've been only barely getting it piece by piece for a month now. Thank you so much for the information.
Sterling Gordon
5,376 PointsSterling Gordon
5,376 PointsThanks for the breakdown!