Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial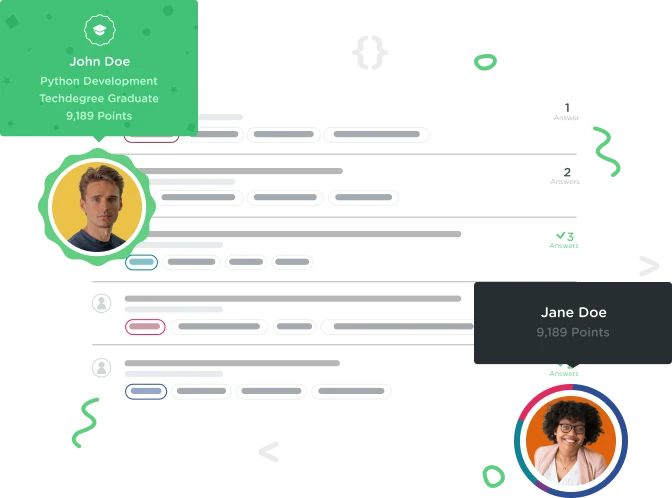

Sophie Rae
3,066 PointsCreate a String for values within a given Range from a Hash
Hi! Would be great if someone could give me a hand with solving this problem. I am not sure how to start...Thanks in advance!
Instructions:
Complete the solution so that it returns a String in the format:
"Key (Value), Key (Value)"
From a hash for values within the given range, sorted by value lowest to highest.
For example:
food = {
"Hamburger"=>1.99,
"Cheese"=>0.99,
"Steak"=>4.99,
"Lobster"=>7.99,
"Fries"=>1.49,
"Sandwich"=>2.49
}
list_in_range(food, (0.99..1.99)) # should return "Cheese (0.99), Fries (1.49), Hamburger (1.99)"
Your solution:
def list_in_range(hash, range)
end
1 Answer
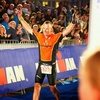
Steve Hunter
57,712 PointsHi Sophie,
Here's my attempt at that.
I'm sure there are far more elegant ways of manipulating hashes than this, though.
I set up the defined range, an empty hash and an empty string. Then, I've iterated through the food hash, pulling out the key and value (k
and v
) for each pair. Then, using the cover?
method on the defined range, I pulled out the pairs where the value was within the range. This pair gets added to the empty hash. Then I reorder the hash and loop through it to create an interpolated string to look like your output.
I hope that's what you need.
Steve.
food = {
"Hamburger" => 1.99,
"Cheese" => 0.99,
"Steak" => 4.99,
"Lobster" => 7.99,
"Fries" => 1.49,
"Sandwich" => 2.49
}
price_range = (0.99..1.99)
def list_in_range(food, price_range)
out_hash = {}
out_string = ""
food.each do |k, v|
if price_range.cover?(v)
out_hash.store(k, v)
end
end
out_hash = out_hash.sort_by { | _key, value | value }
out_hash.each do |k, v|
out_string += "#{k} #{v}, "
end
puts out_string
end
# call the method
list_in_range(food, price_range)
Sophie Rae
3,066 PointsSophie Rae
3,066 PointsHi Steve!
Thanks very much for this. Can I ask what the cover? method does?
Sophie
Steve Hunter
57,712 PointsSteve Hunter
57,712 PointsHi Sophie,
The
cover?
method works on aRange
object and returns true or false if the parameter passed in is within the range. Documentation is here.I iterated over the
food
hash with aneach
loop. I pulled out the key and value with each iteration; I called themk
andv
. To determine if the hash item needed to be in the output hash, i.e. the item was within theprice_range
, I passed the valuev
intocover?
. If I get true back, I added the key and value intoout_hash
using itsstore
methodSteve. .