Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial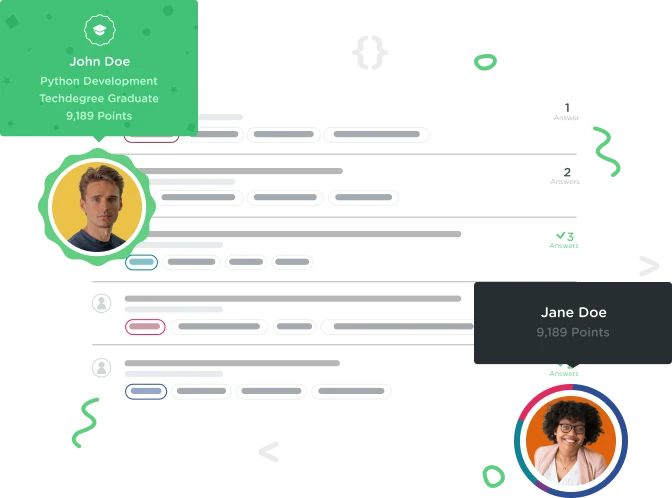

John Cieraszynski
1,580 PointsCreate Max function ?
Challenge Task 1 of 2
Create a new function named max which accepts two numbers as arguments (you can name the arguments, whatever you would like). The function should return the larger of the two numbers.
HINT: You'll need to use a conditional statement to test the 2 parameters to see which is the larger of the two.
I forgot how to write a conditional statement. I think I am overthinking this....any advice?
My code looks something like
function max (big, small) { var biggest = ??????? return biggest
} max (10, 5);
function max (big, small) {
var biggest = big;
return biggest;
}
max (10, 5);
2 Answers

Maximillian Fox
Courses Plus Student 9,236 PointsA conditional statement would look like
if(aCondition){
// do something
} else {
// do something else
}
So you could make a function that takes 2 numbers, let's call them firstNumber and secondNumber. Then, use an operator such as 'less than' or 'greater than' to compare them in a conditional like this...
if(firstNumber < secondNumber){
// give out the secondNumber
} else {
// give out the firstNumber
}

John Cieraszynski
1,580 PointsHello Max, Thanks much. As I thought I was beginning to overthink the solution way too much. I had thoughts of boolean variables going through my head along with var assignments. All I needed was the below...and to watch my speling... :)
function max(number1, number2) { if (number1 > number2) { return number1 } else { return number2 } } max (10, 5);

Maximillian Fox
Courses Plus Student 9,236 PointsSpot on :) Happy coding!
Raymon Oleaga
19,298 PointsRaymon Oleaga
19,298 Pointsfunction max(a, b) {