Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial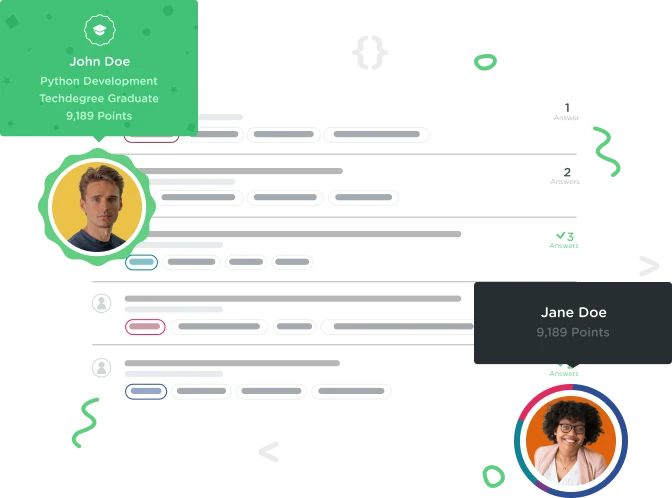
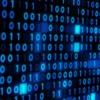
Alexander Davison
65,469 PointsCreating a Chatroom project in C# — But what's wrong in my code?
Hi guys, I just finished the C# Objects course, and I wanted to make a Chatroom application. (Yes, it is text-based and not over the Internet.)
I've already made a pretty decent one with Python. I wanted to try to do it as well with C#, because I felt that I learned enough from the course to be able to.
By the way, I'm fairly new to C#, so sorry if the error is obvious
Here's my User class:
using System;
namespace Chatroom
{
class User
{
public string Username { get; }
public string FullName { get; set; }
public string Email { get; set; }
public string Bio { get; set; }
private readonly string _password;
private const string line = "-" * 30;
public static void User(string username, string fullName,
string email, string password)
{
Username = username;
FullName = fullName;
Email = email;
_password = password;
}
public void DisplayUser()
{
Console.WriteLine(line);
Console.WriteLine(Username);
Console.WriteLine(line);
Console.WriteLine("Full name: " + FullName);
Console.WriteLine("Email: <" + Email + ">");
Console.WriteLine(line);
Console.WriteLine(Bio);
Console.WriteLine(line);
}
}
}
My console output:
treehouse:~/workspace$ mcs -out:Chatroom.exe *.cs && mono Chatroom.exe
Chatroom.cs(10,34): error CS1525: Unexpected symbol `alexdavison888@gmail.com'
Chatroom.cs(10,63): error CS1525: Unexpected symbol `GPIOpython__'
User.cs(16,28): error CS0542: `Chatroom.User.User(string, string, string, string)': member names cannot be the same as their enclosing type
User.cs(5,11): (Location of the symbol related to previous error)
Compilation failed: 3 error(s), 0 warnings
I can't seem to see any kind of error here. Can somebody give me a hand? Thank you
~Alex
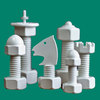
Steven Parker
231,126 Points
I got your message.
But it looks like Mikis already has you covered. I would just add that the reason you can't use the class name as a method name is because it would appear to be a constructor.
But one error message you got is most curious: "Chatroom.cs(10,63): error CS1525: Unexpected symbol 'GPIOpython_'
" — Where the heck does that come from?
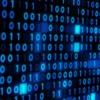
Alexander Davison
65,469 PointsI posted the Chatroom.cs file's code below, just FYI.
1 Answer
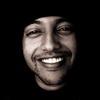
miikis
44,957 PointsHi Alexander,
I'm not too familiar with C# but — from the error message — it seems like you can't have static methods that have the same name as their class; that is, User.User is illegal.
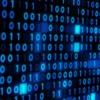
Alexander Davison
65,469 PointsThank you! it fixed one of the errors.
However, after fixing that, I still get this error:
treehouse:~/workspace$ mcs -out:Chatroom.exe *.cs && mono Chatroom.exe
Chatroom.cs(10,34): error CS1525: Unexpected symbol `alexdavison888@gmail.com'
Chatroom.cs(10,63): error CS1525: Unexpected symbol `GPIOpython__'
Compilation failed: 2 error(s), 0 warnings
Also, just in case you want to see, this is my Chatroom
class:
namespace Chatroom
{
class Chatroom
{
static void Main()
{
User user = new User("xela888", "Alexander Davison"
"alex@gmail.com", "password");
}
}
}
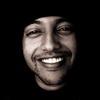
miikis
44,957 PointsYou're missing a comma after "Alexander Davison" when you initialize User in Main().
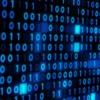
Alexander Davison
65,469 PointsThank you :D
Alexander Davison
65,469 PointsAlexander Davison
65,469 PointsTagging Steven Parker