Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial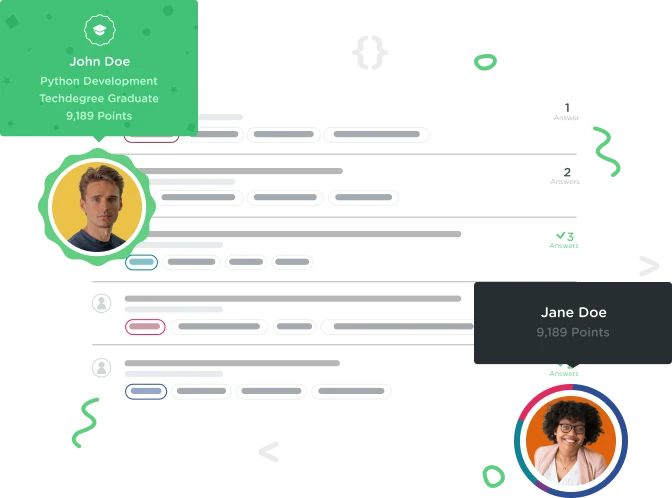
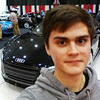
Joel Buzzanco
Courses Plus Student 2,738 Pointscreating a max function challenge
I do not understand what is wrong with my code. It is attached.
This section of the course in JS Basics has been a little confusing to me.
Please help, Thank you
function max () {
var number1 = 1;
var number2 = 2;
if (number2 > number 1) {
return true
}
}
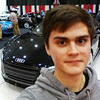
Joel Buzzanco
Courses Plus Student 2,738 Pointsfunction max () { var number1 = 1; var number2 = 2; if (number2 > number 1) { return true } }
4 Answers

Ken Alger
Treehouse TeacherJoel;
Your max()
function isn't accepting any arguments. We need to do something like:
function max(number1, number2) {
// do something magical in here
}
Make any sense?
Happy coding,
Ken
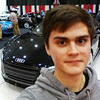
Joel Buzzanco
Courses Plus Student 2,738 PointsOK, thanks everyone for your help so far.
Hit a wall... again.
I don't know what is wrong with my code. Here is what I did:
function max (number1, number2) {
var number1 = 1;
var number2 = 2;
if (number2 > number1) {
return number2;
}
}

Ken Alger
Treehouse TeacherJoel;
Your function declaration looks spot on. Nice work! Now let's take a look inside the function. We don't want to assign values to our function parameters, we want to use those numbers. So if we do something like:
function max (number1, number2) {
if (number1 > number2) {
return number1;
} else if (number2 > number1) {
return number2;
}
}
We are doing what we need to accomplish. Does that make any sense? So, if we were to call max(5, 7)
we would get 7
back from our function.
Inside the function then, it would check to see if 5 > 7
, which it isn't so then it would go to the else if
and do that comparison. Since that (7 > 5
) does resolve as True
our function would return, in this case, 7
.
Post back if you are still stuck on the concept here.
Happy coding,
Ken
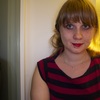
Karolin Rafalski
11,368 PointsA few issues with your code.
- you have declared your arguments (the parameters for your function) inside your function -->move your var number1 & var number 2 outside of your function.
- it is asking that you return the larger number - not a true or false. --> in your if statement change to return number2
- in your current if statement you have a typo - it should by number1 not number 1
I hope this helps!
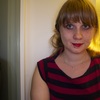
Karolin Rafalski
11,368 Pointsone more thing, you'll need to pass your variables number1 and number2 into your function. you can do so by putting them in the parenthesis after your function and separate them with a comma.
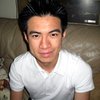
jason chan
31,009 PointsHey Joel,
The whole point of programming is not to repeat yourself. So basically create a function that can be called later on. So always have that mindset.
DRY my friend. DRY.

Inge L
30,058 PointsJoel, delete variables from your code (because you are creating function you will later call with any two numbers) and provide else to if statement. So you need to return
if (number2 > number1) {
return number2;
} else {
return number1;
}
Caleb Kleveter
Treehouse Moderator 37,862 PointsCaleb Kleveter
Treehouse Moderator 37,862 PointsI am not seeing the code.