Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial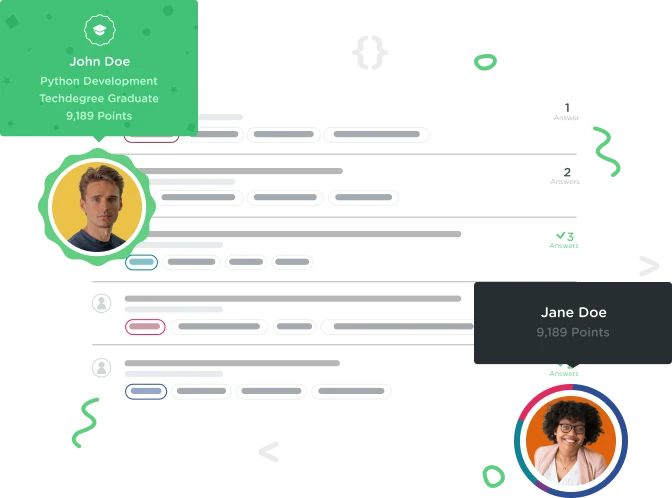

anomalousc0d3r
1,432 PointsDatabase creating duplicate entries in MySQL and PHP.
My database is creating duplicate entries for some reason while running this code in the table "steam users" and column "steamid". I would appreciate some help. Thanks!
<?php
include("settings.php");
if (empty($_SESSION['steam_uptodate']) or $_SESSION['steam_uptodate'] == false or empty($_SESSION['steam_personaname'])) {
$url = file_get_contents("http://api.steampowered.com/ISteamUser/GetPlayerSummaries/v0002/?key=".$steamauth['apikey']."&steamids=".$_SESSION['steamid']);
$content = json_decode($url, true);
$_SESSION['steam_steamid'] = $content['response']['players'][0]['steamid'];
$_SESSION['steam_communityvisibilitystate'] = $content['response']['players'][0]['communityvisibilitystate'];
$_SESSION['steam_profilestate'] = $content['response']['players'][0]['profilestate'];
$_SESSION['steam_personaname'] = $content['response']['players'][0]['personaname'];
$_SESSION['steam_lastlogoff'] = $content['response']['players'][0]['lastlogoff'];
$_SESSION['steam_profileurl'] = $content['response']['players'][0]['profileurl'];
$_SESSION['steam_avatar'] = $content['response']['players'][0]['avatar'];
$_SESSION['steam_avatarmedium'] = $content['response']['players'][0]['avatarmedium'];
$_SESSION['steam_avatarfull'] = $content['response']['players'][0]['avatarfull'];
$_SESSION['steam_personastate'] = $content['response']['players'][0]['personastate'];
$_SESSION['steam_realname'] = $content['response']['players'][0]['realname'];
$_SESSION['steam_primaryclanid'] = $content['response']['players'][0]['primaryclanid'];
$_SESSION['steam_timecreated'] = $content['response']['players'][0]['timecreated'];
$_SESSION['steam_uptodate'] = true;
}
$steamprofile['steamid'] = $_SESSION['steam_steamid'];
$steamprofile['communityvisibilitystate'] = $_SESSION['steam_communityvisibilitystate'];
$steamprofile['profilestate'] = $_SESSION['steam_profilestate'];
$steamprofile['personaname'] = $_SESSION['steam_personaname'];
$steamprofile['lastlogoff'] = $_SESSION['steam_lastlogoff'];
$steamprofile['profileurl'] = $_SESSION['steam_profileurl'];
$steamprofile['avatar'] = $_SESSION['steam_avatar'];
$steamprofile['avatarmedium'] = $_SESSION['steam_avatarmedium'];
$steamprofile['avatarfull'] = $_SESSION['steam_avatarfull'];
$steamprofile['personastate'] = $_SESSION['steam_personastate'];
$steamprofile['realname'] = $_SESSION['steam_realname'];
$steamprofile['primaryclanid'] = $_SESSION['steam_primaryclanid'];
$steamprofile['timecreated'] = $_SESSION['steam_timecreated'];
DEFINE('DB_USER', 'root');
DEFINE('DB_PASSWORD', '');
DEFINE('DB_HOST','localhost');
DEFINE('DB_NAME','codeforage');
$con = new PDO( 'mysql:host='.DB_HOST.';dbname='.DB_NAME.';charset=utf8',DB_USER,DB_PASSWORD );
$con->setAttribute(PDO::ATTR_ERRMODE, PDO::ERRMODE_EXCEPTION);
try {
$query = $con->prepare( "SELECT steamid FROM steam_users WHERE steamid = ?" );
$query->bindValue( 1, $steamprofile['steamid'], PDO::PARAM_STR );
$query->execute();
} catch (Exception $e) {
echo "oh noes!! there's an error...";
}
if( $query->rowCount() > 0 ) { # If rows are found for query
echo "Email found!";
}
else {
echo "Email not found!";
$statement = $con->prepare("INSERT INTO steam_users(steamid) VALUES(' " . $steamprofile['steamid'] . " ')");
$statement->execute();
}
?>
2 Answers
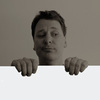
Sean T. Unwin
28,690 PointsIs it duplicating during the "Email not found" echo? If so, is that echo statement being output more than once?
I didn't find any errors (though I may have missed somehting), however I'm wondering if this file/script is able to be ran twice or consecutively somehow from a parent file?

anomalousc0d3r
1,432 PointsThanks for the help, but I have fixed the problem by installing a temporary/permanent solution into the MySQL database by adding the command that doesn't allow duplicates.