Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial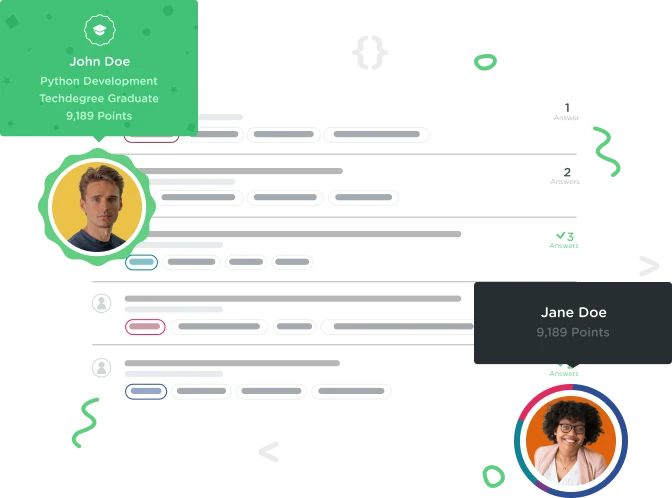

dlpuxdzztg
8,243 PointsDataSource Unable to Create Item After Insterting 'For' Loop in 'create()' Method
What I'm trying to accomplish
I'm saving an array of objects (ListItem) that have their own ArrayList parameter of custom objects (TaskItem). The approach I'm taking to saving both is by looping through the list of ListItem objects and retrieving each object's ArrayList parameter. I'm doing this in my DataSource:
public void create(ListItem item) {
SQLiteDatabase database = open();
database.beginTransaction();
ContentValues contentValues = new ContentValues();
contentValues.put(EasyListSQLiteHelper.LISTS_NAME, item.getListName());
contentValues.put(EasyListSQLiteHelper.LISTS_CHECKED, item.getImportant());
long listId = database.insert(EasyListSQLiteHelper.LISTS_TABLE, null, contentValues);
for (TaskItem taskItem : item.getListsList()) {
ContentValues taskValues = new ContentValues();
taskValues.put(EasyListSQLiteHelper.TASK_NAME, taskItem.getTaskName());
taskValues.put(EasyListSQLiteHelper.TASK_CHECKED, taskItem.getChecked());
taskValues.put(EasyListSQLiteHelper.FOREIGN_KEY_LIST, listId);
database.insert(EasyListSQLiteHelper.TASKS_TABLE, null, taskValues);
}
database.setTransactionSuccessful();
database.endTransaction();
close(database);
}
Then I create an 'addTask' function and call it in 'read' function:
public void addTask(ArrayList<ListItem> listItems) {
SQLiteDatabase database = open();
for (ListItem item : listItems) {
ArrayList<TaskItem> taskItems = new ArrayList<>();
Cursor cursor = database.rawQuery(
"SELECT * FROM " + EasyListSQLiteHelper.TASKS_TABLE + " WHERE LIST_ID = " + item.getId(),
null);
if (cursor.moveToFirst()) {
do {
TaskItem taskItem = new TaskItem(getIntFromColumnName(cursor, BaseColumns._ID),
getStringFromColumnName(cursor, EasyListSQLiteHelper.TASK_NAME),
getIntFromColumnName(cursor, EasyListSQLiteHelper.TASK_CHECKED));
taskItems.add(taskItem);
} while(cursor.moveToNext());
}
item.setListsList(taskItems);
cursor.close();
}
database.close();
}
read():
public ArrayList<ListItem> read() {
ArrayList<ListItem> listItems = readList();
addTask(listItems);
return listItems;
}
What I'm dealing with:
When I add an item to my array of ListItems, I get this error:
04-21 12:04:45.729 13316-13316/com.example.x.x E/AndroidRuntime: FATAL EXCEPTION: main
Process: com.example.x.x, PID: 13316
java.lang.NullPointerException: Attempt to invoke virtual method 'java.util.Iterator java.util.ArrayList.iterator()' on a null object reference
at com.example.x.x.EasyListDataSource.create(EasyListDataSource.java:134)
at com.example.x.x.Activitys.ListsActivity.saveList(ListsActivity.java:310)
at com.example.x.x.Activitys.ListsActivity.createListClickListener(ListsActivity.java:205)
at com.example.x.x.Fragments.NewListDialogFragment$1.onClick(NewListDialogFragment.java:92)
at android.view.View.performClick(View.java:5217)
at android.view.View$PerformClick.run(View.java:21349)
at android.os.Handler.handleCallback(Handler.java:739)
at android.os.Handler.dispatchMessage(Handler.java:95)
at android.os.Looper.loop(Looper.java:148)
at android.app.ActivityThread.main(ActivityThread.java:5585)
at java.lang.reflect.Method.invoke(Native Method)
at com.android.internal.os.ZygoteInit$MethodAndArgsCaller.run(ZygoteInit.java:730)
at com.android.internal.os.ZygoteInit.main(ZygoteInit.java:620)
And I think I know whats going on: When I create a ListItem object, the object does get created. But the 'for' loop won't work because the ListItem's ArrayList parameter is not populated. With that being said, the 'for' loop wont work. So, how should I fix this?