Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial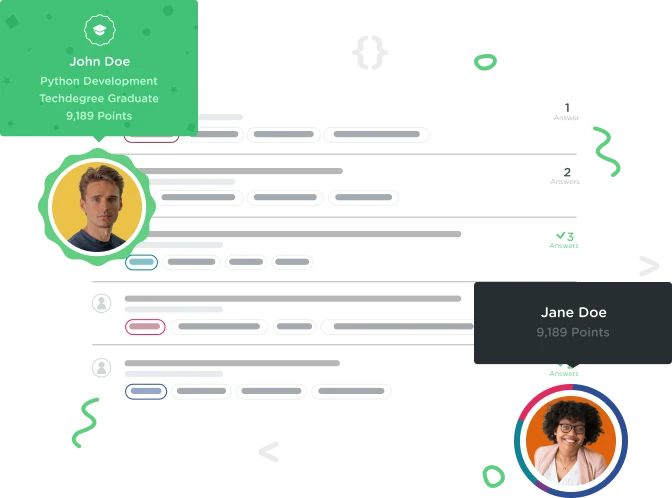
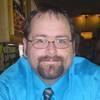
Jerome Allen
42,838 PointsDateTime.TryParse is failing about half the time
I'm working my way back through the C# Streams and Data Processing course. Everything is working to this point except, when the program reads the CSV soccer stats, about half of the DateTimes fails and get added to the array as a zero. I've re-downloaded the CSV file and compared my code to the project code. But I'm clearly missing something. Any ideas? Thanks, JNA
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using System.IO;
namespace SoccerStats
{
class Program
{
static void Main(string[] args)
{
string currentDirectory = Directory.GetCurrentDirectory();
DirectoryInfo directory = new DirectoryInfo(currentDirectory);
var fileName = Path.Combine(directory.FullName, "SoccerGameResults.csv");
var fileContents = ReadSoccerResult(fileName);
}
public static string ReadFile(string fileName)
{
using (var reader = new StreamReader(fileName))
{
return reader.ReadToEnd();
}
}
public static List<GameResult> ReadSoccerResult(string fileName)
{
var soccerResults = new List<GameResult>();
using (var reader = new StreamReader(fileName))
{
string line = "";
reader.ReadLine();
while((line = reader.ReadLine()) != null)
{
var gameResult = new GameResult();
string[] values = line.Split(',');
DateTime gameDate;
if (DateTime.TryParse(values[0], out gameDate))
{
gameResult.GameDate = gameDate;
}
gameResult.TeamName = values[1];
HomeOrAway homeOrAway;
if(Enum.TryParse(values[2], out homeOrAway))
{
gameResult.HomeOrAway = homeOrAway;
}
soccerResults.Add(gameResult);
}
}
return soccerResults;
}
}
}
1 Answer

Christopher Coscina
5,611 PointsThe code is working fine for me... Are one of your break points on or before the line of code in which the DateTime gets assigned to GameResult.gameDate? If you have a breakpoint on that particular line, then GameResult.gameDate shouldn't actually be set yet but gameDate (the local variable declared right before the if block that assigns the value of gameDate to gameResult.GameDate). should be set on that line. If you have a breakpoint on the next line, both gameDate and GameResult.gameDate should be properly set.
Jerome Allen
42,838 PointsJerome Allen
42,838 PointsIt turned out to be my Windows date format. Once I change it back to standard North American dd/mm/yyyy it began working.