Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial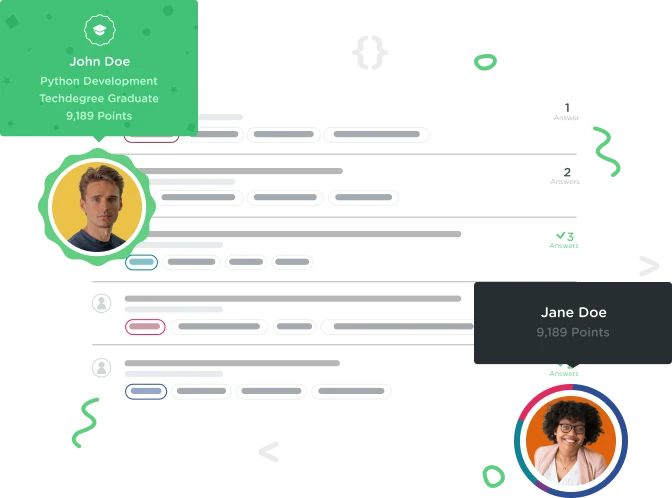
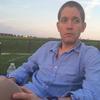
Drew Butcher
33,160 PointsDefault values not appearing
Using this code if i echo $p->getInfo(); all the variable (i.e. properties) are blank. Why do the default values not show up?
3 Answers
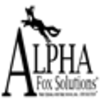
Shawn Gregory
Courses Plus Student 40,672 PointsHello,
Did you instantiate the class? That is how you add the values to the member variables of the class. There are no default values to start with until you instantiate the class and pass the values in through the constructor. Hope this helped you.
Cheers!
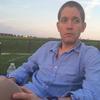
Drew Butcher
33,160 PointsHey Shawn Gregory it looks like I did at the end of the code where it says: "$p = new Product();" but still nothing appears!
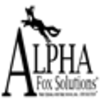
Shawn Gregory
Courses Plus Student 40,672 PointsDrew,
Your problem stems from not providing arguments to your product instantiation. While specifying arguments to a constructor of a class is optional, if you don't specify an argument, PHP will put a NULL value in its place. Your default values are being overwritten by those NULL values and therefor are not displaying anything. Add the arguments and it will display the values you specified. If you just add the name argument and nothing else you'll see that when the page is displayed, only the name will appear.
Default values for member variables are used when you may or may not replace it with anything during run-time and if you do, you will most likely add a conditional statement that either changes it to the new value or leave the default value. If you want it to display the default value if you supply no argument to the instantiation, use the following modifications:
function __construct($name, $price, $description){
if(isset($name)) $this->name = $name;
if(isset($price)) $this->price = $price;
if(isset($description)) $this->description = $description;
}
This way if you supply no arguments or only one or two, the default values you had in the class will display. Try it out and tell me the results.
Cheers!
Drew Butcher
33,160 PointsDrew Butcher
33,160 PointsI don't know what that means. I just followed the code given in the video and I wanted to see what $p-getInfo(); would produce and I was surprised that it $name did not have the value of 'default_name'.... If you want to see my code here it is: