Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial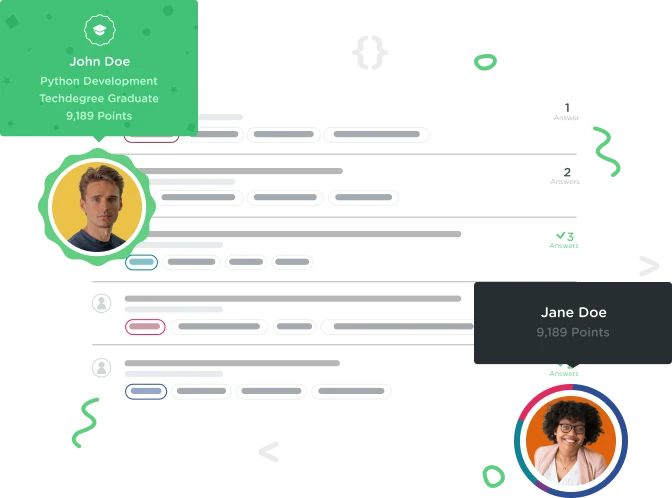

Matt Bloomer
10,608 Pointsdefaulting parameters
What am I doing wrong here?
public class Example {
public static void main(String[] args) {
ShoppingCart cart = new ShoppingCart();
Product pez = new Product("Cherry PEZ refill (12 pieces)");
cart.addItem(pez, 5);
/* Since a quantity of 1 is such a common argument when adding a product to the cart,
* your fellow developers have asked you to make the following code work, as well as keeping
* the ability to add a product and a quantity.
*/
Product dispenser = new Product("Yoda PEZ dispenser");
/* Uncomment the line following this comment,
after adding a new method using method signatures,
to solve their request in ShoppingCart.java
*/
cart.addItem(dispenser);
}
}
public class ShoppingCart {
public void addItem(Product item, int quantity) {
System.out.printf("Adding %d of %s to the cart.%n", quantity, item.getName());
/* Other code omitted for clarity. Please imagine
lots and lots of code here. Don't repeat it.
*/
public void addItem(Product item){
addItem(item+=1); }
}
}
}
public class Product {
/* Other code omitted for clarity, but you could imagine
it would store price, options like size and color
*/
private String mName;
public Product(String name) {
mName = name;
}
public String getName() {
return mName;
}
}
1 Answer
Christopher Augg
21,223 PointsHello Matt,
Your issue is within your overloaded addItem method when you call addItem.
public void addItem(Product item){
addItem(item+=1); }
}
item is an instance of the Product class. Therefore, we do not want to use item+=1 here. This code is correct otherwise and the only change you need to make is to place the correct arguments within the parenthesis where item+=1 is at the moment.
The idea is to call the original addItem method and pass the two arguments that it requires. One of them is the item being passed into your new overloaded method. The other is the amount. Of course, we are not passing in an amount to our new overloaded method. The reason is because we want to explicitly pass in 1 (i.e. item, 1). This allows us to call the overloaded method anytime we only want 1 item and only have to pass it the Product.
Hope this helps you resolve it. However, let me know and I can go over the code in more detail.
Regards,
Chris