Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial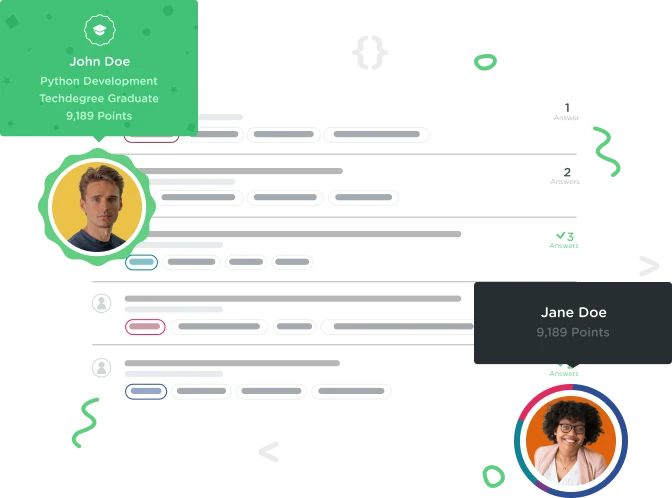

yannverreault
12,599 PointsDifficulty to use mysql db with slim/twig accross different pages.
I have an hotel website that has a database of two tables.
One table is the hotel and its informations like name, description, image.
Second table is the room information like name, size, description, image. Each room is linked to the hotel ID.
The problem I am facing, on the main page(/), I did a query for the hotels information(Main page is simply a dropdown with all the hotels listed. When you select an hotel, it goes to the hotels page) but once I reach the hotels page(all the information about the hotel and the rooms it contains), I have all the info about rooms, but no info about the hotel itself.
I think the problem is because that on my hotels page, I don't have a query for hotels information but I don't know how to do it.
Is there anybody that can help me with that?
<?php
use \Psr\Http\Message\ServerRequestInterface as Request;
use \Psr\Http\Message\ResponseInterface as Response;
require 'vendor/autoload.php';
$app = new \Slim\App();
$container = $app->getContainer();
// Register component on container
$container['view'] = function ($container) {
$view = new \Slim\Views\Twig('templates', [
'cache' => false
]);
$view->addExtension(new \Slim\Views\TwigExtension(
$container['router'],
$container['request']->getUri()
));
return $view;
};
// Render Twig template in route
$app->get('/', function ($request, $response, $args) {
require 'inc/database.php';
try {
$results = $db->prepare('SELECT * FROM hotels WHERE id = ?');
$results->bindParam(1, $id);
$results->execute();
} catch(Exception $e) {
echo $e->getMessage();
die();
}
$ids = $results->FetchAll(PDO::FETCH_ASSOC);
$i = array();
foreach($ids as $id){
$i = $id;
}
try{
$result = $db->query('SELECT * FROM hotels ORDER BY REPLACE(name, "The ", "")');
}catch(Exception $e){
echo $e->getMessage();
die();
}
$hotels = $result->FetchAll(PDO::FETCH_ASSOC);
$items = array();
foreach ($hotels as $hotel){
$items[] = $hotel;
}
return $this->view->render($response, 'home.twig', array('i' => $i,'items' => $items));
});
$app->get('/about', function ($request, $response, $args) {
return $this->view->render($response, 'about.twig'
);
});
$app->get('/contact', function ($request, $response, $args) {
return $this->view->render($response, 'contact.twig'
);
});
$app->get('/upload', function ($request, $response, $args) {
return $this->view->render($response, 'upload.twig'
);
});
$app->get('/hotels/{id}', function ($request, $response, $args) {
require 'inc/database.php';
$id = $args['id'];
try {
$roominfo = $db->prepare('SELECT hotels.name, rooms.*
FROM hotels
INNER JOIN rooms
ON rooms.hotel_id = hotels.id WHERE rooms.hotel_id = ?');
$roominfo->bindParam(1, $id);
$roominfo->execute();
} catch(Exception $e) {
echo $e->getMessage();
die();
};
$rooms = $roominfo->fetchAll(PDO::FETCH_ASSOC);
$r = array();
foreach($rooms as $room){
$r[] = $room;
}
return $this->view->render($response, 'hotels.twig', array('r' => $r));
});
$app->run();