Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial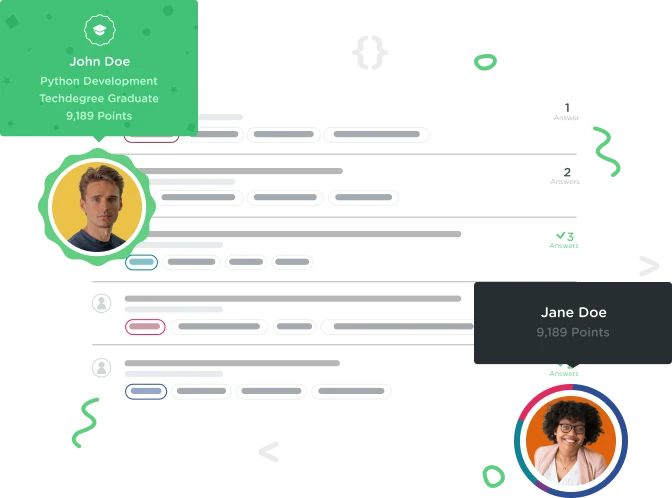
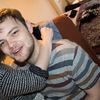
Marc Murray
4,618 PointsDisplaying a Bootstrap Modal after PHP for submission
Hi there guys, this issue has been giving me trouble for the past couple of days and I would really appreciate some clarification/direction. I have a PHP form working fine, but afterwards am looking to display a modal window. I have tried a couple of approaches, from echoing the script out to escaping PHP and running it from there, and have had success making other basic tests scripts work (eg alerts, echoing out words and console logs) so I know the event is triggering, I just can't get the desired functionality to happen. Would anyone be able to help me out here?
Relavent Page: http://marcmurray.net/test_sites/cans/news.php
<?php
if(isset($_POST["submit"])){
// Checking For Blank Fields..
if($_POST["vname"]==""||$_POST["vemail"]==""||$_POST["sub"]==""||$_POST["msg"]==""){
echo "Please fill out everything! We need to know who you are, and why you want to get in touch with us!";
} else {
// Check if the "Sender's Email" input field is filled out
$email=$_POST['vemail'];
// Sanitize E-mail Address
$email =filter_var($email, FILTER_SANITIZE_EMAIL);
// Validate E-mail Address
$email= filter_var($email, FILTER_VALIDATE_EMAIL);
$emailConfirmed=$_POST['vemail'];
if (!$email){
echo "Don't forget to include your email adress! Otherwise we can't get back to you.";
} else {
$subject = $_POST['sub'];
$message = $_POST['msg'];
$headers = 'From:' . $emailConfirmed . "\r\n"; // Sender's Email
$headers .= 'Cc:' . $emailConfirmed . "\r\n"; // Carbon copy to Sender
// Message lines should not exceed 70 characters (PHP rule), so wrap it
$message = wordwrap($message, 70);
// Send Mail By PHP Mail Function
mail("marc.murray.92@gmail.com", $subject, $message, $headers);
echo "<script>$('#thankyouModal').modal('show')</script>";
};
}
}
?>
HTML for the modal in question:
<div class="modal fade" id="thankyouModal" tabindex="-1" role="dialog">
<div class="modal-dialog">
<div class="modal-content">
<div class="modal-header">
<button type="button" class="close" data-dismiss="modal" aria-hidden="true">×</button>
<h4 class="modal-title" id="myModalLabel">Thank you for pre-registering!</h4>
</div>
<div class="modal-body">
<p>Thanks for getting in touch!</p>
</div>
</div>
</div>
</div>
3 Answers

Greg Kaleka
39,021 PointsHi Marc,
Couple of things:
- On your page, the id appears to be #thanks. That could do it, unless I'm just missing the #thankYouModal element
- It looks like you're loading JQuery at the bottom of that modal div. Any chance you're trying to call JQuery code in your PHP before the JQuery library has been loaded? Try adding the JQuery link to your header, and see if it solves the problem

Greg Kaleka
39,021 PointsHi Marc,
FYI, I cleaned up your code spacing for my own ease of reading.
Can we see the rest of the page HTML and the CSS? Your php looks fine, so the issue is elsewhere.
Best,
-Greg
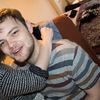
Marc Murray
4,618 PointsHi Greg, thanks for taking a look! Added a link to the relevant page, and the markup for the modal in question.
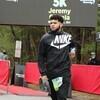
Jeremy Canela
Full Stack JavaScript Techdegree Graduate 30,766 PointsAfter submiting a form, the page will refresh. The code you have only executes once the user submits the form. Once the user submits the form, the script is executed, and then the page refreshes immediately. Something I would do is set a session once the user submits the form. For example:
<?php
if(isset($_POST['submit'])) { // Once the user clicks the submit button
if($_POST["vname"]==""||$_POST["vemail"]==""||$_POST["sub"]==""||$_POST["msg"]=="") { // If an input field is empty
} else {
$_SESSION['formSubmitted'] = true; // Sets session once form is submitted and input fields are not empty
}
}
if(isset($_SESSION['formSubmitted']) && $_SESSION['formSubmitted'] === true) {
echo "<script>$('#thankyouModal').modal('show')</script>"; // Show modal
unset($_SESSION['formSubmitted']; // IMPORTANT - this will unset the value of $_SESSION['formSubmitted'] and will make the value equal to null
}
This is just an example, so I wouldn't recommend copying the code. In the If statement, where you check if the input values are empty, I think you should use the PHP empty() function. Hope it works! Any questions please comment :)!
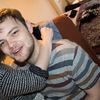
Marc Murray
4,618 PointsWhat I find strange about this is using JS to activate things like alerts, and echoing text onto the page etc is working fine. Is it specific to something about modals that the page refresh is causing an issue?
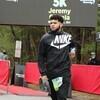
Jeremy Canela
Full Stack JavaScript Techdegree Graduate 30,766 PointsIt has nothing to do with the JS :)! What your code is doing is once the form is submitted, show the modal right? But once the user submits the form, the page will be refreshed immediately and the code will ONLY run once the user submits the form.
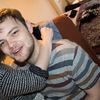
Marc Murray
4,618 PointsHmm so would changing the URL hash be a better option? Something along these lines?
if (window.location.hash === "#thanks") {
$('#thankyouModal').modal('show');
}
I've been using this for other areas of the site and thought it would be a viable alternative, but again it doesn't seem to work very well. I'm not familiar with sessions.

Greg Kaleka
39,021 PointsHi Jeremy,
None of the JS is being fired until the page load that happens on the POST request, so I don't think this is the problem, unless I'm misunderstanding you. All of this is happening inside
Cheers,
-Greg
Marc Murray
4,618 PointsMarc Murray
4,618 PointsHi Greg, Yeah the #thanks id was a mistake I made when adding the code to my question. Moving JQuery up worked perfectly! Well, when using the method of checking the hash of the URL, which seems to be a fine method to suit my purposes at the minute. After several days of banging my head against a wall on a simple issue I just had to move JQuery up. Webdev can be funny sometimes. Thanks a million! :)
Greg Kaleka
39,021 PointsGreg Kaleka
39,021 PointsHah glad you got it working! Always good to make sure dependencies are being loaded before using them :).
Happy coding,
-Greg