Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial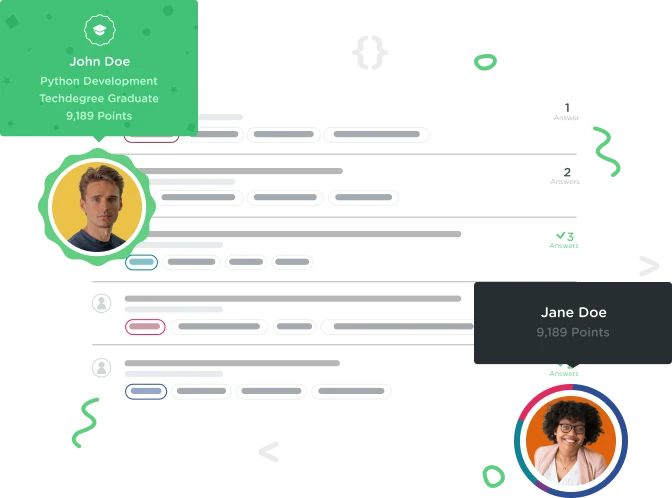
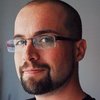
fbenedetti
2,010 PointsDo we need to check for non-letters? (TeacherAssistant.java)
Hi there!
I wonder if we'd check also for non-letters in this task. My second check was like this:
if (fieldName.charAt(1) != Character.toUpperCase(fieldName.charAt(1))){
throw new IllegalArgumentException("Second letter must be uppercase!");
}
I was puzzled to see that "m_first_name" still passed. Apparently, '_' is evaluated as uppercase:
java> String fieldName = "m_first_name"
java.lang.String fieldName = "m_first_name"
java> fieldName.charAt(1) != Character.toUpperCase(fieldName.charAt(1))
java.lang.Boolean res2 = false
java> Character.isLetter(fieldName.charAt(1))
java.lang.Boolean res1 = false
As '_' is not a letter, I had to add a third condition to the code in order to pass the test:
if (! Character.isLetter(fieldName.charAt(1))){
throw new IllegalArgumentException("Second letter must be a letter!");
}
if (fieldName.charAt(1) != Character.toUpperCase(fieldName.charAt(1))){
throw new IllegalArgumentException("Second letter must be uppercase!");
}
return fieldName;
Now, I wonder if that check is required or if there's a more elegant way to achieve the same result.
Cheers, F.
PS: I had a lot of enlightening troubles finding out that one cannot apply methods to primitives...
http://stackoverflow.com/questions/1418296/what-does-it-mean-to-say-a-type-is-boxed
My code that passed the task:
public class TeacherAssistant {
public static String validatedFieldName(String fieldName) {
// These things should be verified:
// 1. Member fields must start with an 'm'
if (fieldName.charAt(0) != 'm') {
throw new IllegalArgumentException("Field must start with an m character!");
}
// 2. The second letter in the field name must be uppercased to ensure camel-casing
// NOTE: To check if something is not equal use the != symbol. eg: 3 != 4
if (! Character.isLetter(fieldName.charAt(1))){
throw new IllegalArgumentException("Second letter must be a letter!");
}
if (fieldName.charAt(1) != Character.toUpperCase(fieldName.charAt(1))){
throw new IllegalArgumentException("Second letter must be uppercase!");
}
return fieldName;
}
}
3 Answers

Craig Dennis
Treehouse TeacherAlso....the reason why '_'
is appearing to not be equal to Character.toUpperCase('_')
is a little tricky. In Java the equality operator ==
is talking about if they represent the same object in memory. Much like String
s this is uber confusing at first...remember this:
"Weird" == new String("Weird") // This is false
"Weird".equals(new String("Weird")) // This is true
So char
s behave similarly
'_' == Character.toUpperCase('_') // returns false
// The wrapper class exposes the equal method. chars by themselves don't have this capability
new Character('_').equals(Character.toUpperCase('_')) // returns true
We'll explore this more in an upcoming course.
Hope that helps!
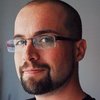
fbenedetti
2,010 PointsThank you so much, Craig! I am looking forward to the next Java track! :)

Craig Dennis
Treehouse TeacherNice job! This is handy knowledge
Character.isUpperCase('_'); // returns false
Nice work digging around and sussing that out!