Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial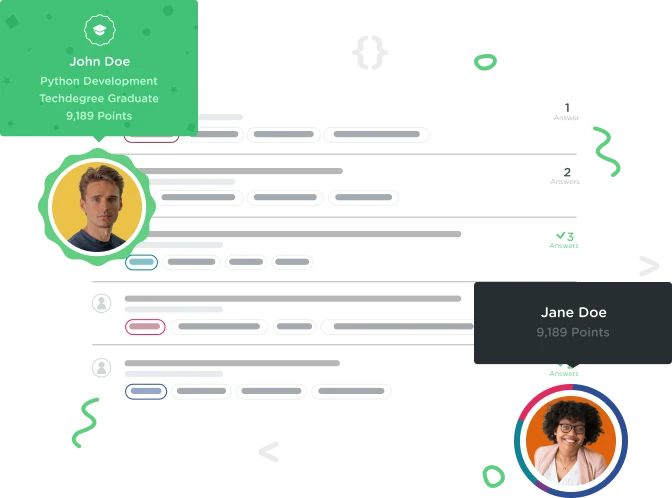
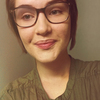
Pauliina K
1,973 Pointsdo while
I don't really understand how the do while loop works. I've tried to read some stuff online, but I still can't seem to get my code right. I think the video that explained this wasn't thorough enough, because I understood everything but the do while loop. So, what am I supposed to do here? And how? Please explain it as well. Thanks in advance.
// I have initialized a java.io.Console for you. It is in a variable named console.
String response;
do {
response = console.readLine("Do you understand do while loops?");
while(response.equalsIgnoreCase("yes"));
}
2 Answers

Matthew Mocniak
1,699 PointsHi Pauliina, and welcome!
Loops are an essential part of any code structure and allow you to cycle through common tasks without repeating code.
There are different kinds of loops - "for loops" and "do/while loops". For loops allow you to specify the number of times your code will repeat itself. Say you have a counter, and you wanted to add the number 1 to that counter every cycle, but you know that you will always want to count to 10... You could configure your for loop to run ten times and add 1 each time.
A do/while loop is best used when you aren't exactly sure when the loop will end. In the code example above, you are looping to check if the code inside the {brackets} meets the criteria of your while( ) statement.
This uses boolean logic, which is simply true or false.
// I have initialized a java.io.Console for you. It is in a variable named console.
String response;
// here, I initialized the string value called "response". We will use it to compare with our loop.
do {
response = console.readLine("Do you understand do while loops?");
// This loop will continually ask "Do you understand do while loops?" and assign it to "response" until the while(condition) is met
} while(response == "No");
// This states that as long as the response value is "No", which is being set right here, then the code inside of the do {brackets} will loop
console.printf("Because you said %s, you passed the test!", response);
// We are able to exit the loop, because hypothetically, our user entered the text "Yes", which broke the conditional rule in the while(condition).
By assigning new values to the String inside the loop instead of at the beginning, it allows us to be more flexible with what values we can assign to that string. A String can be changed to any combination of characters or words, even though it's actual name may remain the same.
I hope this helps you! Don't give up on learning the powers of do while loops! Once you see how they can increase the efficiency and workflow of a program, you will certainly use them more often!
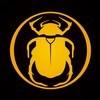
rydavim
18,814 PointsWelcome to Treehouse!
Your answer is very close, so you've got the right idea. Remember that the while evaluation is not part of the loop, you want to evaluate it after the loop runs once.
// I have initialized a java.io.Console for you. It is in a variable named console.
String response;
do {
response = console.readLine("Do you understand do while loops?");
} while(response.equalsIgnoreCase("yes"));
The idea is that you always want the code inside the loop to run at least once. So you do the code once, and then you evaluate if the response is yes.
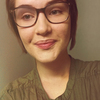
Pauliina K
1,973 PointsThanks! I realised I had written "yes" instead of "no", as well. Once I changed that and changed where the curly bracket was placed, it worked!! Thanks a lot!
Even though I now got it right, I feel like I haven't understood this whole loop thing. I don't even understand the concept of a loop, why do you need something to loop? And how does it loop? Does my "not understanding"-part make sense? It barely even does to me. I just know that loops don't make sense to me.
And I never got what a boolean does. I know what it is in terms of algebra, but I don't know what it means in Java, why and how you use it.
Also, why do you just write the String name and a colon, and then "explain" it inside the loop? Why not explain it where it's declared, and then just write the String name alone?
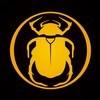
rydavim
18,814 PointsIn this case, you're using a loop because you want to allow the user to give a response multiple times if necessary.
// I have initialized a java.io.Console for you. It is in a variable named console.
String response; // This is initializing the variable 'response', so that you can use it to store values.
do { // You're using a 'do' here to make sure the code runs at least once.
response = console.readLine("Do you understand do while loops?"); // What if they answer 'no' here? We want to run it again.
} while(response.equalsIgnoreCase("no")); // If the answer it no, run the loop again.
This is probably not the best example of demonstrating what loops are good for, since this program doesn't really do anything. Because this is an extremely simple example, there are several different ways to write it - including the way you describe in your last question. You could set the string 'response' equal to 'no' to start with and then use a normal while loop.
I'm actually not particularly familiar with Java, so it would be difficult to give you a better example in proper Java syntax. The below may not actually run correctly, but should give you an example of why you might want to use a loop.
String response; // They haven't guessed the password yet, so we don't know what their response is.
String password = "sesame";
do {
response = console.readLine("What is the password to learn the secret of pie making?");
} while (! (response.equalsIgnoreCase(password))) // If they haven't given the right password, let them guess again.
console.printLine("You know the password! The secret to pie making is using vodka instead of water in the crust.");
Hopefully that makes sense.
Edit - It looks like Matthew Mocniak has some actually Java experience, and was able to give you a better answer. :)
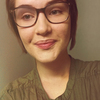
Pauliina K
1,973 PointsIt did make -more- sense at least! Thank you!
Pauliina K
1,973 PointsPauliina K
1,973 PointsOuuuh, I think I got it! The code box with comments made it much easier to understand! Thank you! So, in this example, now that we assign the value to the string -inside- the loop instead of at the beginning, does that mean that we can change it's value in all different loops and similar? Does "String response;" literally just mean that "response" is a variable?
But I do understand why we use do while loops now! And I actually understand -how- it works! Thanks a ton! It's like a nagging friend, continuously asking the same question until you give 'em the answer they want! Haha!
Lastly though, I do now know how the do while loops works, but, I still can't figure out how this code works. Craig introduced too many new terms in one video (boolean, loops etc). It's a part of the Java Basics course, but it just doesn't make sense to me. Could you/someone explain this code block by block? I really want to understand this! And not just in this exact context, but in general. Thanks in advance!