Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial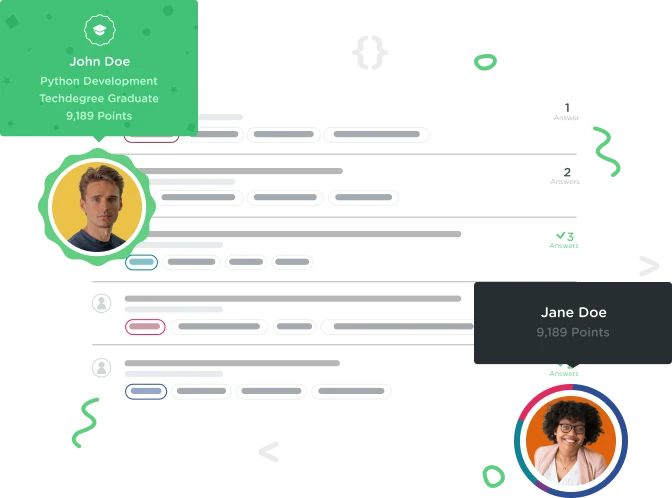

Daniel Hunter
4,622 PointsDocumentation and API request URL no longer exist - Solution found!
as the documentation no longer exists, when following the instructions you will find that you get a 400 or 404 response code and an error message saying unauthorised or not found. I have managed to play with the code a bit to get it working.
when creating a new api key with azure, make sure to name the api key the same as the header you are using, e.g. Ocp-Apim-Subscription-Key, otherwise you do not have authorisation to make the request.
find a valid URL. I used a bing news search, the results you will get back are not what Carling gets in the video but at least you have a successful request and receive some data back from the api.
e.g. https://api.cognitive.microsoft.com/bing/v7.0/news. (see code below)
public static string GetNewsForPlayer(string playerName)
{
var key = "<YOUR API KEY GOES HERE>";
var newsSearchURL = "https://api.cognitive.microsoft.com/bing/v7.0/news";
var webClient = new WebClient();
webClient.Headers.Add("Ocp-Apim-Subscription-Key", key);
byte[] searchResults = webClient.DownloadData(string.Format(newsSearchURL));
using (var stream = new MemoryStream(searchResults))
using (var reader = new StreamReader(stream))
{
return reader.ReadToEnd();
}
}
Alternatively, you can find different api's that are available to make requests to. e.g. https://www.football-data.org which, when you regester, they will email you a API key and name to insert into your code and make the requests.
(see code below)
public static string GetNewsForPlayer(string playerName)
{
var key = "<YOUR API KEY GOES HERE>";
var newsSearchURL = "https://api.football-data.org/v2/teams";
var webClient = new WebClient();
webClient.Headers.Add("X-Auth-Token", key);
byte[] searchResults = webClient.DownloadData(string.Format("newsSearchURL "));
using (var stream = new MemoryStream(searchResults))
using (var reader = new StreamReader(stream))
{
return reader.ReadToEnd();
}
}
Or, you can follow the new documentation to get football results (again, the results are not the same as the video)
(see code below)
public static string GetNewsForPlayer(string playerName)
{
var key = "<YOUR API KEY GOES HERE>";
var client = new NewsSearchClient(new ApiKeyServiceClientCredentials(key));
var newsResults = client.News.SearchAsync(query: playerName, market: "en-us", count: 10).Result;
if (newsResults.Value.Count > 0)
{
var firstNewsResult = newsResults.Value[0].Description;
Console.WriteLine($"TotalEstimatedMatches value: {newsResults.TotalEstimatedMatches}");
Console.WriteLine($"News result count: {newsResults.Value.Count}");
return firstNewsResult;
}
else
{
return "No news found on this player";
}
}
I hope this helps anyone struggling to get past this tutorial.
2 Answers
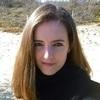
Linda de Haan
12,413 PointsThanks man! Great work!
I also put a response on this video for getting the JSON special paste and the API key for those who struggle getting that, but somehow didn't get linked to this video. I'll just put a link to it here so everything is in one post. https://teamtreehouse.com/community/the-newssearch-class-json-special-paste-result-and-getting-the-api-key

Mark Taylor
13,530 PointsProps to those providing solutions! Hopefully someone will find this small addition helpful as well.
Daniel's first solution is the closest to what's demonstrated in the video, but it lacks a search feature. As a result, returned news articles are simply the top news of the day. You can modify the newsSearchURL to accept a search parameter by appending the final bit of URL (including modification) as seen in the video, which I've done below.
var newsSearchURL = string.Format("https://api.cognitive.microsoft.com/bing/v7.0/news/search?q={0}&mkt=en-us", playerName);
Hibe Davidian
3,521 PointsHibe Davidian
3,521 PointsAwesome, thank you Linda, you're an angel!