Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial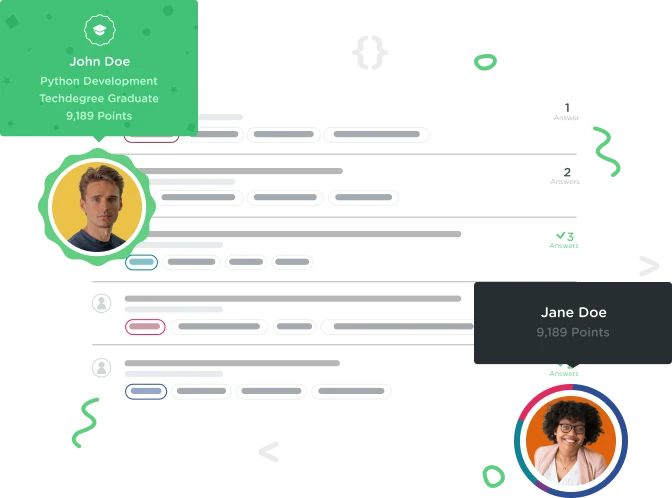

Arania Jain
10,344 PointsError: cannot find symbol symbol: class PezDispeForMnO
Here are the codes
public class PezDispeForMnO
// making a classs
// generally we begin class with a capital letter(easy to identify)
{
public final int NUM_PEZ = 12;
// creating an object with size 12
// Use of "final" so that nobody can change it
private String mcharName;
// Use of "m" before charName to denote it as a member
public PezDispeForMnO(String CharName)
// making a constructor with 1 field/parameter/argument
{
mcharName= CharName;
}
public String getCharName()
{
return mcharName;
}
}
The main code
public class MethsAndObjects
{
public static void main(String[] args)
{
//Code begins from here
// Coode to learn Constructors
// PezDispenser(ek toffee k brand ka naam he) is a new class that we have vreated
// 1 dibbe me hamesha sirf 12 toffee hoti he
System.out.println("Creating a new Pez Dispenser");
PezDispeForMnO dispenser = new PezDispeForMnO("Leo");
System.out.printf("The dispenser name is %s\n", dispenser.getCharName());
}
}
4 Answers

Nikolay Mihaylov
2,810 PointsHi, looking at your post I think something went very bad when you were posting the code snippet. If I am not wrong it looks something like that, with a few comments more or less ( probably less ).
public class PezDispeForMnO {
// making a classs
// generally we begin class with a capital letter(easy to identify)
// creating an object with size 12
// Use of "final" so that nobody can change it
public final int NUM_PEZ = 12;
private String mcharName;
// Use of "m" before charName to denote it as a member
public PezDispeForMnO(String CharName) {
mcharName= CharName;
}
// making a constructor with 1 field/parameter/argument
public String getCharName() {
return mcharName;
}
}
public class MethsAndObjects {
public static void main(String[] args) {
System.out.println("Creating a new Pez Dispenser");
PezDispeForMnO dispenser = new PezDispeForMnO("Leo");
System.out.printf("The dispenser name is %s\n", dispenser.getCharName());
}
}
So I ran it on a workspace and everything seems ok. This is my console output.
treehouse:~/workspace$ javac PezDispeForMnO.java
treehouse:~/workspace$ javac MethsAndObjects.java
treehouse:~/workspace$ java MethsAndObjects
Creating a new Pez Dispenser
The dispenser name is Leo

Arania Jain
10,344 PointsYes, the file name is exactly PezDispeForMnO.java. It is working on console. But it gives error when I use java-repl. The file is even getting loaded, but when I type the command PezDispeForMnO pd = new PezDispeForMnO, it gives error. I have tried running the code for more than 5 times, but everytime I get the same error.
Here is how it looks--
java> :load PezDispeForMnO.java
Loaded source file from PezDispeForMnO.java
java> PezDispeForMnO pd = new PezDispeForMnO("Leo");
ERROR: cannot find symbol
symbol: class PezDispeForMnO
location: interface Evaluation
PezDispeForMnO method$atwpkir8yvxb3f7docn9();
^
Thank you very much for replying.

Allen-Michael Grobelny
20,851 PointsIt looks like there's a typing error in your class declaration:
public PezDispeForMnO(String CharName)
// making a constructor with 1 field/parameter/argument
{
mcharName= CharName;
}
You need to place a space between mcharName and the = symbol, like so:
public PezDispeForMnO(String CharName)
// making a constructor with 1 field/parameter/argument
{
mcharName = CharName;
}

Arania Jain
10,344 PointsDone. But the error persists.

Allen-Michael Grobelny
20,851 PointsTry importing the PezDispeForMnO class in your MethsAndObject file:

Arania Jain
10,344 PointsI rewrote the code and the problem is solved. Thank you for replying.
Craig Dennis
Treehouse TeacherCraig Dennis
Treehouse TeacherDid you you name your file PezDispeForMnO.java Arania Jain ? Case matters.