Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial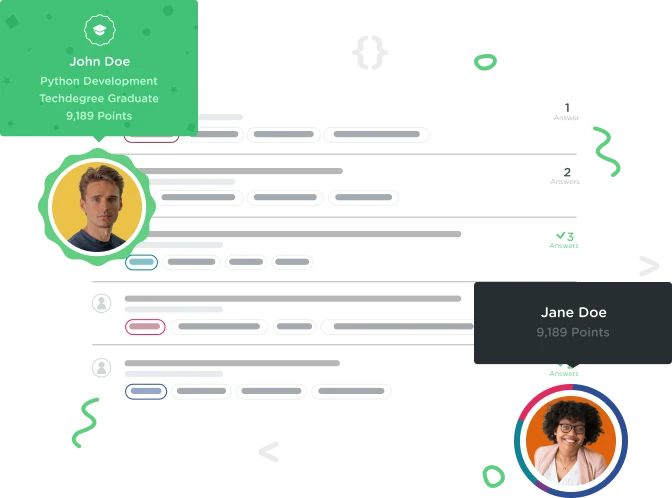

Tatenda Marufu
10,506 Pointserror check
help
string input = Console.ReadLine();
int temperature = int.Parse(input);
if(temperature < 21)
{
Console.WriteLine("Too cold!");
}
else if(tempearature = 21 || 22)
{
Console.WriteLine("Just right.");
}
else(temperature > 22)
{
Console.WriteLine("Too hot!");
}
3 Answers
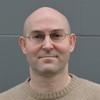
Ted Dunn
43,783 PointsThere are several problems in your else if clause:
First, you have mis-spelled temperature. Second, you are using a single equal sign (=) instead of a double equal sign(==) to attempt a comparison. Single equal is for assignment, double equal is for comparison. Finally, you cannot perform a logical comparison in the format you have listed. The variable must be checked against each value individually. If you wanted to perform the comparison you are attempting, it would need to look like this:
else if (temperature == 21 || temperature == 22)
{
stuff inside...
}
Finally, you do not need to include any conditions in an else clause (ie. you leave out the temperature > 22) as it will be executed if all the preceding clauses are false.
Personally, the way I would write this code as follows:
if (temperature < 21)
{
too cold
}
else if (temperature > 22)
{
too hot
}
else
{
just right
}
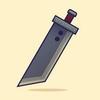
Allan Clark
10,810 Pointsjust eyeballing it looks like your issue is on this line:
else if(tempearature = 21 || 22)
there are 2 issues here. the first is the '=' is the assignment operator not comparison, you will want to change that to '=='.
secondly when you want to chain logic you must repeat the variable. so to check if temp is 21 or 22 you would write the code like.
temp == 21 || temp == 22
if changing this doesnt work please post the error you are getting

Tatenda Marufu
10,506 Pointsthanks for the corrections