Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial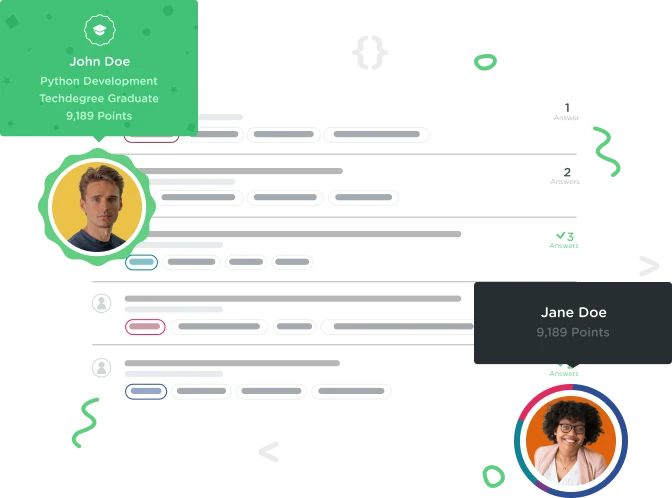
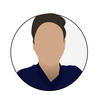
Ryan Darwent
871 PointsError: Program.cs(129,250): error CS1525: Unexpected symbol `end-of-file'
Not sure what to do, also, when I tried a little earlier it worked well adding the minutes, but when I try to quit a bunch of error code appears. This is my code:
using System; // If you write "using System;" at the top of your code, you no longer have to include the word "System" in your code. Ex: System.Console.Write = Console.Write (If you have "using System;" at the top of the script). // There are many of these that you can use, and they are used to reduce the amount of typing that you have tp do in the code.
namespace Treehouse.FitnessFrog
// Namespaces are another way for organization in C#. // Namespaces are used to organize its many classes. // You can have as many Namespaces as you want, just put dots (.) between each name.
{ class Program
// All code is contained in a class; Class = way of organizing code ; Everything in curly brace is part of class; Program can have any number of classes; Classes are usually contained in their own file - Tell them apart by naming them.
// To Compile, type "mcs Program.cs" in console.
// To Run, type "mono Program.exe" in console.
// The right most word is the name of the method, left of that is the name of the class, and everything that remains to the left of that is the namespace.
// REPL | Stands for READ, EVALUATE, PRINT, LOOP.
// One "=" sign means equals, but two, "==", is known as an equality operator. If the left = the right, it evaluates to true, if not, it evaluates to false.
// *WHAT PARAMETERS ARE* Parameter Example: void SendMessage(string message, string recipient) | The parameters are the "string message, string recipient" part of the method.
// Example of identifying parameters
// Void SendMessage(string message, string recipient) | Question: Type of the first parameter | Answer: string
// Void SendMessage(string message, string recipient) | Question: Name of the method | Answer: SendMessage
// Void SendMessage(string message, string recipient) | Question: Name of the second parameter | Answer: recipient
{ // Static keyword allows the method to be called without instantiating an instance of the class. // Classes often have multiple Methods instead of them; Class can have any other of Methods; Methods are used to further organize code into smaller chunks. // Method named Main is what runs first | One method has to be named Main, others can be named whatever you want. // Method has four parts; Method has name Ex: "Main"; All the code for the program will be written inside of the Method; Method can use other Methods, known as "calling a Method"; Third part of Method is perameters, put inside the parenthesis. // The word void means it will not return anything to another method. // The Four P's of Problem Solving are: Prepare, Plan, Perform and Perfect. // Prepare = This is where we understand and diagnose the problem. | Plan = This is where we organize everything before acting. | Perform = We simply put the plan into action. | Perfect = Check to see if creation has solved problem, and how to make better. Use 4 P's again to improve.
static void Main()
{
// This is a new variable that will store the running total and initialize it to 0.
// Int is the name of the type that can store numbers. | Int = Integer
// No need for quotation marks, as it is not a string. (Number is 0, instead of "0").
// Integers are whole numbers ONLY, NO DECIMALS OR QUOTATIONS.
int runningTotal = 0;
// In math, a boolean (bool) is something that can be either true or false.
// Just like STRING can ONLY STORE TEXT, and INT can ONLY STORE INTEGERS, BOOLEAN can ONLY STORE the value of: TRUE or FALSE.
// If we NEVER change the value of keepGoing, the loop will run FOREVER, therefore, the program will run forever until it is forced to stop.
bool keepGoing = true;
// Below is a while loop, this is the most simple type of loop.
// A while loop has two parts.
// The first part is everything between its curly braces is the body, everything in the body is what we want to be looped/repeated.
// The second part is the condition, everything between the parenthesis. This is the part that determines if the loop should run/repeat. If it's true, it'll repeat; if it's false, it'll skip over it.
// Before the program will enter the loop, it will check the value of the bool "keepGoing", and it will skip it or execute it, depending on if it's true or false.
while (keepGoing)
{
// Prompt the user for minutes exercised
// Needs additional information in order to run, info will be in parenthesis. This method needs to know what we want to print to the screen, which will be listed in the parenthesis.
// Make sure to write the text in double quotes, also known as a string.
// Make sure to END ALL LINES WITH A SEMI-COLON.
// The double slashes are there so that the quotations around "quit", can be there and not end the line of code.
Console.Write("Enter how many minutes you exercised or type \"quit\" to exit: ");
// Way to get the user to respond, and get their response to the console. | This tells the console to allow the user to enter the response. | Known as "reading from the console"
// When this method is called, the console will read what the user types in until they hit the "ENTER"/"RETURN" key on the keyboard.
// To assign "System.Console.ReadLine" to the entry variable, set them equal to each other.
// This means that the string returned from this method will be stored in the entry variable.
string entry = Console.ReadLine();
// The difference between an if statement and a while loop, is that If statements DO NOT LOOP.
// When the program gets to an if statement, it checks the condition. If the condition evaluates to true, then the code in the body of the "if" will get executed. If the condition evaluates to false, it skips past the body of the if.
if (entry == "quit")
{
keepGoing = false;
}
else
{
// Stores the converted integer into a new variable called "minutes".
// You can add minutes to running total and then assigns the results back into running total. (It adds running total and minutes, and both of them become the runningTotal.).
int minutes = int.Parse(entry);
runningTotal = runningTotal + minutes;
// Saving what the user enters into a variable so that it can be used later. | A variable is a way to temporarily store information that the code can use. | Variables can be created by first saying what type of information is needed to store.
// In the example below, the variable type is "string", and the variable needs a name, names can have letters, numbers, or an underscore character, but cannot start with a number. Name should be descriptive of what it's meant to store.
// In the example below, the name of the variable is meant to store the person's first name, so the variable name is "firstName". Then, we initialized the "firstName" to "Ryan".
// Things on both sides of the equal sign must be the same type, in the example below, they're both strings.
// The WriteLine method only writes a whole line to the console, just like typing a line to the console, then pressing "ENTER" at the end to move the cursor down to the next line.
// Everything printed on the "WriteLine" method, will be printed on its own line.
// All of the Console.Write code lines below can be compiled into one line, written as " Console.WriteLine("You've entered " + entry + " minutes"); " As shown below.
// Console.Write("You've entered");
// Console.Write(entry);
// Console.WriteLine(" minutes");
// The plus sign is used to stick one string to another, known as string concatenation, or concatenating strings.
// The plus sign is known as the concatenation operator.
Console.WriteLine("You've worked out for " + runningTotal + " minute(s)");
// Below are the coding challenges, may be good to refer to.
// "Declare a string variable named bookTitle. - Don’t assign it anything." | Answer: string bookTitle;
// "Initialize bookTitle with the title of your favorite book." | Answer: string bookTitle = "Book Name";
// "Use the System.Console.Write() method to print "Enter a book title: " to the console." | Answer: System.Console.Write("Enter a book title: ");
// "Use the System.Console.ReadLine method to read the user’s response from the console and store it in a variable named bookTitle." | Answer: string bookTitle = "Whip" + System.Console.Write("Enter a book title: "); + bookTitle = System.Console.ReadLine();
//string firstName = "Ryan";
// Add minutes excerised to total
// Display total minutes exercised to the screen
// Repeat program until the user quits
}
}
Console.WriteLine("Goodbye!");
}
}
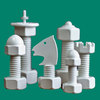
Steven Parker
231,269 PointsWhen posting code, always use the instructions for code formatting in the Markdown Cheatsheet pop-up below the "Add an Answer" area.
Or watch this video on code formatting.
1 Answer
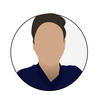
Ryan Darwent
871 Pointsokay, sorry, I never knew how it was done! I just decided to go ahead and take the knowledge I learned to begin a course I bought from Udemy a while back, thanks guys!
Bradley Green
7,442 PointsBradley Green
7,442 PointsThe only problem I'm seeing is when I copy the code you posted, there is a missing "}" so it wouldn't build. When I add the bracket, build and run it, everything works as expected.
Can you copy the error you're seeing into a comment?