Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial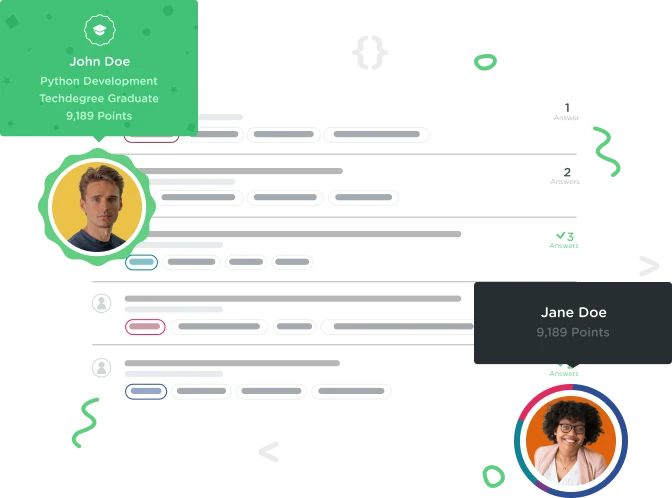

Livia Lulushi
1,777 PointsError: variable post might not have been initialized --> how do I initialize "post"?
Or anything with non-standard class type, for that matter.
public class ForumPost {
private User mAuthor;
private String mTitle;
private String mDescription;
public ForumPost(User author, String title, String description) {
mAuthor = author;
mTitle = title;
mDescription = description;
}
public User getAuthor() {
return mAuthor;
}
public String getTitle() {
return mTitle;
}
public String getDescription() {
return mDescription;
}
// TODO: We need to expose the description
}
public class User {
private String mFirstName;
private String mLastName;
public User(String firstName, String lastName) {
// TODO: Set the private fields here
mFirstName = firstName;
mLastName = lastName;
}
public String getFirstName() {
return mFirstName;
}
public String getLastName() {
return mLastName;
}
}
public class Forum {
private String mTopic;
public Forum (String topic) {
mTopic=topic;
}
public String getTopic() {
return mTopic;
}
public void addPost(ForumPost post) {
System.out.printf("New post from %s %s about %s.\n",
post.getAuthor().getFirstName(),
post.getAuthor().getLastName(),
post.getTitle());
}
}
public class Example {
public static void main(String[] args) {
System.out.println("Starting forum example...");
if (args.length < 2) {
System.out.println("first and last name are required. eg: java Example Craig Dennis");
}
Forum forum = new Forum("Java");
// Take the first two elements passed args
User author = new User("Livia", "Lulushi");
// Add the author, title and description
ForumPost post = new ForumPost(post.getAuthor(), "Wins", "Java");
forum.addPost(post);
}
}
2 Answers

Anthony Conti
2,606 PointsI think Kristian nails it on the head. Don't worry, though. I had just as much trouble as you, if not more.
You want to make sure that you're paying attention to one of the last videos in the Java Objects course. Craig shows us how to use the Strings array that is passed into the main method. Instead of hard-coding your own name into the new author object, you want to pass in the names or arguments that he desires.
If we take a look at your new user object in Example.java, we want to see:
User author = new User(args[0], args[1]);
You are then creating a new forum post object. Kristian mentions that you should pay attention to the user object that you just created, named author. This is very important. Actually, it's the answer:
Forum post = new ForumPost(author, "Wins", "Java");
You'll want to go into Forum.java to rework your addPost method. I decided to pass into the addPost method another parameter, User author. Using the new User object that I created, I called the getFirstName and getLastName methods. To print the title, I left the syntax alone. It should look like this:
public void addPost(ForumPost post, User author) {
System.out.printf("New post from %s %s about %s.\n",
author.getFirstName(),
author.getLastName(),
post.getTitle());
}
At any rate, I hope this helpful to you. Let me know if you come up with a more efficient solution.
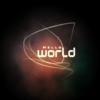
Kristian Terziev
28,449 PointsBasically, you are trying to pass the a non-existing User object as a first parameter. You try to use the author of "post" as a parameter for "post". You cannot do that. Instead you should use the "author" object you created above.
However, you will face another error soon. It will be one due to your distraction. You did not read well what parameters you should pass for the User object. You are not asked for your name - there are specific parameters you should use. Try figuring it on your own. But if you can't, don't worry - that's what we are here for :) Feel free to come back anytime.
Livia Lulushi
1,777 PointsLivia Lulushi
1,777 PointsThe error occurs in the second-to-last line of Example.java