Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial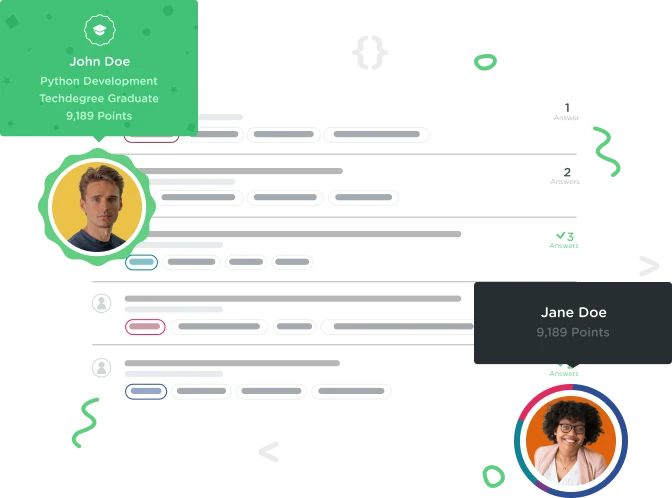

Stefan Elsner
12,452 PointsEven though the li elements appear with the correct class of "empty" or "full", the background color won't change
The JavaScript code I wrote is exactly the same as the one presented in the solution video, and the list items are created with the correct classes of "full" and "empty". This is also verified by the browser's inspector tool. The problem is that the room number's background color doesn't change despite the list item having the correct class. Can someone help me solve this problem?
const xhr = new XMLHttpRequest();
xhr.onreadystatechange = function () {
if(xhr.readyState === 4 && xhr.status === 200) {
const employees = JSON.parse(xhr.responseText);
let statusHTML = '<ul class="bulleted">';
for (let i=0; i<employees.length; i += 1) {
if (employees[i].inoffice === true) {
statusHTML += '<li class="in">';
} else {
statusHTML += '<li class="out">';
}
statusHTML += employees[i].name;
statusHTML += '</li>';
}
statusHTML += '</ul>';
document.getElementById('employeeList').innerHTML = statusHTML;
}
};
xhr.open('GET', '../data/employees.json');
xhr.send();
const roomsXhr = new XMLHttpRequest();
roomsXhr.onreadystatechange = function () {
if (roomsXhr.readyState === 4 && roomsXhr.status === 200) {
const rooms = JSON.parse(roomsXhr.responseText);
let statusHTML = "<ul class='rooms'>";
for (let i=0; i<rooms.length; i += 1) {
if (rooms[i].available === true) {
statusHTML += "<li class='empty'>";
} else {
statusHTML += "<li class='full'>";
}
statusHTML += rooms[i].room;
statusHTML += "</li>"
}
statusHTML += "</ul>";
document.getElementById('roomList').innerHTML = statusHTML;
}
};
roomsXhr.open('GET', '../data/rooms.json');
roomsXhr.send();

Stefan Elsner
12,452 PointsHi Adam. Thank you for your answer.
The CSS file is linked correctly in index.html, as far as I can see.
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<title>AJAX Office Status Widget</title>
<link href='http://fonts.googleapis.com/css?family=Varela+Round' rel='stylesheet' type='text/css'>
<link rel="stylesheet" href="css/main.css">
<script src="js/widget.js"></script>
</head>
<body>
<div class="grid-container centered">
<div class="grid-100">
<div class="contained">
<div class="grid-100">
<div class="heading">
<h1>Corporate Intranet</h1>
</div>
</div>
<section class="grid-70 main">
<h2>Lorem Ipsum Headline</h2>
<p>Sed ut perspiciatis unde omnis iste natus error sit voluptatem accusantium doloremque laudantium, totam rem aperiam, eaque ipsa quae ab illo inventore veritatis et quasi architecto beatae vitae dicta sunt explicabo. Nemo enim ipsam voluptatem quia voluptas sit aspernatur aut odit aut fugit, sed quia consequuntur magni dolores eos qui ratione voluptatem sequi nesciunt. Neque porro quisquam est, qui dolorem ipsum quia dolor sit amet, consectetur, adipisci velit, sed quia non numquam eius modi tempora incidunt ut labore et dolore magnam aliquam quaerat voluptatem. Ut enim ad minima veniam, quis nostrum exercitationem ullam corporis suscipit laboriosam, nisi ut aliquid ex ea commodi consequatur? Quis autem vel eum iure reprehenderit qui in ea voluptate velit esse quam nihil molestiae consequatur, vel illum qui dolorem eum fugiat quo voluptas nulla pariatur?</p>
<p>Sed ut perspiciatis unde omnis iste natus error sit voluptatem accusantium doloremque laudantium, totam rem aperiam, eaque ipsa quae ab illo inventore veritatis et quasi architecto beatae vitae dicta sunt explicabo. Nemo enim ipsam voluptatem quia voluptas sit aspernatur aut odit aut fugit, sed quia consequuntur magni dolores eos qui ratione voluptatem sequi nesciunt. Neque porro quisquam est, qui dolorem ipsum quia dolor sit amet, consectetur, adipisci velit, sed quia non numquam eius modi tempora incidunt ut labore et dolore magnam aliquam quaerat voluptatem. Ut enim ad minima veniam, quis nostrum exercitationem ullam corporis suscipit laboriosam, nisi ut aliquid ex ea commodi consequatur? Quis autem vel eum iure reprehenderit qui in ea voluptate velit esse quam nihil molestiae consequatur, vel illum qui dolorem eum fugiat quo voluptas nulla pariatur?</p>
<p>Sed ut perspiciatis unde omnis iste natus error sit voluptatem accusantium doloremque laudantium, totam rem aperiam, eaque ipsa quae ab illo inventore veritatis et quasi architecto beatae vitae dicta sunt explicabo. Nemo enim ipsam voluptatem quia voluptas sit aspernatur aut odit aut fugit, sed quia consequuntur magni dolores eos qui ratione voluptatem sequi nesciunt. Neque porro quisquam est, qui dolorem ipsum quia dolor sit amet, consectetur, adipisci velit, sed quia non numquam eius modi tempora incidunt ut labore et dolore magnam aliquam quaerat voluptatem. Ut enim ad minima veniam, quis nostrum exercitationem ullam corporis suscipit laboriosam, nisi ut aliquid ex ea commodi consequatur? Quis autem vel eum iure reprehenderit qui in ea voluptate velit esse quam nihil molestiae consequatur, vel illum qui dolorem eum fugiat quo voluptas nulla pariatur?</p>
</section>
<aside class="grid-30 list">
<h2>Meeting Rooms</h2>
<div id="roomList">
</div>
<h2>Employee Office Status</h2>
<div id="employeeList">
</div>
</aside>
<footer class="grid-100">
<p>Copyright, The Intranet Corporation</p>
</footer>
</div>
</div>
</div>
</body>
</html>
Also, there is a reference to the full and empty classes in the CSS file:
ul li {
margin: 15px 0 0;
font-size: 1.6em;
color: #8d9aa5;
position: relative;
}
ul.rooms {
margin-bottom: 30px;
}
ul.rooms li {
font-size: 1em;
display: inline-block;
width: 10%;
padding: 3px 2px 2px;
border-radius: 2px;
margin: 0 3px 3px 3px;
color: white;
text-align: center;
}
ul.rooms li.empty {
background-color: #5fcf80;
}
ul.rooms li.full {
background-color: #ed5a5a;
}
Furthermore, I'm using MAMP and also checked if there was an issue with the local server, but the correct directory is linked. What's puzzling me is that the employee list is shown as it should be, with the 'in' and 'out' flags displayed in the appropriate color. Finding the mistake here is quite challenging to me.
2 Answers

brittany tinsley
Full Stack JavaScript Techdegree Student 10,029 PointsI know its been a minute but it took a while to figure out too. Its the ul classname change it from "bulleted" to "rooms"

Stefan Elsner
12,452 PointsThank you so much, Brittany. Sometimes I just can't find those careless mistakes that hide in my code. But you have finally found it.

Adam VanSlyke
15,988 PointsHmm, I just took your JS and HTML code and put it into a workspace that has a working version of this application. Everything appears to be working fine.
When you run it in MAMP are you seeing any stylization of the webpage? For example the page displays centered on the screen with a nice font (the font isn't the standard Times New Roman you'd see on a non-styled HTML page).
Adam VanSlyke
15,988 PointsAdam VanSlyke
15,988 PointsSo the classes are showing up in the elements in the browser's developer tools, but the colors aren't modifying?
Nothing is standing out in the JavaScript code, so I'd take a look at the index.html file to make sure the css file is linked. Also, take a look at the CSS file to be sure there is a reference to the 'empty' and 'full' classes within that file.
Let me know what you find.