Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial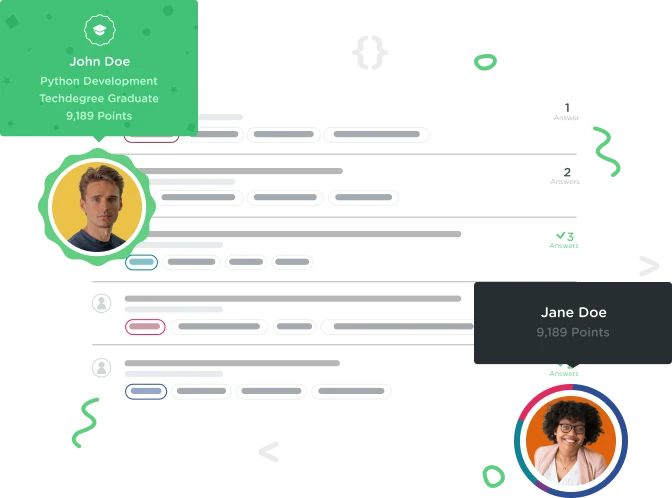

Daniel Lake
6,595 PointsExcept arguments when instantiating a variable
Hey guys,
i'm trying to write some of the stuff we did in the Ruby Track on my own and ask myself the following: When does the instantiated variable take arguments and when not? Here is the code written by Jason (just the beginning of it):
class TodoItem attr_reader :name
def initialize(name) @name = name @complete = false end
This code accepts one argument when i instantiate it. For example: item = TodoItem.new("Milk")
Here is the same spot of the code written by me:
class TodoItem attr_accessor :name, :complete
def create(name, status) @name = name if status.downcase == "complete" @complete = true else @complete = false end item = {name: @name, status: @complete}
return item
end
This code however doesn't accept the two arguments when i try to instantiate it with "item = TodoItem.new("Milk", "incomplete")
Would be great if someone could explain this to me. Thanks in advance :)
1 Answer

Galen Cook
2,463 PointsIf you want to instantiate with two arguments, then you need to have an initialize
method that accepts two arguments. Add this code in:
def initialize(name, status)
@name = name
@status = status
end
The initialize method is run every time you instantiate a class. Your code could work as is, but instead of passing two arguments upon instantiation, you would instantiate then call the create
method you've defined and pass the arguments in there.
item = ToDoItem.new
item.create("Milk", "incomplete")
Daniel Lake
6,595 PointsDaniel Lake
6,595 PointsThanks for your answer. So the initialize method is just there to pass the arguments into the class? If so, I must have missed some seconds of the class :D
Galen Cook
2,463 PointsGalen Cook
2,463 PointsThat's one of the main reasons to use
initialize
, yes!Daniel Lake
6,595 PointsDaniel Lake
6,595 PointsAh okay. So instead of naming my method "create" i'll name it "initialize" and as soon as my class gets instantiated the initialize method gets executed... hope i got that right. Anyway, thank you so much for helping me out.
Seth Reece
32,867 PointsSeth Reece
32,867 PointsHi Daniel,
Just an added note for your question. In Ruby on Rails, your initialize method is just as Galen explained. Create is generally used as a POST method that saves an item in the database.