Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial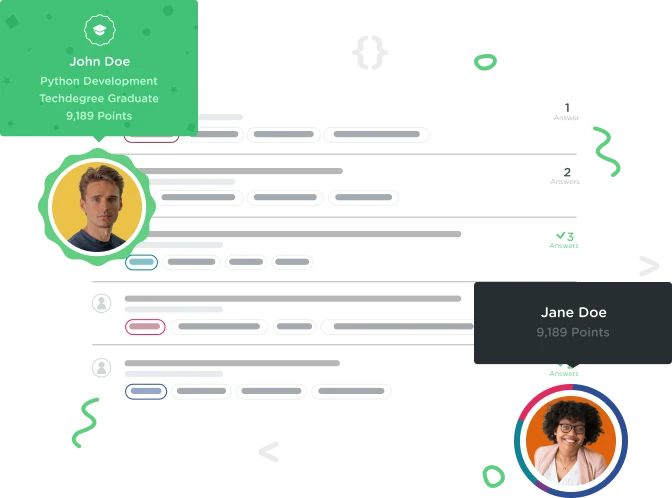
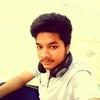
Ashish Mehra
Courses Plus Student 3,575 Pointsexecute() return false
Hey I am practicing login system with oop from codecourse https://www.youtube.com/watch?v=PaBWDOBFxDc&list=PLfdtiltiRHWF5Rhuk7k4UAU1_yLAZzhWc&index=8 . Till the database connectivity everything moving in right direction but when i try to run query execution return false.
//class DB.php
class DB{
private static $_instance = null;
private $_pdo,
$_query,
$_error=false,
$_results,
$_count=0;
private function __construct(){
try{
$this->_pdo = new PDO(
"mysql:".Config::get("mysql/host").";
dbname=".Config::get("mysql/db").";
port=".Config::get("mysql/port")."\",\""
.Config::get("mysql/username")."\",\""
.Config::get("mysql/password")."\"");
}catch(Exception $e){
echo $e->getMessage();
exit();
}
}
public static function get_instance(){
if(!isset(self::$_instance)){
self::$_instance = new DB();
}
return self::$_instance;
}
public function query($sql,$params = array()){
$this->_error = false;
if($this->_query = $this->_pdo->prepare($sql)){
$x=1;
if(count($params)){
foreach ($params as $param) {
$this->_query->bindValue($x,$param);
$x++;
}
}
if($this->_query->execute()){ //here i m geeting value as false
echo "success";
$this->_results = $this->_query->fetchAll(PDO::FETCH_OBJ);
$this->_count = $this->_query->rowCount();
}else{
echo "error";
$this->_error = true;
}
}
}
public function error(){
return $this->_error;
}
}
//index.php
require_once 'core/init.php';
$user = DB::get_instance()->query("Select * from users");
if($user->error()){
echo "No user";
}else{
echo "Ok";
}
I am not getting the reason due to which execute return false every time.
1 Answer

Serhii Lihus
6,352 PointsHi there Ashish!
Let's get straight to the point. Mainly error was in your connection string.
"mysql:".Config::get("mysql/host").";
dbname=".Config::get("mysql/db").";
port=".Config::get("mysql/port")."\",\""
.Config::get("mysql/username")."\",\""
.Config::get("mysql/password")."\"");
A bit messy, but it's ok =)
How dsn connection should look like(from php.net manual):
$dbh = new PDO('mysql:host=localhost;dbname=test', $user, $pass);
Below you can find working sample. Change dbname, username and password(you can also add port to the config array).
<?php
class Config
{
private static $config = array();
public static function setConfig($param_name, $value)
{
self::$config[$param_name] = $value;
}
public static function getConfig($param_name)
{
return self::$config[$param_name];
}
}
Config::setConfig("mysql/host", "localhost");
Config::setConfig("mysql/db", "user");
Config::setConfig("mysql/username", 'username');
Config::setConfig("mysql/password", 'password');
class DB
{
private static $_instance = null;
private $_pdo,
$_query,
$_error=false,
$_results,
$_count=0;
private function __construct()
{
try {
$this->_pdo = new PDO(
"mysql:host=".Config::getConfig("mysql/host").";"
."dbname=".Config::getConfig("mysql/db"),
Config::getConfig("mysql/username"),
Config::getConfig("mysql/password")
);
} catch(Exception $e) {
echo $e->getMessage();
exit();
}
}
public static function get_instance()
{
if(!isset(self::$_instance)){
self::$_instance = new DB();
}
return self::$_instance;
}
public function query($sql, $params = array())
{
$this->_error = false;
$this->_query = $this->_pdo->prepare($sql);
$x=1;
if(count($params)){
foreach ($params as $param) {
$this->_query->bindValue($x,$param);
$x++;
}
}
if($this->_query->execute()) {
$this->_results = $this->_query->fetchAll(PDO::FETCH_OBJ);
$this->_count = $this->_query->rowCount();
} else {
print_r($this->_query->errorInfo());
$this->_error = true;
}
}
public function error()
{
return $this->_error;
}
public function getResults()
{
return $this->_results;
}
}
echo '<pre>';
$user = DB::get_instance();
$user->query("Select * from users");
if(!$user->error()) {
print_r($user->getResults());
} else {
echo "No users in db";
}