Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial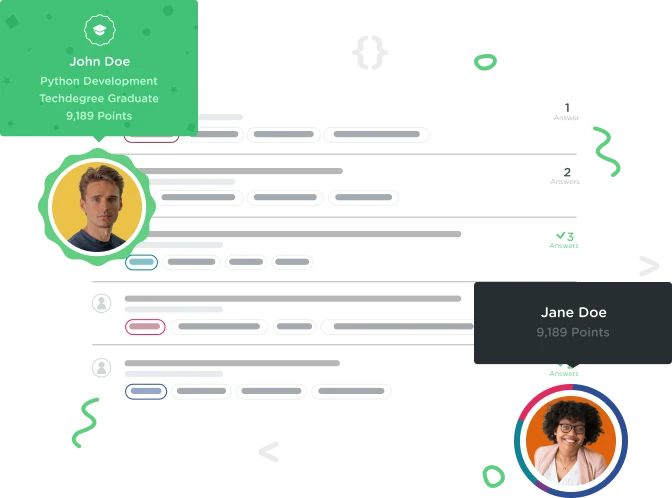

Samuel Savaria
4,809 PointsFeedback on my programs (Averager).
I am doing the 4 side projects that were proposed at the end of C# Basics. I am looking for any type of feedback from the community: How to follow the conventions, improve readability, optimize the program, etc..
This is the first suggestion, the averager. The user enters as many numbers as he wants and when he types "done", the program average them all. In this program I had to declare 3 of the 4 variables at the top of the code, despite my best efforts against it, and I was wondering if it was possible to do otherwise?
using System;
class Averager
{
static void Main()
{
var number = 0.0;
var total = 0.0;
var totalNumber = 0;
Console.Write("Enter any amount of numbers you like or type \"done\" to average them: \n");
while (true)
{
try
{
//Repeatedly prompt the user for numbers
var input = Console.ReadLine();
if (input.ToLower() != "done")
{
//Add all numbers together
//Keep track of the total number of numbers
number = double.Parse(input);
total += number;
totalNumber += 1;
continue;
}
else
{
//When the user types "done", print the average
number = total / totalNumber;
Console.Write("Your average is " + number + "\n");
break;
}
}
catch ( Exception ex )
{
if (ex is FormatException ||
ex is OverflowException)
{
Console.WriteLine("That is not valid input");
continue;
}
throw;
}
}
}
}
1 Answer
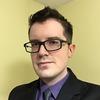
Chris Stowe
2,450 PointsI think your code looks good.
Here is some feedback to help you out:
- Add a namespace (it is good to get into this habit early on).
- Add multiple catch statements (instead of checking the exception type).
- Use the increment operator to increase the variable totalNumber (totalNumber++).
I cannot think of a way to avoid the variables at the top of your code (this is the way that I approached the problem as well). One way to avoid this would be to use a couple of functions. The variables would still need to be declared at the top of one of those functions though.