Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial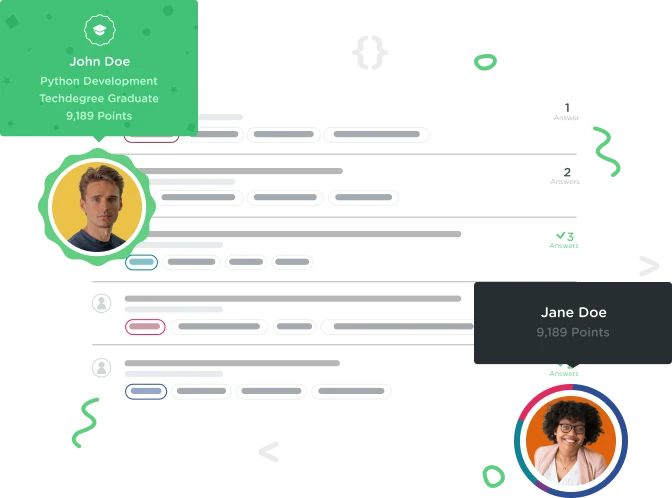

Samuel Savaria
4,809 PointsFeedback on my programs (Calculator).
I am doing the 4 side projects that were proposed at the end of C# Basics. I am looking for any type of feedback from the community: How to follow the conventions, improve readability, optimize the program, etc..
I don't have a lot to say on this one. If anyone has an idea on how to compact it or avoid repetition without using objects and other methods (This is for the C# Basics course) or any other useful tips it would be welcome. Otherwise I'm proud of it, best program of the 4 in my opinion.
using System;
class Program
{
static void Main()
{
while (true)
{
var operation = 0;
var result = 0.0;
var first = 0.0;
var second = 0.0;
var quit = false;
//Prompt the user for a number
Console.Write("Enter a number or type \"quit\" to exit: ");
while (true)
{
var entry = Console.ReadLine();
if (entry.ToLower() == "quit")
{
quit = true;
break;
}
else
{
try
{
first = double.Parse(entry);
break;
}
catch (FormatException)
{
Console.Write("That is not valid input!\n");
continue;
}
}
}
if (quit == true)
{
break;
}
//Prompt the user for an operation (+, -, *, /, ^)
Console.Write("Type \"addition\", \"substraction\", \"multiplication\", \"division\" or \"power\" to select an operation, or type \"quit\" to exit: \n");
while (true)
{
var entry = Console.ReadLine();
if (entry.ToLower() == "quit")
{
quit = true;
break;
}
else if (entry.ToLower() == "addition")
{
operation = 1;
break;
}
else if (entry.ToLower() == "substraction")
{
operation = 2;
break;
}
else if (entry.ToLower() == "multiplication")
{
operation = 3;
break;
}
else if (entry.ToLower() == "division")
{
operation = 4;
break;
}
else if (entry.ToLower() == "power")
{
operation = 5;
break;
}
else
{
Console.Write("That is not valid input! \n");
continue;
}
}
if (quit == true)
{
break;
}
//Prompt the user for another number
Console.Write("Enter another number or type \"quit\" to exit: ");
while(true)
{
var entry = Console.ReadLine();
if (entry.ToLower() == "quit")
{
quit = true;
break;
}
else
{
try
{
second = double.Parse(entry);
break;
}
catch (FormatException)
{
Console.Write("That is not valid input! \n");
continue;
}
}
}
if (quit == true)
{
break;
}
//Perform the operation
if (operation == 1)
{
result = first + second;
}
else if (operation == 2)
{
result = first - second;
}
else if (operation == 3)
{
result = first * second;
}
else if (operation == 4)
{
result = first / second;
}
else if (operation == 5)
{
result = Math.Pow(first, second);
}
//Print the number
Console.Write(result + "\n");
}
}
}
1 Answer
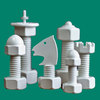
Steven Parker
231,122 PointsTwo optimizations come to mind at first glance.
You could make the operator choice section more compact by using an array and a loop:
operations = ["nil", "addition", "substraction", "multiplication", "division", "power"];
for (var i=1; i < operations.length; i++) {
if (entry.ToLower() == operations[i]) {
operation = i;
break;
}
}
And you could make the performance section a bit more compact by using a switch instead of the if...else chain.