Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial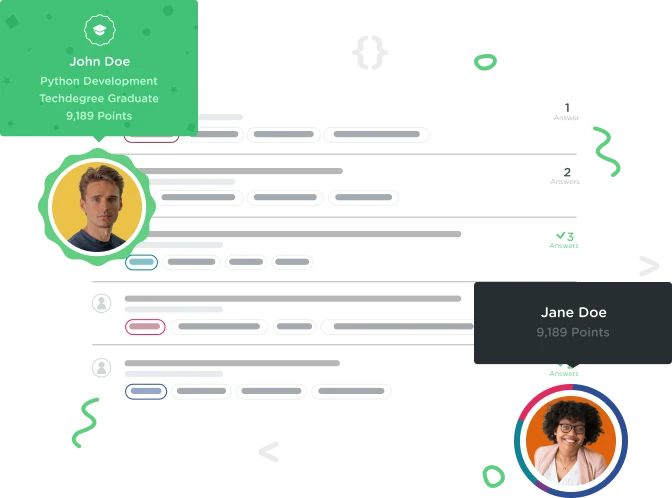

Samuel Savaria
4,809 PointsFeedback on my programs (Math).
I am doing the 4 side projects that were proposed at the end of C# Basics. I am looking for any type of feedback from the community: How to follow the conventions, improve readability, optimize the program, etc..
I am skipping the 3rd project and doing the 4th instead. That is because they are very similar and I am having the same issues with both, but this one included research.
With both programs, I ran into trouble with the loops. I wanted to do like the previous programs: At every step ask for the input. If the user types "quit", exit the program. If they did not enter a valid input, repeat that step, and if they entered the input I was looking for do the next step.
I had five operations (sin, cosine, tangent, square root and logarithm), and in an ideal world I would have used 1 loop for the program as a whole, and 1 loop for each operations. But the problem is, you can't use the values you assigned to variables outside their respective loops, so I couldn't have have seperate loops. I tried having a loop inside a loop, but that created another problem: I could either exit the program (break; at every step), or repeat it (break; at every step except the last), but not both at the same time. So I had to do 1 loop, with if statements inside them. If the user screws up 1 step, he is directed back to the beginning.
Sorry for the long text, anyone have a solution for this?
using System;
class Program
{
static void Main()
{
while (true)
{
try
{
//Prompt the user for an operation
Console.Write("Type \"sine\", \"cosine\", \"tangent\", \"square\" or \"logarithm\" to select an operation, or type \"quit\" to exit: \n");
var entry = Console.ReadLine();
if (entry.ToLower() == "sine")
{
Console.Write("Enter a sine or type \"quit\" to leave: ");
entry = Console.ReadLine();
if (entry.ToLower() == "quit")
{
break;
}
else
{
var parameter = double.Parse(entry);
Console.Write(Math.Asin(parameter)*(180/Math.PI) + "\n");
}
}
else if (entry.ToLower() == "cosine")
{
Console.Write("Enter a cosine or type \"quit\" to leave: ");
entry = Console.ReadLine();
if (entry.ToLower() == "quit")
{
break;
}
else
{
var parameter = double.Parse(entry);
Console.Write(Math.Acos(parameter)*(180/Math.PI) + "\n");
}
}
else if (entry.ToLower() == "tangent")
{
Console.Write("Enter a tangent or type \"quit\" to leave: ");
entry = Console.ReadLine();
if (entry.ToLower() == "quit")
{
break;
}
else
{
var parameter = double.Parse(entry);
Console.Write(Math.Atan(parameter)*(180/Math.PI) + "\n");
}
}
else if (entry.ToLower() == "square")
{
Console.Write("Enter a number or type \"quit\" to leave: ");
entry = Console.ReadLine();
if (entry.ToLower() == "quit")
{
break;
}
else
{
var parameter = double.Parse(entry);
Console.Write(Math.Sqrt(parameter) + "\n");
}
}
else if (entry.ToLower() == "logarithm")
{
Console.Write("Enter the number or type \"quit\" to leave: ");
entry = Console.ReadLine();
Console.Write("Enter the base or type \"quit\" to leave: ");
var input = Console.ReadLine();
if (entry.ToLower() == "quit" || input.ToLower() == "quit")
{
break;
}
else
{
var parameter = double.Parse(entry);
var basic = int.Parse(input);
Console.Write(Math.Log(parameter, basic) + "\n");
}
}
//Exit if the user types "quit"
else if (entry.ToLower() == "quit")
{
break;
}
else
{
Console.Write("This is not valid input");
continue;
}
//Prompt the user for the parameters
//Print
//Repeat
}
catch (FormatException)
{
Console.Write("That is not valid input! \n");
continue;
}
}
}
}
1 Answer
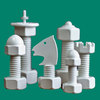
Steven Parker
241,970 PointsHere's a few ideas:
For one thing, you could "use the values you assigned to variables outside their respective loops" if you declare those variables first in the wider scope.
But it seems you can compact this program significantly if you create a dictionary of the operation terms as keys and function delegates as the values, and then invoke the delegate to perform the operation inside the main loop. I found a blog post that gives some examples.
Samuel Savaria
4,809 PointsSamuel Savaria
4,809 PointsDid not know that you could use the values outside the loop. I thought the value was destroyed at the end of the loop, thank you very much.
As for the dictionary, is it something we learn in a later course? If so I'd rather wait until then to have a better explaination.
Steven Parker
241,970 PointsSteven Parker
241,970 PointsYou are correct that the variable is disposed when you exit the scope it was declared/defined in. That's why I suggested declaring it in the wider scope and just assigning it within the loop.
And I do believe both dictionaries and delegates are introduced in later course(s) but I'm not sure which one(s).
Samuel Savaria
4,809 PointsSamuel Savaria
4,809 PointsOh, so the variable is disposed of at the end of the scope it was DEFINED in. I thought the value assigned to the variable was destroyed at the end of the scop it was assigned in. Again, thanks a lot for that information, will make my code a lot easier.
I'll look it up if it's not in any of the courses, thanks.