Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial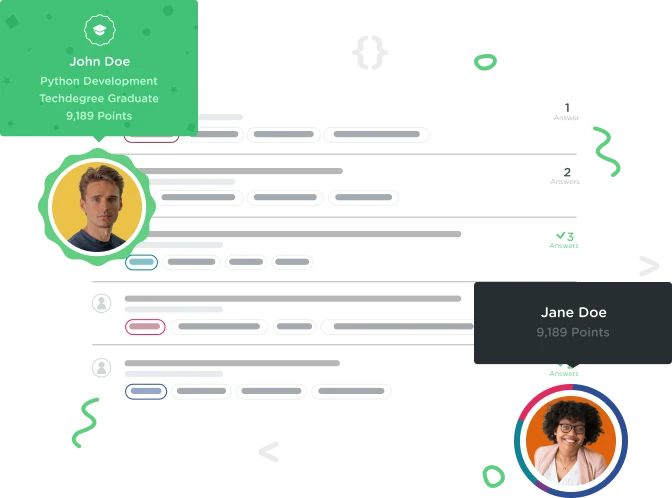

Samuel Savaria
4,809 PointsFeedback on my programs (Quiz).
I am doing the 4 side projects that were proposed at the end of C# Basics. I am looking for any type of feedback from the community: How to follow the conventions, improve readability, optimize the program, etc..
This is the second suggestion, the Quiz. The program has to ask the user 3 multiple-choice questions or true/false questions. It also needs to print the score at the end. My main issue with this program is it's length and repetitiveness. I know we will learn how to call other methods and classes later, as well as how to create objects, but I would like to know if there is a way to re-use the same bit of code with my current knowledge. Thanks.
using System;
class Program
{
static void Main()
{
var good = 0;
Console.Write("Enter \"a\" \"b\" \"c\" or \"d\" for multiple choice questions and \"true\" or \"false\" for true/false questions.\nPress any key to continue.\n");
Console.ReadLine();
//Question 1
Console.Write("How is the front of a ship called?: \na)Forefront\nb)Bow\nc)Stern\nd)Keel\n");
while (true)
{
//Receive input
var entry = Console.ReadLine();
//Give feedback
if (entry.ToLower() == "b")
{
Console.Write("Well done! ");
good += 1;
break;
}
else if (entry.ToLower() == "a" || entry.ToLower() == "c" || entry.ToLower() == "d")
{
Console.Write("Wrong. The front of a ship is called the bow. ");
break;
}
else
{
Console.Write("Please enter a valid input. \n");
}
}
Console.Write("Press any key to continue.\n");
Console.ReadLine();
//Question 2
Console.Write("How is the back of a ship called?: \na)Forefront\nb)Rudder\nc)Stern\nd)Keel\n");
while (true)
{
//Receive input
var entry = Console.ReadLine();
//Give feedback
if (entry.ToLower() == "c")
{
Console.Write("Well done! ");
good += 1;
break;
}
else if (entry.ToLower() == "a" || entry.ToLower() == "b" || entry.ToLower() == "d")
{
Console.Write("Wrong. The back of a ship is called the stern. ");
break;
}
else
{
Console.Write("Please enter a valid input. \n");
}
}
Console.Write("Press any key to continue.\n");
Console.ReadLine();
//Question 3
Console.Write("True or False? A Snow has 3 masts. \n");
while (true)
{
//Receive input
var entry = Console.ReadLine();
//Give feedback
if (entry.ToLower() == "false")
{
Console.Write("Well done! ");
good += 1;
break;
}
else if (entry.ToLower() == "true")
{
Console.Write("Wrong. A Snow has 2 masts. ");
break;
}
else
{
Console.Write("Please enter a valid input. \n");
}
}
Console.Write("Press any key to continue.\n");
Console.ReadLine();
//Print the score
Console.Write("You score is: " + good + "/3!\n");
}
}
1 Answer
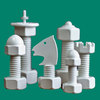
Steven Parker
231,122 PointsSure, you can easily compact this code.
One common method is to identify places in the code where the same thing is done over and over (such as checking answers and giving a response) and creating a function to do those things which you can call from multiple places.
You'll see this and other methods for optimizing your code explained and illustrated in later lessons.