Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial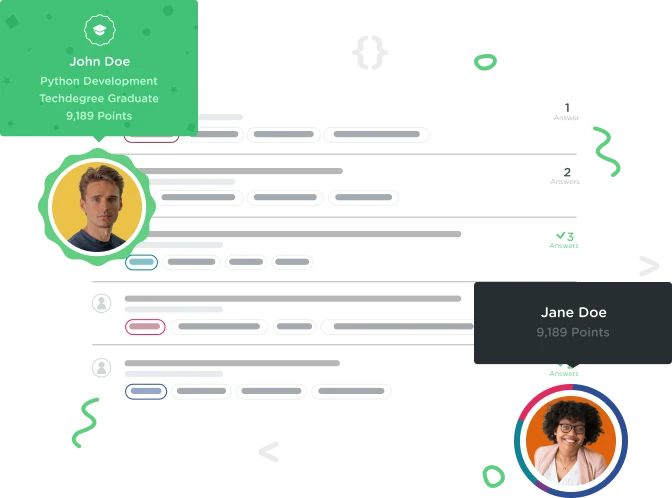
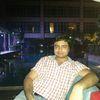
KnowledgeWoods Consulting
5,607 PointsFind sets of values in array whose Sum is equal to some number
I have an array containing the marks of 10 students. I want to calculate the number of sets in which marks of $students equals to maximum marks
1 Answer
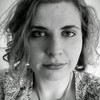
Grace Kelly
33,990 PointsHi Chetan, This solution uses a very simple associative array to store the marks of the students:
<?php
//set the maximum mark
$maximumMark = 500;
//student marks array
$studentMarks = array(
"Adam" => array(80, 90, 50, 20, 100),
"Mary" => array(95, 50, 45, 100, 90),
"Susan" => array(100,100,100,100,100),
"Joe" => array(100,100,100,100,100)
);
//set the counter
$count = 0;
//loop through the student marks array
foreach($studentMarks as $studentMark => $mark) {
//if the total sum of the student's marks matches the maximum mark, add 1 to the counter
if(array_sum($mark) === $maximumMark) {
$count++;
}
}
//echo out the results
echo "There are " . $count . " students with the maxiumum marks of " . $maximumMark;
?>
As you can see by setting a counter before the loop and adding to it while the loop runs, you can track the number of students that achieved the highest marks, hope this helps!!