Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial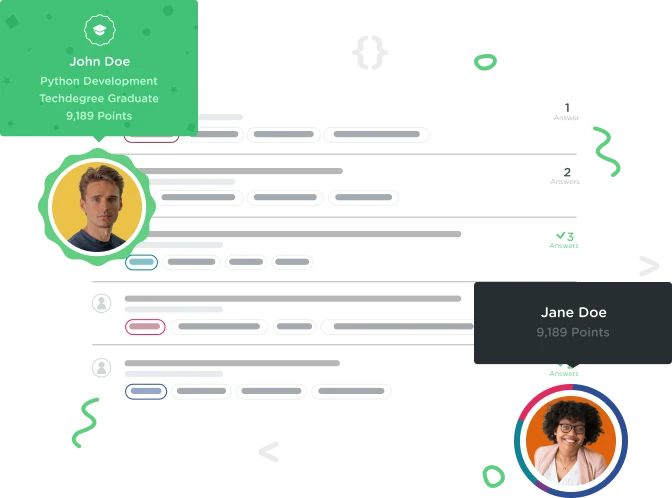
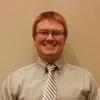
Patrick Shushereba
10,911 PointsFinding Prime Factors in Ruby
I'm trying to write a program that will find all of the prime factors of a given number. When I run the code that I have, it gives me the first couple of prime numbers, but only when the "n" value is small.
#The prime factors of 13195 are 5, 7, 13 and 29.
#What is the largest prime factor of the number 600851475143?
def prime_factors(n)
prime_array = []
p = 2
if n < 2
return p
end
while (n % p == 0) && (p < n)
prime_array.push(p)
p += 1
end
#puts prime_array.inspect
return prime_array
end
#prime_factors(600851475143)
puts prime_factors(30)
3 Answers

Daniel Cunningham
21,109 PointsMy read on your code: <br><br>
- Method "Prime Factors takes n value as an argument" <br>
- Creates a blank array called prime_array and a variable p = 2 <br>
- If n is less than 2, immediately return 2 (value of p) <br> This next part confuses me...
- While value n modulo p is equal to zero AND p is less than n, you will push P into the prime array. <br>
The moment n modulo p is not equal to zero, the method stops when it should continue until it finds all prime numbers generating a zero. Try this:
While p < n
if n % p == 0
prime_array.push(p)
end
p +=1
end
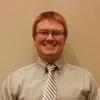
Patrick Shushereba
10,911 PointsGood call. All it's doing it outputting 2 continuously. But I'm not sure how else to increment the loop.

Daniel Cunningham
21,109 PointsI put your code into a file and ran it, and received 2,3,5,6,10, and 15 for results for your function.
the code I used was
def prime_factors(n)
prime_array = []
p = 2
if n < 2
return p
end
while p < n
if n % p == 0
prime_array.push(p)
end
p +=1
end
#puts prime_array.inspect
return prime_array
end
#prime_factors(600851475143)
puts prime_factors(30)
Perhaps an "end" was in the wrong place for your code, but the issue was that the incrementer was being ignored.
Good luck, hope that helps!
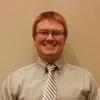
Patrick Shushereba
10,911 PointsThank you! I got the program running. I'm finding that the results give me all of the factors, not just the prime ones. So I have to go through and figure out how to fix that. I know there's a prime numbers class, but I'm trying to avoid using it. Thank you for all of the help.

Daniel Cunningham
21,109 PointsYou're very welcome! As a thought, you can probably add a second test to find out if any of the factors are divisible by any of the earlier factors. If the answer is yes, then it shouldnt be pushed into the array finder. If not, then it is added to the array finder and used in the testing process of the next p value.
good luck!
Patrick Shushereba
10,911 PointsPatrick Shushereba
10,911 PointsThanks a lot. When I run the program in the terminal I don't get a response. I don't know if I have a memory issue, but even when I run the test with a small "n" value (I've been using 30 as a test), the program continues to run but doesn't give me a response. What can I do to fix that?
Daniel Cunningham
21,109 PointsDaniel Cunningham
21,109 PointsAs a diagnostic test, add "puts p" to the bottom of the while loop. If it keeps outputting 2 or isnt incrementing correctly, then we know it's a problem with incrementing in the loop. It shouldnt take long for it to get to 30.