Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial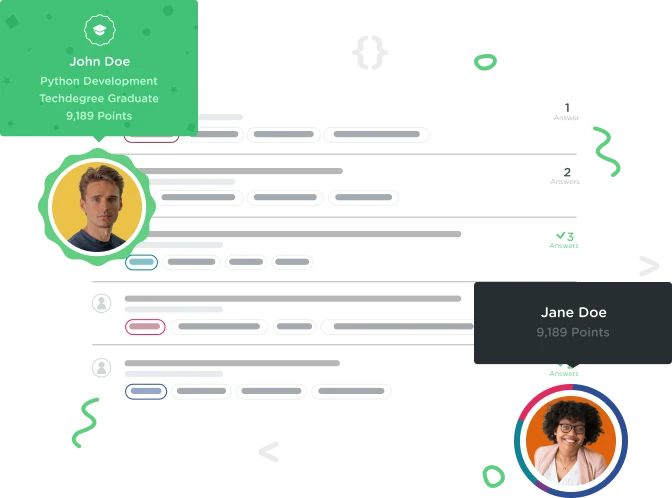

douglas gray
888 Pointsfinishing this challenge
Hello, all. For the following challenge you are given directions to 1) ask a user for a number of times to print "yay" and 2) print "yay" to the screen that many times using a loop. I assumed that I should create my own variable and assign it the user's response using the ReadLine() method and then create a while loop, but I'm being told that an unexpected symbol - "while"- appears and my code cant be compiled. Could anyone tell me if there is a better way to accomplish the goal, or why my while loop isn't recognized? My code is below. Thank you.
using System;
namespace Treehouse.CodeChallenges { class Program { static void Main() { Console.Write("Enter the number of times to print \"Yay!\": ");
var yayTimes = Console.ReadLine()
while (yayTimes >= 1)
{
Console.WriteLine("yay")
yayTimes = yayTimes - 1
}
}
}
}
using System;
namespace Treehouse.CodeChallenges
{
class Program
{
static void Main()
{
Console.Write("Enter the number of times to print \"Yay!\": ");
var yayTimes = Console.ReadLine()
while (yayTimes >= 1)
{
Console.WriteLine("yay")
yayTimes = yayTimes - 1
}
}
}
}
2 Answers

Joshua Edwards
52,175 PointsRemember to end your code statements with a semicolon. Also, remember that you need to convert what you are getting from the console (a string) into an integer if you want to perform mathematical operations on it.

andren
28,558 PointsThere are a two issues with your code:
Code statements must end with a semicolon, you seem to have forgotten to include it on pretty much all of the code you wrote, that is why the compiler is confused by "while" suddenly appearing, it is still waiting for the previous code statement to be ended by a semicolon.
Console.ReadLine() returns a string not an int, strings can not be compared to int's with <,>,= etc. The solution to this problem is to convert the string to an int, this can be done in a few ways but the simplest is probably by using the int.Parse method, int.Parse take a string a returns it as an int.
I'd suggest you try solving the issue on your own using the hints I gave you, but if you still don't understand how it's done then I have posted the solution code here for you to look at.

douglas gray
888 PointsThank you. I was a bit hasty asking the question. I made those adjustments and it runs fine.
douglas gray
888 Pointsdouglas gray
888 PointsThank you. I made a couple of adjustments and it runs fine. I was also wondering, is there better way to read a users input in one step, like with the input() function in python?