Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial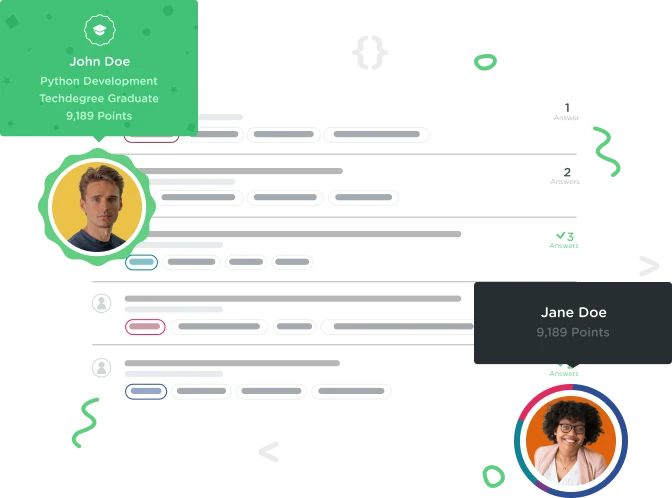
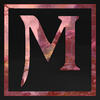
Matthew Lang
13,483 PointsForm Validation doesn't work PHP
Hey. Trying to do some form validation on my own, but I have stumbled into a problem. I am using the POST method, and the action is just PHP_SELF. As I display the form, below each field I am using PHP to check an 'error' array, and if it has items, display the strings. Once the user submits the form, it then goes through a validation check. Currently I only have 1 validation check, and that is for the username to not contain any whitespace. If it does contain whitespace, then it adds a string onto the username_err array, which should then be displayed back to the user, right? well, it doesn't and I'm stuck. any help? Here's my code
<div class="sign-up-form">
<h1>Register an account</h1>
<p>Already have an account? <a id="login-refer" href='login.php'>Log in.</a></p>
<form method="POST" action="<?php echo htmlspecialchars($_SERVER["PHP_SELF"]);?>">
<?php
// Initialize variables for error reporting
$username_err = [];
$password_err = [];
?>
<!-- Username -->
<label for='label_username'>Username</label><br>
<input type='text' id='label_username' name="input-username"></input><br>
<?php
if(count($username_err) > 0) {
foreach($username_err as $error) {
echo '<p class="error">' . $error . '</p>';
}
}
?>
<!-- Email -->
<label for='label_email'>Email</label><br>
<input type='email' id='label_email' name="input-email"></input><br>
<!-- Password -->
<label for='label_password'>Password</label><br>
<input type='password' id='label_password' name="input-pass"></input><br>
<!-- Re-Type Password -->
<label for='label_repassword'>Re-type Password</label><br>
<input type='password' id='label_repassword' name="input-repass"></input><br>
<?php
if(count($password_err) > 0) {
foreach($password_err as $error) {
echo '<p class="error">' . $error . '</p>';
}
}
?>
<!-- Submit -->
<input type='submit' value='Register'></input>
<?php
// Validation of form checking
if(isset($_POST['input-username'])) {
$username = $_POST['input-username'];
$email = $_POST['input-email'];
$password = $_POST['input-pass'];
$repassword = $_POST['input-repass'];
}
if(trim($username) !== $username) {
$username_err[] = "Username must not contain any spaces.";
}
?>
</form>
</div>
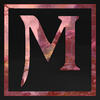
Matthew Lang
13,483 PointsThank you so much! Now that you've pointed it out to me it makes so much sense now and I feel really dumb. thank you :)
Ben Payne
1,464 PointsBen Payne
1,464 PointsHey Mathew,
You're overwriting the error arrays right after the opening
form
tag. So whatever was in there from the validation is gone. Also, inputs are self closing, they don't need a</input>
closing tag.I reworked the script for you here: