Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial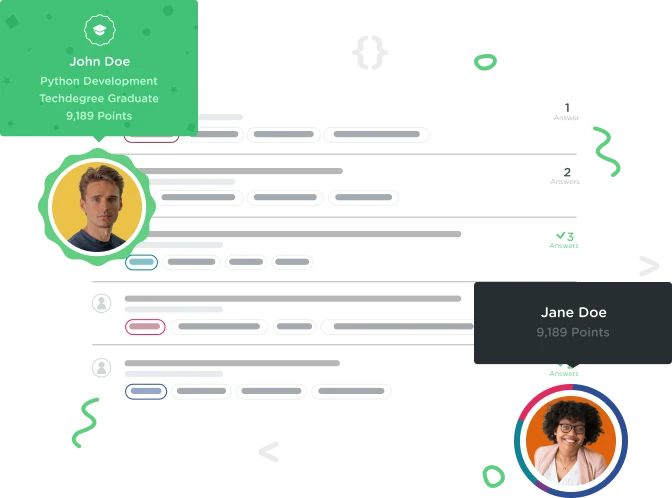

kdkimmer
1,109 PointsForumPost will not except my args or at least the first one.
I am passing in author. That should work as I have already put my args in User author.
public class ForumPost {
private User mAuthor;
private String mTitle;
private String mDescription;
public ForumPost(User author, String title, String description) {
mAuthor = author;
mTitle = title;
mDescription = description;
}
public String getDescription() {
return mDescription;
}
public User getAuthor() {
return mAuthor;
}
public String getTitle() {
return mTitle;
}
// TODO: We need to expose the description
}
public class User {
private String mFirstName;
private String mLastName;
public String getFirstName() {
return mFirstName;
}
public String getLastName() {
return mLastName;
}
public User(String firstName, String lastName) {
// TODO: Set the private fields here
mFirstName = firstName;
mLastName = lastName;
}
}
public class Forum {
private String mTopic;
public String getTopic() {
return mTopic;
}
public Forum(String topic) {
mTopic = topic;
}
public void addPost(ForumPost post) {
// When all is ready uncomment this...
System.out.printf("New post from %s %s about %s.\n",
post.getAuthor().getFirstName(),
post.getAuthor().getLastName(),
post.getTitle());
}
}
public class Example {
public static void main(String[] args) {
System.out.println("Starting forum example...");
if (args.length < 2) {
System.out.println("first and last name are required. eg: java Example Craig Dennis");
}
Forum forum = new Forum("Java");
// Take the first two elements passed args
User author = new User("Kd", "Kimmer");
// Add the author, title and description
ForumPost post = new ForumPost(author, "It is Finished", "Thrilled");
forum.addPost(post);
}
}

kdkimmer
1,109 Points./Example.java:12: error: cannot find symbol ForumPost post = new ForumPost(author, "It is Finished", "Thrilled"); ^ symbol: variable User location: class Example 1 error
4 Answers
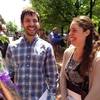
Mike D'Aguillo
5,475 PointsHey kdkimmer, would you mind posting any error messages that you're running into? Just post the exact text that's printed by your console, it should help us debug.
My only thought is perhaps you haven't imported the User class in your example class? Tough to tell without any error messages. The rest of your code looks fine at first glance.

kdkimmer
1,109 PointsMy error says: Bummer I expected to see the names I passed in through the args but I do not. Hmm.
./Example.java:12: error: cannot find symbol ForumPost post = new ForumPost(author, "It is Finished", "Thrilled"); ^ symbol: variable User location: class Example 1 error
I am not sure what I am doing wrong.
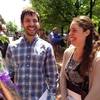
Mike D'Aguillo
5,475 PointsAhh I didn't realize that this is part of an exercise, I thought you were just having trouble with a program in general. In this instance, the exercise is expecting certain arguments to be passed to the User object when you first initialize it.
For one, learning to read an error message will help you tremendously when you're debugging programs on your own in the future. I want to point out a key phrase from the console printout:
"Example.java:12: error: cannot find symbol" - this is telling you to look at your Example class, and at the 12th line of code. You'll see exactly which line the program is running into trouble. Second the part that says "symbol: variable User" is indicating the the specific issue lies with the User object, so you know you have to fix that.
When you're first learning Java, the syntax of writing your main class can seem really confusing. In all cases with Java, your main class will always take a parameter "String[] args", much how like many of your other objects or functions you create might take a parameter of their own. As far as I understand, this string array comes from the parameters you include when you run a program from the command line (ex: java Example.class ARG0 ARG1 ARG2), though I could be wrong.
Now reread the instructions of the exercise with this new understanding. It sounds like you need to be using the args passed into the example class. How would you do that?

Craig Dennis
Treehouse TeacherThis hint should help:
// Take the first two elements passed args
Let me know if it doesn't ;)

Craig Dennis
Treehouse TeacherWhen I run the test I pass in two arguments to the main method. They are first name, last name and are in the args array. You are expected to use them.
Hope that helps!

kdkimmer
1,109 PointsThanks to both of you. I cannot believe I did that....and I got so stuck. instead of referencing the args I added my own personal info even though on the user class I knew I had my getters that would post into my args. Do not know why I did that and got that stuck. Thanks.
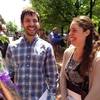
Mike D'Aguillo
5,475 PointsNo problem man, happy to help :) No matter the scale of the problem, solving an error is one of the more gratifying feelings when programming. Everyone makes dumb little mistakes no matter the experience level, so don't put yourself down!

Craig Dennis
Treehouse TeacherI have a permanent bruise on my forehead from face palming all the time. Glad you made it through! Congrats, that one was intense!

Michael McKenna
3,451 Pointskdkimmer, what do you end up putting in the last part? I'm not understanding what Craig meant in his tip.
kdkimmer
1,109 Pointskdkimmer
1,109 PointsMy error says: Bummer I expected to see the names I passed in through the args but I do not. Hmm.